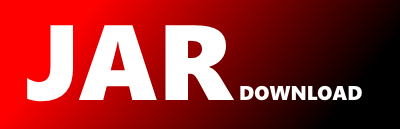
com.mntviews.upload.impl.OracleUploadServiceImpl Maven / Gradle / Ivy
package com.mntviews.upload.impl;
import com.mntviews.upload.UploadService;
import com.mntviews.upload.exception.OracleUploadServiceException;
import com.mntviews.upload.model.ConnectionData;
import lombok.extern.slf4j.Slf4j;
import java.io.*;
import java.nio.file.Files;
import java.util.UUID;
import java.util.concurrent.TimeUnit;
@Slf4j
public class OracleUploadServiceImpl implements UploadService {
private static final String CONTROL_FILE_EXT = ".ctrl";
private static final String LOG_FILE_EXT = ".log";
public static final int SQLLDR_TIMEOUT_MINUTES = 5;
public static final int SQLLDR_TIMEOUT_DESTROY_SECONDS = 10;
private static final int SQLLDR_TIMEOUT_FORCIBLY_DESTROY_SECONDS = 60;
@Override
public void upload(ConnectionData connectionData, File file, String script) {
String errorMessage = "";
String uuid = UUID.randomUUID().toString();
File controlFile = new File(file.getAbsolutePath() + "_" + uuid + CONTROL_FILE_EXT);
File logFile = new File(file.getAbsolutePath() + "_" + uuid + LOG_FILE_EXT);
String osName = System.getProperty("os.name");
try {
Process process = null;
boolean isExited = true;
try {
try (OutputStream outputStream = new FileOutputStream(controlFile)) {
outputStream.write(script.getBytes());
}
String command = String.format("sqlldr userid=%s@%s/%s control=%s log=%s"
, connectionData.getUserName()
, connectionData.getUrl()
, connectionData.getPassword()
, controlFile.getName()
, logFile.getName());
log.debug(command);
ProcessBuilder pb;
if (osName.contains("Windows")) {
pb = new ProcessBuilder("cmd.exe", "/c", command);
} else if (osName.contains("Linux")) {
pb = new ProcessBuilder("sh", "-c", command);
} else
throw new OracleUploadServiceException("Operation system not supported {osName=" + osName + "}");
pb.directory(new File(file.getParent()));
log.debug("pb.directory=" + pb.directory());
pb.redirectErrorStream(true);
process = pb.start();
StringBuilder sb = new StringBuilder();
try (BufferedReader inStreamReader = new BufferedReader(
new InputStreamReader(process.getInputStream()))) {
String line;
while ((line = inStreamReader.readLine()) != null) {
log.debug(line);
if (line.contains("SQL*Loader-") || line.contains("LRM"))
sb.append(line).append(System.lineSeparator());
}
}
isExited = process.waitFor(SQLLDR_TIMEOUT_MINUTES, TimeUnit.MINUTES);
if (!isExited)
throw new OracleUploadServiceException("sqlldr timeout expired {}");
if (process.exitValue() != 0) {
throw new OracleUploadServiceException("sqlldr exitValue!=0 {exitValue= " + process.exitValue() + "}");
}
try (BufferedReader reader = new BufferedReader(new InputStreamReader(new FileInputStream(logFile)))) {
String line;
while ((line = reader.readLine()) != null) {
log.debug(line);
if (line.contains("ORA-")) {
sb.append(line).append(System.lineSeparator());
}
}
}
errorMessage = sb.toString();
} catch (Exception e) {
throw new OracleUploadServiceException(e);
} finally {
Files.deleteIfExists(controlFile.toPath());
Files.deleteIfExists(logFile.toPath());
if (process != null && !isExited) {
process.destroy();
isExited = process.waitFor(SQLLDR_TIMEOUT_DESTROY_SECONDS, TimeUnit.SECONDS);
if (!isExited)
process.destroyForcibly();
isExited = process.waitFor(SQLLDR_TIMEOUT_FORCIBLY_DESTROY_SECONDS, TimeUnit.SECONDS);
if (!isExited)
log.debug("Process not destroyed");
}
}
} catch (Exception e) {
throw new OracleUploadServiceException(e);
}
if (!errorMessage.equals(""))
throw new OracleUploadServiceException(String.format("sqlldr error {%s}", errorMessage));
}
@Override
public void uploadFile(ConnectionData connectionData, File file, String script) {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy