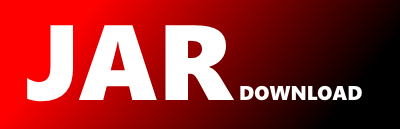
com.mntviews.jreport.JRTemplateSourceFile Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mnt-report Show documentation
Show all versions of mnt-report Show documentation
JasperReport server engine
package com.mntviews.jreport;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonPOJOBuilder;
import com.mntviews.jreport.exception.JRTemplateException;
import java.io.InputStream;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.sql.Connection;
import static com.mntviews.jreport.JRContext.checkIfNullThenDefault;
@JsonDeserialize(builder = JRTemplateSourceFile.Builder.class)
public class JRTemplateSourceFile extends JRTemplateSource {
@JsonProperty("name")
private final String name;
@JsonProperty("path")
private final String path;
public static Builder custom() {
return new Builder();
}
public String getName() {
return name;
}
public String getPath() {
return path;
}
private JRTemplateSourceFile(Builder builder) {
JRTemplateSourceFile jrTemplateSourceFile = JRContext.findProfile().getJrTemplateSourceFile();
this.name = checkIfNullThenDefault(builder.name, jrTemplateSourceFile == null ? null : jrTemplateSourceFile.getName());
this.path = builder.path;
if (jrTemplateSourceFile != null) {
if (this.name == null)
throw new JRTemplateException("'name' is not defined");
}
}
@Override
InputStream load(JRTemplateCommon jrTemplateCommon) {
try {
String pathLocal;
/* It's better to get common path from Package first before default profile */
if (this.path == null) {
if (jrTemplateCommon.getPath() == null) {
JRTemplateSourceFile jrTemplateSourceFile = JRContext.findProfile().getJrTemplateSourceFile();
pathLocal = jrTemplateSourceFile.getPath();
} else
pathLocal = jrTemplateCommon.getPath();
} else
pathLocal = this.path;
Path pathToTemplate = Paths.get(pathLocal + (pathLocal == null || pathLocal.equals("") ? "" : '/') + name);
return Files.newInputStream(pathToTemplate);
} catch (Exception e) {
throw new JRTemplateException(e);
}
}
@JsonPOJOBuilder
public static class Builder {
@JsonProperty("name")
private String name;
@JsonProperty("path")
private String path;
public Builder withName(String name) {
this.name = name;
return this;
}
public Builder withPath(String path) {
this.path = path;
return this;
}
public JRTemplateSourceFile build() {
return new JRTemplateSourceFile(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy