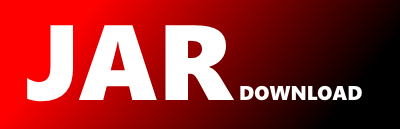
com.mockrunner.example.jdbc.BankTest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mockrunner-jdk1.3-j2ee1.3 Show documentation
Show all versions of mockrunner-jdk1.3-j2ee1.3 Show documentation
Mockrunner is a lightweight framework for unit testing applications
in the J2EE environment. It supports servlets, filters, tag classes
and Struts actions. It includes a JDBC a JMS and a JCA test
framework and can be used to test EJB based applications.
The newest version!
package com.mockrunner.example.jdbc;
import java.sql.SQLException;
import com.mockrunner.jdbc.BasicJDBCTestCaseAdapter;
import com.mockrunner.jdbc.StatementResultSetHandler;
import com.mockrunner.mock.jdbc.MockResultSet;
/**
* Example test for {@link Bank}. Demonstrates the usage of
* {@link com.mockrunner.jdbc.JDBCTestModule}
* and {@link com.mockrunner.jdbc.BasicJDBCTestCaseAdapter}.
* Please note that the framework does not execute any SQL statements.
* You have to specify the MockResultSet
of the select
* statement. Since there is only one select in this test, the
* MockResultSet
is set as the global one.
* The Java API is used to add one row to the MockResultSet
.
* It's also possible to read the test tables from text files.
* This test covers a valid transaction test
* (there's enough money on the source account) and the failure case.
* You do not have to specify the exact SQL statements,
* select balance is ok for select balance from account where id=1.
* You can specify the search parameters of SQL statements with
* {@link com.mockrunner.jdbc.JDBCTestModule#setExactMatch} and
* {@link com.mockrunner.jdbc.JDBCTestModule#setCaseSensitive}.
* The default is false
for both.
*/
public class BankTest extends BasicJDBCTestCaseAdapter
{
private void prepareEmptyResultSet()
{
StatementResultSetHandler statementHandler = getJDBCMockObjectFactory().getMockConnection().getStatementResultSetHandler();
MockResultSet result = statementHandler.createResultSet();
statementHandler.prepareGlobalResultSet(result);
}
private void prepareResultSet()
{
StatementResultSetHandler statementHandler = getJDBCMockObjectFactory().getMockConnection().getStatementResultSetHandler();
MockResultSet result = statementHandler.createResultSet();
result.addRow(new Integer[] {new Integer(10000)});
statementHandler.prepareGlobalResultSet(result);
}
public void testWrongId() throws SQLException
{
prepareEmptyResultSet();
Bank bank = new Bank();
bank.connect();
bank.transfer(1, 2, 5000);
bank.disconnect();
verifySQLStatementExecuted("select balance");
verifySQLStatementNotExecuted("update account");
verifyNotCommitted();
verifyRolledBack();
verifyAllResultSetsClosed();
verifyAllStatementsClosed();
verifyConnectionClosed();
}
public void testTransferOk() throws SQLException
{
prepareResultSet();
Bank bank = new Bank();
bank.connect();
bank.transfer(1, 2, 5000);
bank.disconnect();
verifySQLStatementExecuted("select balance");
verifySQLStatementExecuted("update account");
verifySQLStatementParameter("update account", 0, 1, new Integer(-5000));
verifySQLStatementParameter("update account", 0, 2, new Integer(1));
verifySQLStatementParameter("update account", 1, 1, new Integer(5000));
verifySQLStatementParameter("update account", 1, 2, new Integer(2));
verifyCommitted();
verifyNotRolledBack();
verifyAllResultSetsClosed();
verifyAllStatementsClosed();
verifyConnectionClosed();
}
public void testTransferFailure() throws SQLException
{
prepareResultSet();
Bank bank = new Bank();
bank.connect();
bank.transfer(1, 2, 20000);
bank.disconnect();
verifySQLStatementExecuted("select balance");
verifySQLStatementNotExecuted("update account");
verifyNotCommitted();
verifyRolledBack();
verifyAllResultSetsClosed();
verifyAllStatementsClosed();
verifyConnectionClosed();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy