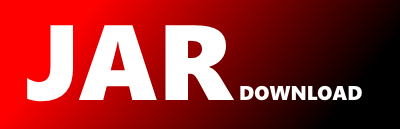
com.mntviews.upload.UploadContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mnt-upload Show documentation
Show all versions of mnt-upload Show documentation
Data upload library for the different types of databases
The newest version!
package com.mntviews.upload;
import com.mntviews.Uploadable;
import com.mntviews.model.UploadType;
import com.mntviews.upload.model.ConnectionData;
import java.io.File;
import static com.mntviews.Util.nvl;
public class UploadContext implements Uploadable {
private final String userName;
private final String password;
private final String url;
private final String script;
private final UploadType uploadType;
private final UploadDataBaseType uploadDataBaseType;
private UploadContext(Builder builder) {
this.userName = builder.userName;
this.password = builder.password;
this.url = builder.url;
this.script = builder.script;
this.uploadType = nvl(builder.uploadType, UploadType.TABLE);
this.uploadDataBaseType = builder.uploadDataBaseType;
}
public static Builder custom() {
return new Builder();
}
@Override
public void upload(File file) {
if (uploadType == UploadType.RAW)
uploadDataBaseType.uploadFile(new ConnectionData(url, userName, password), file, script.replace(UploadService.FILE_NAME_TEMPLATE, file.getAbsolutePath()));
else
uploadDataBaseType.upload(new ConnectionData(url, userName, password), file, script.replace(UploadService.FILE_NAME_TEMPLATE, file.getAbsolutePath()));
}
public static class Builder {
private String userName;
private String password;
private String url;
private String script;
private UploadType uploadType;
private UploadDataBaseType uploadDataBaseType;
public Builder withUserName(String userName) {
this.userName = userName;
return this;
}
public Builder withUploadType(UploadType uploadType) {
this.uploadType = uploadType;
return this;
}
public Builder withPassword(String password) {
this.password = password;
return this;
}
public Builder withUrl(String url) {
this.url = url;
return this;
}
public Builder withScript(String script) {
this.script = script;
return this;
}
public Builder withDataBaseType(UploadDataBaseType uploadDataBaseType) {
this.uploadDataBaseType = uploadDataBaseType;
return this;
}
public UploadContext build() {
return new UploadContext(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy