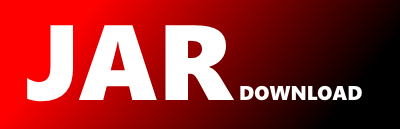
com.mntviews.jreport.JROutputTelegram Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mnt-report Show documentation
Show all versions of mnt-report Show documentation
JasperReport server engine
package com.mntviews.jreport;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonPOJOBuilder;
import com.mntviews.jreport.exception.JROutputException;
import lombok.extern.slf4j.Slf4j;
import org.jfree.util.Log;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.List;
import static com.mntviews.jreport.JRContext.checkIfNullThenDefault;
@Slf4j
@JsonDeserialize(builder = JROutputTelegram.Builder.class)
public class JROutputTelegram extends JROutput {
@JsonProperty("botId")
private final String botId;
@JsonProperty("chatId")
private final String chatId;
@JsonProperty("message")
private final String message;
@JsonProperty("url")
private final String url;
public static Builder custom() {
return new Builder();
}
private JROutputTelegram(Builder builder) {
JROutputTelegram jrOutputTelegram = JRContext.findProfile().getJrOutputTelegram();
this.botId = checkIfNullThenDefault(builder.botId, jrOutputTelegram == null ? null : jrOutputTelegram.botId);
this.chatId = checkIfNullThenDefault(builder.chatId, jrOutputTelegram == null ? null : jrOutputTelegram.chatId);
this.message = checkIfNullThenDefault(builder.message, jrOutputTelegram == null ? null : jrOutputTelegram.message);
this.url = checkIfNullThenDefault(builder.url, jrOutputTelegram == null ? null : jrOutputTelegram.url);
if (jrOutputTelegram != null) {
if (this.botId == null)
throw new JROutputException("'botId' is not defined");
if (this.chatId == null)
throw new JROutputException("'chatId' is not defined");
if (this.url == null)
throw new JROutputException("'url' is not defined");
}
}
@Override
public void execute(List fileList) {
URL urlTelegram;
try {
String fullUrl = String.format(url, botId, chatId, message);
Log.debug("GET " + fullUrl);
urlTelegram = new URL(fullUrl);
HttpURLConnection con = (HttpURLConnection) urlTelegram.openConnection();
con.setRequestMethod("GET");
BufferedReader in = new BufferedReader(new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuilder sb = new StringBuilder();
sb.append("response: ");
while ((inputLine = in.readLine()) != null) {
sb.append(inputLine);
}
Log.debug(sb.toString());
in.close();
} catch (Exception e) {
throw new JROutputException(e);
}
}
@JsonPOJOBuilder
public static class Builder {
private String botId;
private String chatId;
private String message;
private String url;
@JsonProperty("botId")
public Builder withBotId(String botId) {
this.botId = botId;
return this;
}
@JsonProperty("chatId")
public Builder withChatId(String chatId) {
this.chatId = chatId;
return this;
}
@JsonProperty("message")
public Builder withMessage(String message) {
this.message = message;
return this;
}
@JsonProperty("url")
public Builder withUrl(String url) {
this.url = url;
return this;
}
public JROutputTelegram build() {
return new JROutputTelegram(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy