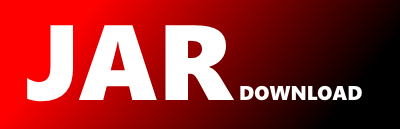
com.mntviews.jreport.JRTemplateSourceDB Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mnt-report Show documentation
Show all versions of mnt-report Show documentation
JasperReport server engine
package com.mntviews.jreport;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonPOJOBuilder;
import com.mntviews.jreport.exception.JRTemplateException;
import java.io.ByteArrayInputStream;
import java.io.InputStream;
import java.nio.charset.StandardCharsets;
import java.sql.Connection;
import static com.mntviews.jreport.JRContext.checkIfNullThenDefault;
@JsonDeserialize(builder = JRTemplateSourceDB.Builder.class)
@JsonInclude(JsonInclude.Include.NON_NULL)
public class JRTemplateSourceDB extends JRTemplateSource {
@JsonProperty("tag")
private final String tag;
@JsonProperty("connection")
private final JRConnection connection;
public String getTag() {
return tag;
}
@JsonIgnore
private JRTemplateDBExtractor templateDBExtractor;
@JsonIgnore
public JRConnection getJRConnection() {
return connection;
}
public JRTemplateDBExtractor getTemplateDBExtractor() {
return templateDBExtractor;
}
private JRTemplateSourceDB(Builder builder) {
JRProfile jrProfile = JRContext.findProfile();
JRTemplateSourceDB jrTemplateSourceDB = jrProfile.getJrTemplateSourceDB();
JRConnection jrConnection = jrProfile.getJrConnection();
JRTemplateDBExtractor templateDBExtractorTemp = JRContext.findTemplateDBExtractor();
if (builder.connection!=null
&& builder.connection.getTag()!=null
&& builder.connection.getTag().equalsIgnoreCase((JRContext.DEFAULT_CONNECTION_TAG))) {
this.connection = JRConnection.clone(jrConnection);
}
else
this.connection=builder.connection;
this.tag = checkIfNullThenDefault(builder.tag, jrTemplateSourceDB == null ? null : jrTemplateSourceDB.getTag());
this.templateDBExtractor = checkIfNullThenDefault(builder.templateDBExtractor, templateDBExtractorTemp);
if (jrTemplateSourceDB != null) {
if (this.tag == null)
throw new JRTemplateException("'tag' is not defined");
if (this.templateDBExtractor == null && this.connection!=null)
throw new JRTemplateException("'templateDBExtractor' is not defined");
}
}
public static Builder custom() {
return new Builder();
}
@Override
InputStream load(JRTemplateCommon jrTemplateCommon) {
String template;
Connection conn;
/* It's better to get common connection from Package first before default profile */
if (connection == null) {
if (jrTemplateCommon.getJrConnection() == null) {
throw new JRTemplateException("Connection not found");
} else
conn = jrTemplateCommon.getJrConnection().getConnection();
} else
conn = connection.getConnection();
if (conn == null)
throw new JRTemplateException("Connection is null");
template = templateDBExtractor.findTemplateByTag(conn, tag);
if (template == null) {
throw new JRTemplateException("Template with tag=" + tag + " not found");
}
return
new ByteArrayInputStream(template.getBytes((StandardCharsets.UTF_8)));
}
@JsonPOJOBuilder
public static class Builder {
private String tag;
private JRConnection connection;
private JRTemplateDBExtractor templateDBExtractor;
@JsonProperty("connection")
public Builder withConnection(JRConnection connection) {
this.connection = connection;
return this;
}
@JsonProperty("tag")
public Builder withTag(String tag) {
this.tag = tag;
return this;
}
@JsonIgnore
public Builder withTemplateDBExtractor(JRTemplateDBExtractor templateDBExtractor) {
this.templateDBExtractor = templateDBExtractor;
return this;
}
public JRTemplateSourceDB build() {
return new JRTemplateSourceDB(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy