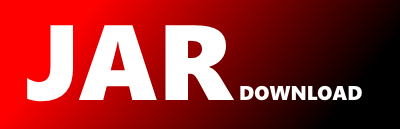
com.mntviews.jreport.JRFile Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mnt-report Show documentation
Show all versions of mnt-report Show documentation
JasperReport server engine
package com.mntviews.jreport;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonPOJOBuilder;
import com.mntviews.jreport.exception.JRFileException;
import java.io.ByteArrayOutputStream;
import java.util.Base64;
import static com.mntviews.jreport.JRContext.checkIfNullThenDefault;
@JsonDeserialize(builder = JRFile.Builder.class)
@JsonIgnoreProperties(ignoreUnknown = true)
public class JRFile {
private final ByteArrayOutputStream data;
private final String name;
private final JRExportType exportType;
private JRFile(Builder builder) {
JRFile jrFile = JRContext.findProfile().getJrFile();
this.name = checkIfNullThenDefault(builder.name, jrFile == null ? null : jrFile.name);
this.exportType = checkIfNullThenDefault(builder.exportType, jrFile == null ? null : jrFile.exportType);
this.data = builder.data;
if (jrFile != null) {
if (this.data == null)
throw new JRFileException("'data' is not defined");
if (this.name == null)
throw new JRFileException("'name' is not defined");
if (this.exportType == null)
throw new JRFileException("'exportType' is not defined");
}
}
public static Builder custom() {
return new Builder();
}
@JsonPOJOBuilder
public static class Builder {
private ByteArrayOutputStream data;
private String name;
private JRExportType exportType;
@JsonIgnore
Builder withData(ByteArrayOutputStream data) {
this.data = data;
return this;
}
@JsonProperty("data")
Builder withDataBase64(String data) {
if (data == null)
throw new JRFileException("data is null");
byte[] dataArray = Base64.getDecoder().decode(data);
this.data = new ByteArrayOutputStream(dataArray.length);
this.data.write(dataArray, 0, dataArray.length);
return this;
}
@JsonProperty("name")
Builder withName(String name) {
this.name = name;
return this;
}
@JsonIgnore
Builder withExportType(JRExportType exportType) {
this.exportType = exportType;
return this;
}
@JsonProperty("exportType")
Builder withExportTypeTag(String exportTypeTag) {
try {
this.exportType = JRExportType.valueOf(exportTypeTag);
} catch (IllegalArgumentException e) {
throw new JRFileException("exportType '" + exportTypeTag + "' is not defined");
}
return this;
}
public JRFile build() {
return new JRFile(this);
}
}
@JsonIgnore
public ByteArrayOutputStream getData() {
return data;
}
@JsonProperty("data")
public String getDataAsBase64() {
if (data != null)
return Base64.getEncoder().encodeToString(data.toByteArray());
else
return null;
}
@JsonProperty("name")
public String getName() {
return name;
}
@JsonIgnore
public JRExportType getExportType() {
return exportType;
}
@JsonProperty("exportType")
public String getExportTypeTag() {
return exportType == null ? null : exportType.toString();
}
@JsonProperty("mimeType")
public String getMimeType() {
return exportType == null ? null : exportType.getMimeType();
}
@JsonProperty("fileName")
public String getFileName() {
return name + "." + (exportType == null ? null : exportType.getExtension());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy