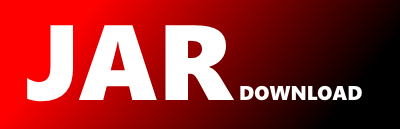
org.restcomm.protocols.ss7.tcapAnsi.TCAPStackImpl Maven / Gradle / Ivy
/*
* Mobius Software LTD
* Copyright 2019, Mobius Software LTD and individual contributors
* by the @authors tag.
*
* This program is free software: you can redistribute it and/or modify
* under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation; either version 3 of
* the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see
*/
package org.restcomm.protocols.ss7.tcapAnsi;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.Executors;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.atomic.AtomicLong;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import org.restcomm.protocols.ss7.sccp.SccpProvider;
import org.restcomm.protocols.ss7.tcapAnsi.api.TCAPProvider;
import org.restcomm.protocols.ss7.tcapAnsi.api.TCAPStack;
import org.restcomm.protocols.ss7.tcapAnsi.api.asn.comp.ComponentType;
import org.restcomm.protocols.ss7.tcapAnsi.api.asn.comp.PAbortCause;
import org.restcomm.protocols.ss7.tcapAnsi.api.asn.comp.RejectProblem;
import org.restcomm.protocols.ss7.tcapAnsi.asn.TCUnifiedMessageImpl;
/**
* @author amit bhayani
* @author baranowb
* @author yulianoifa
*
*/
public class TCAPStackImpl implements TCAPStack {
private final Logger logger;
protected static final String TCAP_MANAGEMENT_PERSIST_DIR_KEY = "tcapmanagement.persist.dir";
protected static final String USER_DIR_KEY = "user.dir";
protected static final String PERSIST_FILE_NAME = "management.xml";
// default value of idle timeout and after TC_END remove of task.
// TCAP state data, it is used ONLY on client side
protected TCAPProviderImpl tcapProvider;
protected ScheduledExecutorService service;
private final String name;
protected String persistDir = null;
private volatile boolean started = false;
private long dialogTimeout = _DIALOG_TIMEOUT;
private long invokeTimeout = _INVOKE_TIMEOUT;
// TODO: make this configurable
private long dialogIdRangeStart = 1;
private long dialogIdRangeEnd = Integer.MAX_VALUE;
private ConcurrentHashMap extraSsns = new ConcurrentHashMap();
private boolean isSwapTcapIdBytes = true; // for now configurable only via XML file
private int ssn = -1;
// SLS value
private SlsRangeType slsRange = SlsRangeType.All;
private ConcurrentHashMap messagesSentByType=new ConcurrentHashMap();
private ConcurrentHashMap messagesReceivedByType=new ConcurrentHashMap();
private ConcurrentHashMap componentsSentByType=new ConcurrentHashMap();
private ConcurrentHashMap componentsReceivedByType=new ConcurrentHashMap();
private ConcurrentHashMap rejectsSentByType=new ConcurrentHashMap();
private ConcurrentHashMap rejectsReceivedByType=new ConcurrentHashMap();
private ConcurrentHashMap abortsSentByType=new ConcurrentHashMap();
private ConcurrentHashMap abortsReceivedByType=new ConcurrentHashMap();
private AtomicLong incomingDialogsProcessed=new AtomicLong(0L);
private AtomicLong outgoingDialogsProcessed=new AtomicLong(0L);
private AtomicLong dialogTimeoutProcessed=new AtomicLong(0L);
private AtomicLong invokeTimeoutProcessed=new AtomicLong(0L);
private AtomicLong bytesSent=new AtomicLong(0L);
private AtomicLong bytesReceived=new AtomicLong(0L);
private ConcurrentHashMap> messagesSentByTypeAndNetwork=new ConcurrentHashMap>();
private ConcurrentHashMap> messagesReceivedByTypeAndNetwork=new ConcurrentHashMap>();
private ConcurrentHashMap> componentsSentByTypeAndNetwork=new ConcurrentHashMap>();
private ConcurrentHashMap> componentsReceivedByTypeAndNetwork=new ConcurrentHashMap>();
private ConcurrentHashMap> rejectsSentByTypeAndNetwork=new ConcurrentHashMap>();
private ConcurrentHashMap> rejectsReceivedByTypeAndNetwork=new ConcurrentHashMap>();
private ConcurrentHashMap> abortsSentByTypeAndNetwork=new ConcurrentHashMap>();
private ConcurrentHashMap> abortsReceivedByTypeAndNetwork=new ConcurrentHashMap>();
private ConcurrentHashMap incomingDialogsProcessedByNetwork=new ConcurrentHashMap();
private ConcurrentHashMap outgoingDialogsProcessedByNetwork=new ConcurrentHashMap();
private ConcurrentHashMap dialogTimeoutProcessedByNetwork=new ConcurrentHashMap();
private ConcurrentHashMap invokeTimeoutProcessedByNetwork=new ConcurrentHashMap();
private ConcurrentHashMap bytesSentByNetwork=new ConcurrentHashMap();
private ConcurrentHashMap bytesReceivedByNetwork=new ConcurrentHashMap();
public static List allAbortCauses=new ArrayList();
static
{
for(PAbortCause abortType:PAbortCause.values())
allAbortCauses.add(abortType.name());
allAbortCauses.add("User");
}
public TCAPStackImpl(String name,int threads) {
super();
this.name = name;
service=Executors.newScheduledThreadPool(threads);
this.logger = LogManager.getLogger(TCAPStackImpl.class.getCanonicalName() + "-" + this.name);
for(String currName:TCUnifiedMessageImpl.getAllNames()) {
messagesSentByType.put(currName,new AtomicLong(0));
messagesReceivedByType.put(currName,new AtomicLong(0));
}
for(ComponentType currComponentType:ComponentType.values()) {
componentsSentByType.put(currComponentType.name(),new AtomicLong(0));
componentsReceivedByType.put(currComponentType.name(),new AtomicLong(0));
}
for(RejectProblem rejectProblem:RejectProblem.values()) {
rejectsSentByType.put(rejectProblem.name(),new AtomicLong(0));
rejectsReceivedByType.put(rejectProblem.name(),new AtomicLong(0));
}
for(PAbortCause abortType:PAbortCause.values()) {
abortsReceivedByType.put(abortType.name(),new AtomicLong(0));
abortsSentByType.put(abortType.name(),new AtomicLong(0));
}
abortsReceivedByType.put("User",new AtomicLong(0));
abortsSentByType.put("User",new AtomicLong(0));
}
public TCAPStackImpl(String name, SccpProvider sccpProvider, int ssn,int threads) {
this(name,threads);
this.tcapProvider = new TCAPProviderImpl(sccpProvider, this, ssn,service);
this.ssn = ssn;
}
@Override
public String getName() {
return name;
}
@Override
public String getPersistDir() {
return persistDir;
}
@Override
public int getSubSystemNumber(){
return this.ssn;
}
public void start() throws Exception {
logger.info("Starting ..." + tcapProvider);
if (this.dialogTimeout < 0) {
throw new IllegalArgumentException("DialogIdleTimeout value must be greater or equal to zero.");
}
if (this.dialogTimeout < this.invokeTimeout) {
throw new IllegalArgumentException("DialogIdleTimeout value must be greater or equal to invoke timeout.");
}
if (this.invokeTimeout < 0) {
throw new IllegalArgumentException("InvokeTimeout value must be greater or equal to zero.");
}
tcapProvider.start();
this.started = true;
}
private void checkDialogIdRangeValues(long rangeStart, long rangeEnd) {
if (rangeStart >= rangeEnd)
throw new IllegalArgumentException("Range start value cannot be equal/greater than Range end value");
if (rangeStart < 1)
throw new IllegalArgumentException("Range start value must be greater or equal 1");
if (rangeEnd > Integer.MAX_VALUE)
throw new IllegalArgumentException("Range end value must be less or equal " + Integer.MAX_VALUE);
if ((rangeEnd - rangeStart) < 10000)
throw new IllegalArgumentException("Range \"end - start\" must has at least 10000 possible dialogs");
}
public void stop() {
this.tcapProvider.stop();
service.shutdownNow();
this.started = false;
}
/**
* @return the started
*/
public boolean isStarted() {
return this.started;
}
/*
* (non-Javadoc)
*
* @see org.restcomm.protocols.ss7.tcap.api.TCAPStack#getProvider()
*/
public TCAPProvider getProvider() {
return tcapProvider;
}
/*
* (non-Javadoc)
*
* @see org.restcomm.protocols.ss7.tcap.api.TCAPStack#setDialogIdleTimeout(long)
*/
public void setDialogIdleTimeout(long v) throws Exception {
if (!this.started)
throw new Exception("DialogIdleTimeout parameter can be updated only when TCAP stack is running");
if (v < 0) {
throw new IllegalArgumentException("DialogIdleTimeout value must be greater or equal to zero.");
}
if (v < this.invokeTimeout) {
throw new IllegalArgumentException("DialogIdleTimeout value must be greater or equal to invoke timeout.");
}
this.dialogTimeout = v;
}
/*
* (non-Javadoc)
*
* @see org.restcomm.protocols.ss7.tcap.api.TCAPStack#getDialogIdleTimeout()
*/
public long getDialogIdleTimeout() {
return this.dialogTimeout;
}
/*
* (non-Javadoc)
*
* @see org.restcomm.protocols.ss7.tcap.api.TCAPStack#setInvokeTimeout(long)
*/
public void setInvokeTimeout(long v) throws Exception {
if (!this.started)
throw new Exception("InvokeTimeout parameter can be updated only when TCAP stack is running");
if (v < 0) {
throw new IllegalArgumentException("InvokeTimeout value must be greater or equal to zero.");
}
if (v > this.dialogTimeout) {
throw new IllegalArgumentException("InvokeTimeout value must be smaller or equal to dialog timeout.");
}
this.invokeTimeout = v;
}
/*
* (non-Javadoc)
*
* @see org.restcomm.protocols.ss7.tcap.api.TCAPStack#getInvokeTimeout()
*/
public long getInvokeTimeout() {
return this.invokeTimeout;
}
public void setDialogIdRangeStart(long val) throws Exception {
if (!this.started)
throw new Exception("DialogIdRangeStart parameter can be updated only when TCAP stack is running");
this.checkDialogIdRangeValues(val, this.getDialogIdRangeEnd());
dialogIdRangeStart = val;
tcapProvider.resetDialogIdValueAfterRangeChange();
}
public void setDialogIdRangeEnd(long val) throws Exception {
if (!this.started)
throw new Exception("DialogIdRangeEnd parameter can be updated only when TCAP stack is running");
this.checkDialogIdRangeValues(this.getDialogIdRangeStart(), val);
dialogIdRangeEnd = val;
tcapProvider.resetDialogIdValueAfterRangeChange();
}
public long getDialogIdRangeStart() {
return dialogIdRangeStart;
}
public long getDialogIdRangeEnd() {
return dialogIdRangeEnd;
}
public void setExtraSsns(List extraSsnsNew) throws Exception {
if (this.started)
throw new Exception("ExtraSsns parameter can be updated only when TCAP stack is NOT running");
if (extraSsnsNew != null) {
ConcurrentHashMap extraSsnsTemp = new ConcurrentHashMap();
for(Integer ssn:extraSsnsNew)
extraSsnsTemp.put(ssn, ssn);
this.extraSsns = extraSsnsTemp;
}
}
public Collection getExtraSsns() {
return extraSsns.values();
}
public boolean isExtraSsnPresent(int ssn) {
if (this.ssn == ssn)
return true;
if (extraSsns != null) {
if (extraSsns.containsKey(ssn))
return true;
}
return false;
}
@Override
public String getSubSystemNumberList() {
StringBuilder sb = new StringBuilder();
sb.append(this.ssn);
if (extraSsns != null) {
Iterator iterator=extraSsns.values().iterator();
while(iterator.hasNext()) {
sb.append(", ");
sb.append(iterator.next());
}
}
return sb.toString();
}
public void setSlsRange(String val) throws Exception {
if (val.equals(SlsRangeType.All.toString())) {
this.slsRange = SlsRangeType.All;
} else if (val.equals(SlsRangeType.Odd.toString())) {
this.slsRange = SlsRangeType.Odd;
} else if (val.equals(SlsRangeType.Even.toString())) {
this.slsRange = SlsRangeType.Even;
} else {
throw new Exception("SlsRange value is invalid");
}
}
public String getSlsRange() {
return this.slsRange.toString();
}
@Override
public boolean getSwapTcapIdBytes() {
return isSwapTcapIdBytes;
}
@Override
public void setSwapTcapIdBytes(boolean isSwapTcapIdBytes) {
this.isSwapTcapIdBytes = isSwapTcapIdBytes;
}
public SlsRangeType getSlsRangeType() {
return this.slsRange;
}
@Override
public Map getComponentsSentByType() {
Map result=new HashMap();
Iterator> iterator=componentsSentByType.entrySet().iterator();
while(iterator.hasNext()) {
Entry currEntry=iterator.next();
result.put(currEntry.getKey(), currEntry.getValue().get());
}
return result;
}
@Override
public Map getComponentsReceivedByType() {
Map result=new HashMap();
Iterator> iterator=componentsReceivedByType.entrySet().iterator();
while(iterator.hasNext()) {
Entry currEntry=iterator.next();
result.put(currEntry.getKey(), currEntry.getValue().get());
}
return result;
}
@Override
public Map getMessagesSentByType() {
Map result=new HashMap();
Iterator> iterator=messagesSentByType.entrySet().iterator();
while(iterator.hasNext()) {
Entry currEntry=iterator.next();
result.put(currEntry.getKey(), currEntry.getValue().get());
}
return result;
}
@Override
public Map getMessagesReceivedByType() {
Map result=new HashMap();
Iterator> iterator=messagesReceivedByType.entrySet().iterator();
while(iterator.hasNext()) {
Entry currEntry=iterator.next();
result.put(currEntry.getKey(), currEntry.getValue().get());
}
return result;
}
@Override
public Map getRejectsSentByType() {
Map result=new HashMap();
Iterator> iterator=rejectsSentByType.entrySet().iterator();
while(iterator.hasNext()) {
Entry currEntry=iterator.next();
result.put(currEntry.getKey(), currEntry.getValue().get());
}
return result;
}
@Override
public Map getRejectsReceivedByType() {
Map result=new HashMap();
Iterator> iterator=rejectsReceivedByType.entrySet().iterator();
while(iterator.hasNext()) {
Entry currEntry=iterator.next();
result.put(currEntry.getKey(), currEntry.getValue().get());
}
return result;
}
@Override
public Map getAbortsSentByType() {
Map result=new HashMap();
Iterator> iterator=abortsSentByType.entrySet().iterator();
while(iterator.hasNext()) {
Entry currEntry=iterator.next();
result.put(currEntry.getKey(), currEntry.getValue().get());
}
return result;
}
@Override
public Map getAbortsReceivedByType() {
Map result=new HashMap();
Iterator> iterator=abortsReceivedByType.entrySet().iterator();
while(iterator.hasNext()) {
Entry currEntry=iterator.next();
result.put(currEntry.getKey(), currEntry.getValue().get());
}
return result;
}
@Override
public Long getIncomingDialogsProcessed() {
return incomingDialogsProcessed.get();
}
@Override
public Long getOutgoingDialogsProcessed() {
return outgoingDialogsProcessed.get();
}
@Override
public Long getBytesSent() {
return bytesSent.get();
}
@Override
public Long getBytesReceived() {
return bytesReceived.get();
}
@Override
public Long getDialogTimeoutProcessed() {
return dialogTimeoutProcessed.get();
}
@Override
public Long getInvokeTimeoutProcessed() {
return invokeTimeoutProcessed.get();
}
@Override
public Map getComponentsSentByTypeAndNetwork(Integer networkID) {
Map result=new HashMap();
Map componentsSentByType = componentsSentByTypeAndNetwork.get(networkID);
if(componentsSentByType!=null) {
Iterator> iterator=componentsSentByType.entrySet().iterator();
while(iterator.hasNext()) {
Entry currEntry=iterator.next();
result.put(currEntry.getKey(), currEntry.getValue().get());
}
}
return result;
}
@Override
public Map getComponentsReceivedByTypeAndNetwork(Integer networkID) {
Map result=new HashMap();
Map componentsReceivedByType = componentsReceivedByTypeAndNetwork.get(networkID);
if(componentsReceivedByType!=null) {
Iterator> iterator=componentsReceivedByType.entrySet().iterator();
while(iterator.hasNext()) {
Entry currEntry=iterator.next();
result.put(currEntry.getKey(), currEntry.getValue().get());
}
}
return result;
}
@Override
public Map getMessagesSentByTypeAndNetwork(Integer networkID) {
Map result=new HashMap();
Map messagesSentByType = messagesSentByTypeAndNetwork.get(networkID);
if(messagesSentByType!=null) {
Iterator> iterator=messagesSentByType.entrySet().iterator();
while(iterator.hasNext()) {
Entry currEntry=iterator.next();
result.put(currEntry.getKey(), currEntry.getValue().get());
}
}
return result;
}
@Override
public Map getMessagesReceivedByTypeAndNetwork(Integer networkID) {
Map result=new HashMap();
Map messagesReceivedByType = messagesReceivedByTypeAndNetwork.get(networkID);
if(messagesReceivedByType!=null) {
Iterator> iterator=messagesReceivedByType.entrySet().iterator();
while(iterator.hasNext()) {
Entry currEntry=iterator.next();
result.put(currEntry.getKey(), currEntry.getValue().get());
}
}
return result;
}
@Override
public Map getRejectsSentByTypeAndNetwork(Integer networkID) {
Map result=new HashMap();
Map rejectsSentByType = rejectsSentByTypeAndNetwork.get(networkID);
if(rejectsSentByType!=null) {
Iterator> iterator=rejectsSentByType.entrySet().iterator();
while(iterator.hasNext()) {
Entry currEntry=iterator.next();
result.put(currEntry.getKey(), currEntry.getValue().get());
}
}
return result;
}
@Override
public Map getRejectsReceivedByTypeAndNetwork(Integer networkID) {
Map result=new HashMap();
Map rejectsReceivedByType = rejectsReceivedByTypeAndNetwork.get(networkID);
if(rejectsReceivedByType!=null) {
Iterator> iterator=rejectsReceivedByType.entrySet().iterator();
while(iterator.hasNext()) {
Entry currEntry=iterator.next();
result.put(currEntry.getKey(), currEntry.getValue().get());
}
}
return result;
}
@Override
public Map getAbortsSentByTypeAndNetwork(Integer networkID) {
Map result=new HashMap();
Map abortsSentByType = abortsSentByTypeAndNetwork.get(networkID);
if(abortsSentByType!=null) {
Iterator> iterator=abortsSentByType.entrySet().iterator();
while(iterator.hasNext()) {
Entry currEntry=iterator.next();
result.put(currEntry.getKey(), currEntry.getValue().get());
}
}
return result;
}
@Override
public Map getAbortsReceivedByTypeAndNetwork(Integer networkID) {
Map result=new HashMap();
Map abortsReceivedByType = abortsReceivedByTypeAndNetwork.get(networkID);
if(abortsReceivedByType!=null) {
Iterator> iterator=abortsReceivedByType.entrySet().iterator();
while(iterator.hasNext()) {
Entry currEntry=iterator.next();
result.put(currEntry.getKey(), currEntry.getValue().get());
}
}
return result;
}
@Override
public Long getIncomingDialogsProcessedByNetwork(Integer networkID) {
AtomicLong result = incomingDialogsProcessedByNetwork.get(networkID);
if(result==null)
return 0L;
return result.get();
}
@Override
public Long getOutgoingDialogsProcessedByNetwork(Integer networkID) {
AtomicLong result = outgoingDialogsProcessedByNetwork.get(networkID);
if(result==null)
return 0L;
return result.get();
}
@Override
public Long getBytesSentByNetwork(Integer networkID) {
AtomicLong result = bytesSentByNetwork.get(networkID);
if(result==null)
return 0L;
return result.get();
}
@Override
public Long getBytesReceivedByNetwork(Integer networkID) {
AtomicLong result = bytesReceivedByNetwork.get(networkID);
if(result==null)
return 0L;
return result.get();
}
@Override
public Long getDialogTimeoutProcessedByNetwork(Integer networkID) {
AtomicLong result = dialogTimeoutProcessedByNetwork.get(networkID);
if(result==null)
return 0L;
return result.get();
}
@Override
public Long getInvokeTimeoutProcessedByNetwork(Integer networkID) {
AtomicLong result = invokeTimeoutProcessedByNetwork.get(networkID);
if(result==null)
return 0L;
return result.get();
}
protected void newIncomingDialogProcessed(int networkID) {
incomingDialogsProcessed.incrementAndGet();
AtomicLong incomingDialogsProcessed = incomingDialogsProcessedByNetwork.get(networkID);
if(incomingDialogsProcessed==null) {
incomingDialogsProcessed=new AtomicLong();
AtomicLong oldValue=incomingDialogsProcessedByNetwork.putIfAbsent(networkID, incomingDialogsProcessed);
if(oldValue!=null)
incomingDialogsProcessed=oldValue;
}
incomingDialogsProcessed.incrementAndGet();
}
protected void newOutgoingDialogProcessed(int networkID) {
outgoingDialogsProcessed.incrementAndGet();
AtomicLong outgoingDialogsProcessed = outgoingDialogsProcessedByNetwork.get(networkID);
if(outgoingDialogsProcessed==null) {
outgoingDialogsProcessed=new AtomicLong();
AtomicLong oldValue=outgoingDialogsProcessedByNetwork.putIfAbsent(networkID, outgoingDialogsProcessed);
if(oldValue!=null)
invokeTimeoutProcessed=oldValue;
}
outgoingDialogsProcessed.incrementAndGet();
}
protected void newComponentSent(String componentName,int networkID) {
componentsSentByType.get(componentName).incrementAndGet();
ConcurrentHashMap componentsSentByType =componentsSentByTypeAndNetwork.get(networkID);
if(componentsSentByType==null) {
componentsSentByType=new ConcurrentHashMap();
for(ComponentType currComponentType:ComponentType.values())
componentsSentByType.put(currComponentType.name(),new AtomicLong(0));
ConcurrentHashMap oldValue=componentsSentByTypeAndNetwork.putIfAbsent(networkID, componentsSentByType);
if(oldValue!=null)
componentsSentByType=oldValue;
}
componentsSentByType.get(componentName).incrementAndGet();
}
protected void newComponentReceived(String componentName,int networkID) {
componentsReceivedByType.get(componentName).incrementAndGet();
ConcurrentHashMap componentsReceivedByType =componentsReceivedByTypeAndNetwork.get(networkID);
if(componentsReceivedByType==null) {
componentsReceivedByType=new ConcurrentHashMap();
for(ComponentType currComponentType:ComponentType.values())
componentsReceivedByType.put(currComponentType.name(),new AtomicLong(0));
ConcurrentHashMap oldValue=componentsReceivedByTypeAndNetwork.putIfAbsent(networkID, componentsReceivedByType);
if(oldValue!=null)
componentsReceivedByType=oldValue;
}
componentsReceivedByType.get(componentName).incrementAndGet();
}
protected void newRejectSent(String rejectReason,int networkID) {
rejectsSentByType.get(rejectReason).incrementAndGet();
ConcurrentHashMap rejectsSentByType =rejectsSentByTypeAndNetwork.get(networkID);
if(rejectsSentByType==null) {
rejectsSentByType=new ConcurrentHashMap();
for(RejectProblem rejectProblem:RejectProblem.values())
rejectsSentByType.put(rejectProblem.name(),new AtomicLong(0));
ConcurrentHashMap oldValue=rejectsSentByTypeAndNetwork.putIfAbsent(networkID, rejectsSentByType);
if(oldValue!=null)
rejectsSentByType=oldValue;
}
rejectsSentByType.get(rejectReason).incrementAndGet();
}
protected void newRejectReceived(String rejectReason,int networkID) {
rejectsReceivedByType.get(rejectReason).incrementAndGet();
ConcurrentHashMap rejectsReceivedByType =rejectsReceivedByTypeAndNetwork.get(networkID);
if(rejectsReceivedByType==null) {
rejectsReceivedByType=new ConcurrentHashMap();
for(RejectProblem rejectProblem:RejectProblem.values())
rejectsReceivedByType.put(rejectProblem.name(),new AtomicLong(0));
ConcurrentHashMap oldValue=rejectsReceivedByTypeAndNetwork.putIfAbsent(networkID, rejectsReceivedByType);
if(oldValue!=null)
rejectsReceivedByType=oldValue;
}
rejectsReceivedByType.get(rejectReason).incrementAndGet();
}
protected void newAbortSent(String abortCause,int networkID) {
abortsSentByType.get(abortCause).incrementAndGet();
ConcurrentHashMap abortsSentByType =abortsSentByTypeAndNetwork.get(networkID);
if(abortsSentByType==null) {
abortsSentByType=new ConcurrentHashMap();
for(PAbortCause abortType:PAbortCause.values())
abortsSentByType.put(abortType.name(),new AtomicLong(0));
abortsSentByType.put("User",new AtomicLong(0));
ConcurrentHashMap oldValue=abortsSentByTypeAndNetwork.putIfAbsent(networkID, abortsSentByType);
if(oldValue!=null)
abortsSentByType=oldValue;
}
abortsSentByType.get(abortCause).incrementAndGet();
}
protected void newAbortReceived(String abortCause,Integer networkID) {
abortsReceivedByType.get(abortCause).incrementAndGet();
if(networkID!=null) {
ConcurrentHashMap abortsReceivedByType =abortsReceivedByTypeAndNetwork.get(networkID);
if(abortsReceivedByType==null) {
abortsReceivedByType=new ConcurrentHashMap();
for(PAbortCause abortType:PAbortCause.values())
abortsReceivedByType.put(abortType.name(),new AtomicLong(0));
abortsReceivedByType.put("User",new AtomicLong(0));
ConcurrentHashMap oldValue=abortsReceivedByTypeAndNetwork.putIfAbsent(networkID, abortsReceivedByType);
if(oldValue!=null)
abortsReceivedByType=oldValue;
}
abortsReceivedByType.get(abortCause).incrementAndGet();
}
}
protected void newMessageSent(String messageType,int bytes,int networkID) {
messagesSentByType.get(messageType).incrementAndGet();
bytesSent.addAndGet(bytes);
ConcurrentHashMap messagesSentByType =messagesSentByTypeAndNetwork.get(networkID);
if(messagesSentByType==null) {
messagesSentByType=new ConcurrentHashMap();
for(String currName:TCUnifiedMessageImpl.getAllNames())
messagesSentByType.put(currName,new AtomicLong(0));
ConcurrentHashMap oldValue=messagesSentByTypeAndNetwork.putIfAbsent(networkID, messagesSentByType);
if(oldValue!=null)
messagesSentByType=oldValue;
}
messagesSentByType.get(messageType).incrementAndGet();
AtomicLong bytesSent = bytesSentByNetwork.get(networkID);
if(bytesSent==null) {
bytesSent=new AtomicLong();
AtomicLong oldValue=bytesSentByNetwork.putIfAbsent(networkID, bytesSent);
if(oldValue!=null)
bytesSent=oldValue;
}
bytesSent.addAndGet(bytes);
}
protected void newMessageReceived(String messageType,int bytes,int networkID) {
messagesReceivedByType.get(messageType).incrementAndGet();
bytesReceived.addAndGet(bytes);
ConcurrentHashMap messagesReceivedByType =messagesReceivedByTypeAndNetwork.get(networkID);
if(messagesReceivedByType==null) {
messagesReceivedByType=new ConcurrentHashMap();
for(String currName:TCUnifiedMessageImpl.getAllNames())
messagesReceivedByType.put(currName,new AtomicLong(0));
ConcurrentHashMap oldValue=messagesReceivedByTypeAndNetwork.putIfAbsent(networkID, messagesReceivedByType);
if(oldValue!=null)
messagesReceivedByType=oldValue;
}
messagesReceivedByType.get(messageType).incrementAndGet();
AtomicLong bytesReceived = bytesReceivedByNetwork.get(networkID);
if(bytesReceived==null) {
bytesReceived=new AtomicLong();
AtomicLong oldValue=bytesReceivedByNetwork.putIfAbsent(networkID, bytesReceived);
if(oldValue!=null)
bytesReceived=oldValue;
}
bytesReceived.addAndGet(bytes);
}
protected void dialogTimedOut(int networkID) {
dialogTimeoutProcessed.incrementAndGet();
AtomicLong dialogTimeoutProcessed = dialogTimeoutProcessedByNetwork.get(networkID);
if(dialogTimeoutProcessed==null) {
dialogTimeoutProcessed=new AtomicLong();
AtomicLong oldValue=dialogTimeoutProcessedByNetwork.putIfAbsent(networkID, dialogTimeoutProcessed);
if(oldValue!=null)
dialogTimeoutProcessed=oldValue;
}
dialogTimeoutProcessed.incrementAndGet();
}
protected void invokeTimedOut(int networkID) {
invokeTimeoutProcessed.incrementAndGet();
AtomicLong invokeTimeoutProcessed = invokeTimeoutProcessedByNetwork.get(networkID);
if(invokeTimeoutProcessed==null) {
invokeTimeoutProcessed=new AtomicLong();
AtomicLong oldValue=invokeTimeoutProcessedByNetwork.putIfAbsent(networkID, invokeTimeoutProcessed);
if(oldValue!=null)
invokeTimeoutProcessed=oldValue;
}
invokeTimeoutProcessed.incrementAndGet();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy