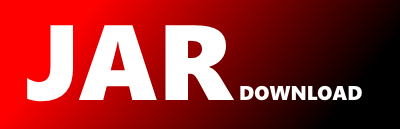
commonMain.com.harmony.kotlin.data.datasource.flow.FlowDataSourceMapper.kt Maven / Gradle / Ivy
package com.harmony.kotlin.data.datasource.flow
import com.harmony.kotlin.data.mapper.Mapper
import com.harmony.kotlin.data.mapper.map
import com.harmony.kotlin.data.query.Query
import kotlinx.coroutines.flow.Flow
import kotlinx.coroutines.flow.map
/**
* This data source uses mappers to map objects and redirects them to the contained data source, acting as a simple "translator".
*
* @param getDataSource Data source with get operations
* @param putDataSource Data source with put operations
* @param deleteDataSource Data source with delete operations
* @param toOutMapper Mapper to map data source objects to repository objects
* @param toInMapper Mapper to map repository objects to data source objects
*/
class FlowDataSourceMapper(
getDataSource: FlowGetDataSource,
putDataSource: FlowPutDataSource,
private val deleteDataSource: FlowDeleteDataSource,
toOutMapper: Mapper,
toInMapper: Mapper
) : FlowGetDataSource, FlowPutDataSource, FlowDeleteDataSource {
private val getDataSourceMapper = FlowGetDataSourceMapper(getDataSource, toOutMapper)
private val putDataSourceMapper = FlowPutDataSourceMapper(putDataSource, toOutMapper, toInMapper)
override fun get(query: Query): Flow = getDataSourceMapper.get(query)
@Deprecated("Use get instead")
override fun getAll(query: Query): Flow> = getDataSourceMapper.getAll(query)
override fun put(query: Query, value: Out?): Flow = putDataSourceMapper.put(query, value)
@Deprecated("Use put instead")
override fun putAll(query: Query, value: List?): Flow> = putDataSourceMapper.putAll(query, value)
override fun delete(query: Query): Flow = deleteDataSource.delete(query)
}
class FlowGetDataSourceMapper(
private val getDataSource: FlowGetDataSource,
private val toOutMapper: Mapper
) : FlowGetDataSource {
override fun get(query: Query): Flow = getDataSource.get(query).map { toOutMapper.map(it) }
@Deprecated("Use get instead")
override fun getAll(query: Query): Flow> = getDataSource.getAll(query).map { toOutMapper.map(it) }
}
class FlowPutDataSourceMapper(
private val putDataSource: FlowPutDataSource,
private val toOutMapper: Mapper,
private val toInMapper: Mapper
) : FlowPutDataSource {
override fun put(query: Query, value: Out?): Flow {
val mapped = value?.let { toInMapper.map(it) }
return putDataSource.put(query, mapped)
.map { toOutMapper.map(it) }
}
@Deprecated("Use put instead")
override fun putAll(query: Query, value: List?): Flow> {
val mapped = value?.map { toInMapper.map(it) }
return putDataSource.putAll(query, mapped)
.map { toOutMapper.map(it) }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy