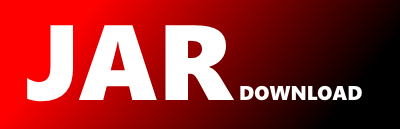
com.mntviews.jreport.JRPackage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mnt-report Show documentation
Show all versions of mnt-report Show documentation
JasperReport server engine
package com.mntviews.jreport;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonPOJOBuilder;
import com.mntviews.jreport.exception.JRPackageException;
import lombok.extern.slf4j.Slf4j;
import java.io.*;
import java.nio.charset.StandardCharsets;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
import static com.mntviews.jreport.JRContext.checkIfNullThenDefault;
@JsonDeserialize(builder = JRPackage.Builder.class)
@JsonInclude(JsonInclude.Include.NON_NULL)
@Slf4j
public class JRPackage {
@JsonProperty("outputs")
private final List outputList;
@JsonProperty("reports")
private final List reportList;
@JsonProperty("connection")
private final JRConnection connection;
@JsonProperty("isZip")
private final Boolean isZip;
@JsonProperty("params")
private final List paramList;
@JsonProperty("profile")
private final String profile;
private static final ObjectMapper objectMapper = new ObjectMapper();
public List getOutputList() {
return outputList;
}
private JRPackage(Builder builder) {
JRProfile jrProfile = JRContext.findProfile();
JRPackage jrPackage = jrProfile.getJrPackage();
if (jrPackage != null) {
this.outputList = builder.outputList;
this.reportList = builder.reportList;
this.isZip = checkIfNullThenDefault(builder.isZip, jrPackage.isZip);
this.profile = checkIfNullThenDefault(builder.profile, jrPackage.profile);
this.connection = checkIfNullThenDefault(builder.connection, jrProfile.getJrConnection());
this.paramList = JRParamList.combineParamList(jrProfile.getJrParamList(), builder.paramList);
if (this.profile == null)
throw new JRPackageException("'profile' is not defined");
if (this.connection == null)
throw new JRPackageException("'connection' is not defined");
if (this.paramList == null)
throw new JRPackageException("'paramList' is not defined");
} else { /* Profile initialization */
this.connection = null;
this.paramList = null;
this.outputList = null;
this.reportList = null;
this.isZip = builder.isZip;
this.profile = builder.profile;
}
}
public static Builder custom() {
return new JRPackage.Builder();
}
public static JRPackage deserializeJRPackage(InputStream scriptStream) {
try (BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(scriptStream, StandardCharsets.UTF_8))) {
String script = bufferedReader.lines().collect(Collectors.joining(System.lineSeparator()));
JRPackageProfileStub jrPackageProfileStub = objectMapper.readValue(script, JRPackageProfileStub.class);
if (jrPackageProfileStub != null && jrPackageProfileStub.getProfileTag() != null)
JRContext.setProfileTag(jrPackageProfileStub.getProfileTag().toUpperCase());
else
JRContext.setProfileTag(JRContext.DEFAULT_PROFILE_TAG);
log.debug("setProfileTag=" + JRContext.getProfileTag());
return objectMapper.readValue(script, JRPackage.class);
} catch (IOException e) {
throw new JRPackageException(e);
} finally {
// JRContext.setProfileTag(JRContext.DEFAULT_PROFILE_TAG);
}
}
public static JRPackage deserializeJRPackage(String script) {
return deserializeJRPackage(new ByteArrayInputStream(script.getBytes()));
}
public static String serializeJRPackage(JRPackage jRPackage) {
try {
return objectMapper.writerWithDefaultPrettyPrinter().writeValueAsString(jRPackage);
} catch (JsonProcessingException e) {
throw new JRPackageException(e);
}
}
@JsonPOJOBuilder
public static class Builder {
private List outputList;
private List reportList;
private List paramList;
private JRConnection connection;
private Boolean isZip;
private String profile;
Builder() {
this.reportList = new ArrayList<>();
this.outputList = new ArrayList<>();
this.paramList = new ArrayList<>();
}
@JsonProperty("outputs")
public Builder withOutputList(List outputList) {
this.outputList = outputList;
return this;
}
@JsonProperty("reports")
public Builder withReportList(List reportList) {
this.reportList = reportList;
return this;
}
@JsonProperty("params")
public JRPackage.Builder withParamList(List paramList) {
this.paramList = JRParamList.clone(paramList);
return this;
}
@JsonProperty("profile")
public JRPackage.Builder withProfile(String profile) {
this.profile = profile;
return this;
}
@JsonProperty("connection")
public Builder withConnection(JRConnection connection) {
this.connection = connection;
return this;
}
@JsonProperty("isZip")
public Builder withIsZip(Boolean isZip) {
this.isZip = isZip;
return this;
}
public JRPackage build() {
return new JRPackage(this);
}
}
public List execute(JRParam... paramArray) {
return execute(Arrays.asList(paramArray));
}
public List execute(List paramList) {
List newParamList;
if (paramList == null || paramList.isEmpty())
newParamList = this.paramList;
else
newParamList = JRParamList.combineParamList(this.paramList, paramList);
log.debug("execute");
log.debug("profileTag=" + JRContext.getProfileTag());
log.debug("params=");
newParamList.forEach(p -> log.debug(p.getKey() + "=" + p.getVal()));
final List jrFileList = reportList != null ? reportList.stream().map(r -> r.execute(connection, newParamList)).collect(Collectors.toList()) : new ArrayList<>();
if (outputList != null)
outputList.forEach(r -> r.execute(jrFileList));
return jrFileList;
}
public List execute() {
return execute(new ArrayList<>());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy