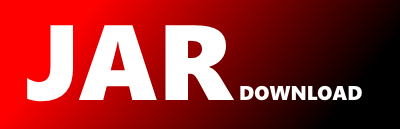
org.robovm.compiler.llvm.BasicBlock Maven / Gradle / Ivy
The newest version!
/*
* Copyright (C) 2012 RoboVM AB
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU General Public License
* as published by the Free Software Foundation; either version 2
* of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package org.robovm.compiler.llvm;
import java.io.IOException;
import java.io.Writer;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
/**
*
* @version $Id$
*/
public class BasicBlock implements Writable {
private final Function function;
private final Label label;
private final List instructions = new ArrayList();
BasicBlock(Function function, Label label) {
this.function = function;
this.label = label;
}
public BasicBlockRef ref() {
return new BasicBlockRef(function, label);
}
public String getName() {
return function.getLabel(this);
}
public Label getLabel() {
return label;
}
public Function getFunction() {
return function;
}
public Set getWritesTo() {
Set result = new HashSet();
for (Instruction i : instructions) {
result.addAll(i.getWritesTo());
}
return result;
}
public Set getReadsFrom() {
Set result = new HashSet();
for (Instruction i : instructions) {
result.addAll(i.getReadsFrom());
}
return result;
}
public void add(Instruction instruction) {
instructions.add(instruction);
instruction.basicBlock = this;
}
public void insertBefore(Instruction before, Instruction instruction) {
instructions.add(instructions.indexOf(before), instruction);
instruction.basicBlock = this;
}
public void insertAfter(Instruction after, Instruction instruction) {
instructions.add(instructions.indexOf(after) + 1, instruction);
instruction.basicBlock = this;
}
public List getInstructions() {
return instructions;
}
public Instruction first() {
if (instructions.isEmpty()) {
return null;
}
return instructions.get(0);
}
public Instruction last() {
if (instructions.isEmpty()) {
return null;
}
return instructions.get(instructions.size() - 1);
}
@Override
public void write(Writer writer) throws IOException {
writer.write(getName());
writer.write(":\n");
for (Instruction instruction : instructions) {
writer.write(" ");
instruction.write(writer);
List metadata = instruction.getMetadata();
if (!metadata.isEmpty()) {
for (Metadata md : metadata) {
writer.write(", ");
md.write(writer);
}
}
writer.write('\n');
}
}
@Override
public String toString() {
return toString(this::write);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy