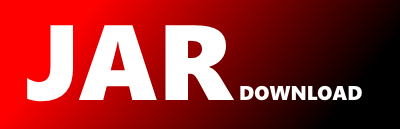
commonMain.com.harmony.kotlin.domain.interactor.FlowInteractor.kt Maven / Gradle / Ivy
package com.harmony.kotlin.domain.interactor
import com.harmony.kotlin.data.operation.DefaultOperation
import com.harmony.kotlin.data.operation.Operation
import com.harmony.kotlin.data.query.Query
import com.harmony.kotlin.data.query.VoidQuery
import com.harmony.kotlin.data.repository.flow.FlowDeleteRepository
import com.harmony.kotlin.data.repository.flow.FlowGetRepository
import com.harmony.kotlin.data.repository.flow.FlowPutRepository
import kotlinx.coroutines.CoroutineScope
import kotlinx.coroutines.Dispatchers
import kotlinx.coroutines.flow.Flow
class FlowGetInteractor(private val scope: CoroutineScope, private val getRepository: FlowGetRepository) {
operator fun invoke(query: Query = VoidQuery, operation: Operation = DefaultOperation): Flow = getRepository.get(query, operation)
}
@Deprecated(message = "Use FlowGetInteractor instead")
class FlowGetAllInteractor(private val scope: CoroutineScope, private val getRepository: FlowGetRepository) {
operator fun invoke(query: Query = VoidQuery, operation: Operation = DefaultOperation): Flow> = getRepository.getAll(query, operation)
}
class FlowPutInteractor(private val scope: CoroutineScope, private val putRepository: FlowPutRepository) {
operator fun invoke(m: M?, query: Query = VoidQuery, operation: Operation = DefaultOperation): Flow = putRepository.put(query, m, operation)
}
@Deprecated(message = "Use FlowPutInteractor instead")
class FlowPutAllInteractor(private val scope: CoroutineScope, private val putRepository: FlowPutRepository) {
operator fun invoke(m: List?, query: Query = VoidQuery, operation: Operation = DefaultOperation): Flow> = putRepository.putAll(
query, m,
operation
)
}
class FlowDeleteInteractor(private val scope: CoroutineScope, private val deleteRepository: FlowDeleteRepository) {
operator fun invoke(query: Query = VoidQuery, operation: Operation = DefaultOperation) = deleteRepository.delete(query, operation)
}
//region Creation
fun FlowGetRepository.toFlowGetInteractor(scope: CoroutineScope = CoroutineScope(Dispatchers.Default)) = FlowGetInteractor(scope, this)
@Deprecated(message = "Replaced by toFlowGetInteractor")
fun FlowGetRepository.toFlowGetAllInteractor(scope: CoroutineScope = CoroutineScope(Dispatchers.Default)) = FlowGetAllInteractor(scope, this)
fun FlowPutRepository.toFlowPutInteractor(scope: CoroutineScope = CoroutineScope(Dispatchers.Default)) = FlowPutInteractor(scope, this)
@Deprecated(message = "Replaced by toFlowPutInteractor")
fun FlowPutRepository.toFlowPutAllInteractor(scope: CoroutineScope = CoroutineScope(Dispatchers.Default)) = FlowPutAllInteractor(scope, this)
fun FlowDeleteRepository.toFlowDeleteInteractor(scope: CoroutineScope = CoroutineScope(Dispatchers.Default)) = FlowDeleteInteractor(scope, this)
//endregion
© 2015 - 2025 Weber Informatics LLC | Privacy Policy