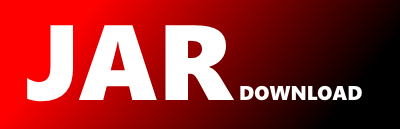
com.mockrunner.jdbc.AbstractOutParameterResultSetHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mockrunner-jdk1.3-j2ee1.3 Show documentation
Show all versions of mockrunner-jdk1.3-j2ee1.3 Show documentation
Mockrunner is a lightweight framework for unit testing applications
in the J2EE environment. It supports servlets, filters, tag classes
and Struts actions. It includes a JDBC a JMS and a JCA test
framework and can be used to test EJB based applications.
The newest version!
package com.mockrunner.jdbc;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.TreeMap;
/**
* Abstract base class for all statement types
* that support out parameters, i.e. CallableStatement
.
*/
public abstract class AbstractOutParameterResultSetHandler extends AbstractParameterResultSetHandler
{
private boolean mustRegisterOutParameters = false;
private Map globalOutParameter = null;
private Map outParameterForStatement = new TreeMap();
private Map outParameterForStatementParameters = new TreeMap();
/**
* Set if out parameters must be registered to be returned.
* The default is false
, i.e. if there are matching
* out parameters prepared, they are returned even if the
* registerOutParameter
methods of CallableStatement
* have not been called. If set to true
, registerOutParameter
* must be called.
* @param mustOutParameterBeRegistered must out parameter be registered
*/
public void setMustRegisterOutParameters(boolean mustOutParameterBeRegistered)
{
this.mustRegisterOutParameters = mustOutParameterBeRegistered;
}
/**
* Get if out parameter must be registered to be returned.
* @return must out parameter be registered
*/
public boolean getMustRegisterOutParameters()
{
return mustRegisterOutParameters;
}
/**
* Returns the first out parameter Map
that matches
* the specified SQL string.
* Please note that you can modify the match parameters with
* {@link #setCaseSensitive}, {@link #setExactMatch} and
* {@link #setUseRegularExpressions}.
* @param sql the SQL string
* @return the corresponding out parameter Map
*/
public Map getOutParameter(String sql)
{
SQLStatementMatcher matcher = new SQLStatementMatcher(getCaseSensitive(), getExactMatch(), getUseRegularExpressions());
List list = matcher.getMatchingObjects(outParameterForStatement, sql, true, true);
if(null != list && list.size() > 0)
{
return (Map)list.get(0);
}
return null;
}
/**
* Returns the first out parameter Map
that matches
* the specified SQL string and the specified parameters.
* Please note that you can modify the match parameters with
* {@link #setCaseSensitive}, {@link #setExactMatch} and
* {@link #setUseRegularExpressions} and the match parameters for the
* specified parameter list with {@link #setExactMatchParameter}.
* @param sql the SQL string
* @param parameters the parameters
* @return the corresponding out parameter Map
*/
public Map getOutParameter(String sql, Map parameters)
{
MockOutParameterWrapper wrapper = (MockOutParameterWrapper)getMatchingParameterWrapper(sql, parameters, outParameterForStatementParameters);
if(null != wrapper)
{
return wrapper.getOutParameter();
}
return null;
}
/**
* Clears the out parameters.
*/
public void clearOutParameter()
{
outParameterForStatement.clear();
outParameterForStatementParameters.clear();
}
/**
* Returns the global out parameter Map
.
* @return the global out parameter Map
*/
public Map getGlobalOutParameter()
{
return globalOutParameter;
}
/**
* Prepares the global out parameter Map
.
* @param outParameters the global out parameter Map
*/
public void prepareGlobalOutParameter(Map outParameters)
{
globalOutParameter = new HashMap(outParameters);
}
/**
* Prepare an out parameter Map
for a specified
* SQL string.
* Please note that you can modify the match parameters with
* {@link #setCaseSensitive}, {@link #setExactMatch} and
* {@link #setUseRegularExpressions}.
* @param sql the SQL string
* @param outParameters the out parameter Map
*/
public void prepareOutParameter(String sql, Map outParameters)
{
outParameterForStatement.put(sql, new HashMap(outParameters));
}
/**
* Prepare an out parameter Map
for a specified SQL string and
* the specified parameters. The specified parameters array
* must contain the parameters in the correct order starting with index 0 for
* the first parameter. Please keep in mind that parameters in
* CallableStatement
objects start with 1 as the first
* parameter. So parameters[0]
maps to the
* parameter with index 1.
* Please note that you can modify the match parameters with
* {@link #setCaseSensitive}, {@link #setExactMatch} and
* {@link #setUseRegularExpressions} and the match parameters for the
* specified parameter list with {@link #setExactMatchParameter}.
* @param sql the SQL string
* @param outParameters the corresponding out parameter Map
* @param parameters the parameters
*/
public void prepareOutParameter(String sql, Map outParameters, Object[] parameters)
{
prepareOutParameter(sql, outParameters, Arrays.asList(parameters));
}
/**
* Prepare an out parameter Map
for a specified SQL string and
* the specified parameters. The specified parameters array
* must contain the parameters in the correct order starting with index 0 for
* the first parameter. Please keep in mind that parameters in
* CallableStatement
objects start with 1 as the first
* parameter. So parameters.get(0)
maps to the
* parameter with index 1.
* Please note that you can modify the match parameters with
* {@link #setCaseSensitive}, {@link #setExactMatch} and
* {@link #setUseRegularExpressions} and the match parameters for the
* specified parameter list with {@link #setExactMatchParameter}.
* @param sql the SQL string
* @param outParameters the corresponding out parameter Map
* @param parameters the parameters
*/
public void prepareOutParameter(String sql, Map outParameters, List parameters)
{
Map params = new HashMap();
for(int ii = 0; ii < parameters.size(); ii++)
{
params.put(new Integer(ii + 1), parameters.get(ii));
}
prepareOutParameter(sql, outParameters, params);
}
/**
* Prepare an out parameter Map
for a specified SQL string
* and the specified parameters. The specified parameters Map
* must contain the parameters by mapping Integer
or
* String
objects to the corresponding parameter.
* An Integer
object is the index of the parameter.
* A String
is the name of the parameter.
* Please note that you can modify the match parameters with
* {@link #setCaseSensitive}, {@link #setExactMatch} and
* {@link #setUseRegularExpressions} and the match parameters for the
* specified parameter list with {@link #setExactMatchParameter}.
* @param sql the SQL string
* @param outParameters the corresponding out parameter Map
* @param parameters the parameters
*/
public void prepareOutParameter(String sql, Map outParameters, Map parameters)
{
List list = (List)outParameterForStatementParameters.get(sql);
if(null == list)
{
list = new ArrayList();
outParameterForStatementParameters.put(sql, list);
}
list.add(new MockOutParameterWrapper(new HashMap(outParameters), new HashMap(parameters)));
}
private class MockOutParameterWrapper extends ParameterWrapper
{
private Map outParameter;
public MockOutParameterWrapper(Map outParameter, Map parameters)
{
super(parameters);
this.outParameter = outParameter;
}
public Map getOutParameter()
{
return outParameter;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy