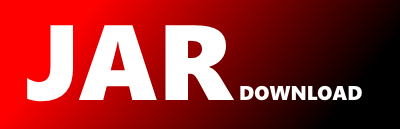
com.moandjiezana.toml.Toml Maven / Gradle / Ivy
package com.moandjiezana.toml;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.Reader;
import java.util.ArrayList;
import java.util.Date;
import java.util.HashMap;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.google.gson.Gson;
import com.google.gson.JsonElement;
/**
* Provides access to the keys and tables in a TOML data source.
*
* All getters can fall back to default values if they have been provided as a constructor argument.
* Getters for simple values (String, Date, etc.) will return null if no matching key exists.
* {@link #getList(String)}, {@link #getTable(String)} and {@link #getTables(String)} return empty values if there is no matching key.
*
* All read methods throw an {@link IllegalStateException} if the TOML is incorrect.
*
* Example usage:
*
* Toml toml = new Toml().read(getTomlFile());
* String name = toml.getString("name");
* Long port = toml.getLong("server.ip"); // compound key. Is equivalent to:
* Long port2 = toml.getTable("server").getLong("ip");
* MyConfig config = toml.to(MyConfig.class);
*
*
*/
public class Toml {
private static final Gson DEFAULT_GSON = new Gson();
private Map values = new HashMap();
private final Toml defaults;
/**
* Creates Toml instance with no defaults.
*/
public Toml() {
this(null);
}
/**
* @param defaults fallback values used when the requested key or table is not present in the TOML source that has been read.
*/
public Toml(Toml defaults) {
this(defaults, new HashMap());
}
/**
* Populates the current Toml instance with values from file.
*
* @param file The File to be read. Expected to be encoded as UTF-8.
* @return this instance
* @throws IllegalStateException If file contains invalid TOML
*/
public Toml read(File file) {
try {
return read(new InputStreamReader(new FileInputStream(file), "UTF8"));
} catch (Exception e) {
throw new RuntimeException(e);
}
}
/**
* Populates the current Toml instance with values from inputStream.
*
* @param inputStream Closed after it has been read.
* @return this instance
* @throws IllegalStateException If file contains invalid TOML
*/
public Toml read(InputStream inputStream) {
return read(new InputStreamReader(inputStream));
}
/**
* Populates the current Toml instance with values from reader.
*
* @param reader Closed after it has been read.
* @return this instance
* @throws IllegalStateException If file contains invalid TOML
*/
public Toml read(Reader reader) {
BufferedReader bufferedReader = null;
try {
bufferedReader = new BufferedReader(reader);
StringBuilder w = new StringBuilder();
String line = bufferedReader.readLine();
while (line != null) {
w.append(line).append('\n');
line = bufferedReader.readLine();
}
read(w.toString());
} catch (IOException e) {
throw new RuntimeException(e);
} finally {
try {
bufferedReader.close();
} catch (IOException e) {}
}
return this;
}
/**
* Populates the current Toml instance with values from otherToml.
*
* @param otherToml
* @return this instance
*/
public Toml read(Toml otherToml) {
this.values = otherToml.values;
return this;
}
/**
* Populates the current Toml instance with values from tomlString.
*
* @param tomlString String to be read.
* @return this instance
* @throws IllegalStateException If tomlString is not valid TOML
*/
public Toml read(String tomlString) throws IllegalStateException {
Results results = TomlParser.run(tomlString);
if (results.errors.hasErrors()) {
throw new IllegalStateException(results.errors.toString());
}
this.values = results.consume();
return this;
}
public String getString(String key) {
return (String) get(key);
}
public String getString(String key, String defaultValue) {
String val = getString(key);
return val == null ? defaultValue : val;
}
public Long getLong(String key) {
return (Long) get(key);
}
public Long getLong(String key, Long defaultValue) {
Long val = getLong(key);
return val == null ? defaultValue : val;
}
/**
* @param key a TOML key
* @param type of list items
* @return null
if the key is not found
*/
public List getList(String key) {
@SuppressWarnings("unchecked")
List list = (List) get(key);
return list;
}
/**
* @param key a TOML key
* @param defaultValue a list of default values
* @param type of list items
* @return null
is the key is not found
*/
public List getList(String key, List defaultValue) {
List list = getList(key);
return list != null ? list : defaultValue;
}
public Boolean getBoolean(String key) {
return (Boolean) get(key);
}
public Boolean getBoolean(String key, Boolean defaultValue) {
Boolean val = getBoolean(key);
return val == null ? defaultValue : val;
}
public Date getDate(String key) {
return (Date) get(key);
}
public Date getDate(String key, Date defaultValue) {
Date val = getDate(key);
return val == null ? defaultValue : val;
}
public Double getDouble(String key) {
return (Double) get(key);
}
public Double getDouble(String key, Double defaultValue) {
Double val = getDouble(key);
return val == null ? defaultValue : val;
}
/**
* @param key A table name, not including square brackets.
* @return A new Toml instance or null
if no value is found for key.
*/
@SuppressWarnings("unchecked")
public Toml getTable(String key) {
Map map = (Map) get(key);
return map != null ? new Toml(null, map) : null;
}
/**
* @param key Name of array of tables, not including square brackets.
* @return A {@link List} of Toml instances or null
if no value is found for key.
*/
@SuppressWarnings("unchecked")
public List getTables(String key) {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy