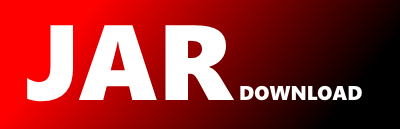
com.mntviews.jreport.JRReport Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mnt-report Show documentation
Show all versions of mnt-report Show documentation
JasperReport server engine
package com.mntviews.jreport;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonPOJOBuilder;
import com.mntviews.jreport.exception.JRReportException;
import lombok.extern.slf4j.Slf4j;
import net.sf.jasperreports.engine.*;
import net.sf.jasperreports.engine.fill.JRGzipVirtualizer;
import java.sql.Connection;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import static com.mntviews.jreport.JRContext.checkIfNullThenDefault;
@JsonDeserialize(builder = JRReport.Builder.class)
@JsonInclude(JsonInclude.Include.NON_NULL)
@Slf4j
public class JRReport {
@JsonProperty("template")
private final JRTemplate template;
@JsonProperty("input")
private final JRInput input;
@JsonIgnore
private final JRExportType exportType;
@JsonProperty("params")
private final List paramList;
@JsonProperty("path")
private final String path;
@JsonProperty("fileName")
private final String fileName;
@JsonProperty("isZip")
private final Boolean isZip;
@JsonProperty("configScript")
private final String configScript;
@JsonIgnore
private final JRBeforeReportAction beforeReportAction;
public JRReport(Builder builder) {
JRReport jrReport = JRContext.findProfile().getJrReport();
this.isZip = checkIfNullThenDefault(builder.isZip, jrReport == null ? null : jrReport.isZip);
this.configScript = checkIfNullThenDefault(builder.configScript, jrReport == null ? null : jrReport.configScript);
this.fileName = checkIfNullThenDefault(builder.fileName, jrReport == null ? null : jrReport.fileName);
this.path = checkIfNullThenDefault(builder.path, jrReport == null ? null : jrReport.path);
this.beforeReportAction = checkIfNullThenDefault(builder.jrBeforeReportAction, JRContext.findProfile().getJrBeforeReportAction());
List jrParamList = JRContext.findProfile().getJrParamList();
if (jrParamList != null)
this.paramList = JRParamList.combineParamList(jrParamList, builder.paramList);
else
this.paramList = JRParamList.clone(builder.paramList);
this.exportType = checkIfNullThenDefault(builder.exportType, jrReport == null ? null : jrReport.exportType);
this.template = builder.template;
if (builder.input == null)
this.input = JRInput.custom().withInputSource(JRInputSourceDB.clone(JRContext.findProfile().getJrInputSourceDB())).build();
else
this.input = builder.input;
if (jrReport != null) {
if (this.exportType == null)
throw new JRReportException("'exportType' is not defined");
if (this.input == null)
throw new JRReportException("'input' is not defined");
if (this.template == null)
throw new JRReportException("'template' is not defined");
}
}
public JRTemplate getTemplate() {
return template;
}
@JsonIgnore
public JRExportType getExportType() {
return exportType;
}
@JsonProperty(value = "exportType")
public String getExportTypeTag() {
return exportType == null ? null : exportType.toString();
}
public String getFileName() {
return fileName;
}
public static Builder custom() {
return new Builder();
}
/**
* Executes report and makes output JRFile . Also executes arbitrary action before/
*
* @param jRConnection connection to the database. If null then predefined connection
* @param paramList
* @return generated JRFile
*/
public JRFile execute(JRConnection jRConnection, List paramList) {
HashMap properties = new HashMap<>();
List newParamList;
if (paramList == null || paramList.isEmpty())
newParamList = this.paramList;
else
newParamList = JRParamList.combineParamList(paramList, this.paramList);
newParamList.forEach(p -> properties.put(p.getKey(), p.getVal()));
JRGzipVirtualizer virtualizer = new JRGzipVirtualizer(4);
try {
Connection connection = null;
JRInputSource inputSource = input.getJrInputSource();
JRTemplateCommon jrTemplateCommon = JRTemplateCommon.custom().withConnection(jRConnection).withPath(path).build();
if (inputSource instanceof JRInputSourceDB) {
if (((JRInputSourceDB) (input.getJrInputSource())).getJRConnection() == null)
if (jrTemplateCommon.getJrConnection() != null)
connection = jrTemplateCommon.getJrConnection().getConnection();
else {
JRInputSourceDB jrInputSourceDB = (JRInputSourceDB) input.getJrInputSource();
if (jrInputSourceDB.getJRConnection() != null)
connection = jrInputSourceDB.getJRConnection().getConnection();
}
if (connection == null)
log.warn("DB connection not found");
}
if (beforeReportAction != null) {
beforeReportAction.execute(connection);
}
properties.put(JRParameter.REPORT_VIRTUALIZER, virtualizer);
JasperPrint jasperPrint;
if (inputSource instanceof JRInputSourceDB && connection != null) {
jasperPrint = JasperFillManager.fillReport(template.compile(jrTemplateCommon), properties, connection);
} else {
JRDataSource jrDataSource = inputSource.find();
if (jrDataSource == null)
jasperPrint = JasperFillManager.fillReport(template.compile(jrTemplateCommon), properties);
else
jasperPrint = JasperFillManager.fillReport(template.compile(jrTemplateCommon), properties, jrDataSource);
}
return JRFile.custom().withData(exportType.export(jasperPrint, configScript)).withName(fileName).withExportType(exportType).build();
} catch (JRException | SQLException e) {
throw new JRReportException(e);
} finally {
virtualizer.cleanup();
}
}
@JsonPOJOBuilder
public static class Builder {
private JRTemplate template;
private JRExportType exportType;
private String fileName;
private List paramList;
private JRBeforeReportAction jrBeforeReportAction;
private Boolean isZip;
private String configScript;
private JRInput input;
private String path;
@JsonCreator
public Builder() {
this.paramList = new ArrayList<>();
}
@JsonProperty("exportType")
public Builder withExportTypeTag(String exportTypeTag) {
try {
this.exportType = JRExportType.valueOf(exportTypeTag);
} catch (IllegalArgumentException e) {
throw new JRReportException("exportType '" + exportTypeTag + "' is not defined");
}
return this;
}
public Builder withExportType(JRExportType exportType) {
this.exportType = exportType;
return this;
}
public Builder withBeforeReportAction(JRBeforeReportAction jrBeforeReportAction) {
this.jrBeforeReportAction = jrBeforeReportAction;
return this;
}
@JsonProperty("template")
public Builder withTemplate(JRTemplate template) {
this.template = template;
return this;
}
@JsonProperty("fileName")
public Builder withFileName(String fileName) {
this.fileName = fileName;
return this;
}
@JsonProperty("path")
public Builder withPath(String path) {
this.path = path;
return this;
}
@JsonProperty("params")
public Builder withParamList(List paramList) {
this.paramList = JRParamList.clone(paramList);
return this;
}
@JsonProperty("isZip")
public Builder withIsZip(Boolean isZip) {
this.isZip = isZip;
return this;
}
@JsonProperty("input")
public Builder withInput(JRInput input) {
this.input = input;
return this;
}
@JsonProperty("configScript")
public Builder withConfigScript(String configScript) {
this.configScript = configScript;
return this;
}
public JRReport build() {
return new JRReport(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy