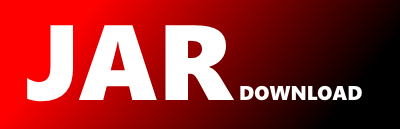
com.mockrunner.mock.web.WebMockObjectFactory Maven / Gradle / Ivy
package com.mockrunner.mock.web;
import java.lang.reflect.Constructor;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.jsp.JspFactory;
import com.mockrunner.base.NestedApplicationException;
/**
* Used to create all types of web mock objects. Maintains
* the necessary dependencies between the mock objects.
* If you use the mock objects returned by this
* factory in your tests you can be sure that they are all
* up to date.
*/
public class WebMockObjectFactory
{
private HttpServletRequest wrappedRequest;
private HttpServletResponse wrappedResponse;
private MockHttpServletRequest request;
private MockHttpServletResponse response;
private MockServletConfig config;
private MockServletContext context;
private MockHttpSession session;
private MockPageContext pageContext;
private MockFilterConfig filterConfig;
private MockFilterChain filterChain;
private JspFactory jspFactory;
/**
* Creates a new set of mock objects.
*/
public WebMockObjectFactory()
{
createMockObjects();
}
/**
* Creates a set of mock objects based on another one.
* The created mock objects will have their own
* request and session objects, but they will share
* one ServletContext
.
* @param factory the other factory
*/
public WebMockObjectFactory(WebMockObjectFactory factory)
{
createMockObjectsBasedOn(factory);
}
/**
* Creates a set of mock objects based on another one.
* You can specify, if the created mock objects should
* share the same session. They will share one
* ServletContext
anyway.
* @param factory the other factory
* @param createNewSession true
creates a new session,
* false
uses the session from factory
*/
public WebMockObjectFactory(WebMockObjectFactory factory, boolean createNewSession)
{
createMockObjectsBasedOn(factory, createNewSession);
}
private void createMockObjects()
{
createNewMockObjects(true);
context = createMockServletContext();
setUpDependencies();
JspFactory.setDefaultFactory(jspFactory);
}
private void createMockObjectsBasedOn(WebMockObjectFactory factory)
{
createMockObjectsBasedOn(factory, true);
}
private void createMockObjectsBasedOn(WebMockObjectFactory factory, boolean createNewSession)
{
createNewMockObjects(createNewSession);
if(!createNewSession) session = factory.getMockSession();
context = factory.getMockServletContext();
setUpDependencies();
JspFactory.setDefaultFactory(jspFactory);
}
private void createNewMockObjects(boolean createNewSession)
{
request = createMockRequest();
response = createMockResponse();
wrappedRequest = request;
wrappedResponse = response;
if(createNewSession) session = createMockSession();
config = createMockServletConfig();
filterChain = createMockFilterChain();
filterConfig = createMockFilterConfig();
jspFactory = createMockJspFactory();
}
private void setUpDependencies()
{
config.setServletContext(context);
request.setSession(session);
session.setupServletContext(context);
pageContext = createMockPageContext();
pageContext.setServletConfig(config);
pageContext.setServletRequest(request);
pageContext.setServletResponse(response);
filterConfig.setupServletContext(context);
setUpJspFactory();
}
private void setUpJspFactory()
{
if(jspFactory instanceof MockJspFactory)
{
((MockJspFactory)jspFactory).setPageContext(pageContext);
}
}
/**
* Sets the default JspFactory
by calling
* JspFactory.setDefaultFactory()
.
* @param jspFactory the JspFactory
*/
public void setDefaultJspFactory(JspFactory jspFactory)
{
JspFactory.setDefaultFactory(jspFactory);
this.jspFactory = jspFactory;
setUpJspFactory();
}
/**
* Refreshes the mock objects dependencies. May be called after setting request
* and response wrappers.
*/
public void refresh()
{
pageContext = new MockPageContext(config, wrappedRequest, wrappedResponse);
setUpJspFactory();
}
/**
* Creates the {@link com.mockrunner.mock.web.MockServletContext} using new
.
* This method can be overridden to return a subclass of {@link com.mockrunner.mock.web.MockServletContext}.
* @return the {@link com.mockrunner.mock.web.MockServletContext}
*/
public MockServletContext createMockServletContext()
{
return new MockServletContext();
}
/**
* Creates the {@link com.mockrunner.mock.web.MockServletConfig} using new
.
* This method can be overridden to return a subclass of {@link com.mockrunner.mock.web.MockServletConfig}.
* @return the {@link com.mockrunner.mock.web.MockServletConfig}
*/
public MockServletConfig createMockServletConfig()
{
return new MockServletConfig();
}
/**
* Creates the {@link com.mockrunner.mock.web.MockHttpServletResponse} using new
.
* This method can be overridden to return a subclass of {@link com.mockrunner.mock.web.MockHttpServletResponse}.
* @return the {@link com.mockrunner.mock.web.MockHttpServletResponse}
*/
public MockHttpServletResponse createMockResponse()
{
return new MockHttpServletResponse();
}
/**
* Creates the {@link com.mockrunner.mock.web.MockHttpServletRequest} using new
.
* This method can be overridden to return a subclass of {@link com.mockrunner.mock.web.MockHttpServletRequest}.
* @return the {@link com.mockrunner.mock.web.MockHttpServletRequest}
*/
public MockHttpServletRequest createMockRequest()
{
return new MockHttpServletRequest();
}
/**
* Creates the {@link com.mockrunner.mock.web.MockHttpSession} using new
.
* This method can be overridden to return a subclass of {@link com.mockrunner.mock.web.MockHttpSession}.
* @return the {@link com.mockrunner.mock.web.MockHttpSession}
*/
public MockHttpSession createMockSession()
{
return new MockHttpSession();
}
/**
* Creates the {@link com.mockrunner.mock.web.MockPageContext} using new
.
* This method can be overridden to return a subclass of {@link com.mockrunner.mock.web.MockPageContext}.
* @return the {@link com.mockrunner.mock.web.MockPageContext}
*/
public MockPageContext createMockPageContext()
{
return new MockPageContext();
}
/**
* Creates the {@link com.mockrunner.mock.web.MockFilterConfig} using new
.
* This method can be overridden to return a subclass of {@link com.mockrunner.mock.web.MockFilterConfig}.
* @return the {@link com.mockrunner.mock.web.MockFilterConfig}
*/
public MockFilterConfig createMockFilterConfig()
{
return new MockFilterConfig();
}
/**
* Creates the {@link com.mockrunner.mock.web.MockFilterChain} using new
.
* This method can be overridden to return a subclass of {@link com.mockrunner.mock.web.MockFilterChain}.
* @return the {@link com.mockrunner.mock.web.MockFilterChain}
*/
public MockFilterChain createMockFilterChain()
{
return new MockFilterChain();
}
/**
* Creates the {@link com.mockrunner.mock.web.MockJspFactory} using new
.
* This method can be overridden to return a subclass of {@link com.mockrunner.mock.web.MockJspFactory}.
* @return the {@link com.mockrunner.mock.web.MockJspFactory}
*/
public MockJspFactory createMockJspFactory()
{
return new MockJspFactory();
}
/**
* Returns the MockServletConfig
* @return the MockServletConfig
*/
public MockServletConfig getMockServletConfig()
{
return config;
}
/**
* Returns the {@link com.mockrunner.mock.web.MockServletContext}.
* @return the {@link com.mockrunner.mock.web.MockServletContext}
*/
public MockServletContext getMockServletContext()
{
return context;
}
/**
* Returns the {@link com.mockrunner.mock.web.MockHttpServletRequest}.
* @return the {@link com.mockrunner.mock.web.MockHttpServletRequest}
*/
public MockHttpServletRequest getMockRequest()
{
return request;
}
/**
* Returns the {@link com.mockrunner.mock.web.MockHttpServletResponse}.
* @return the {@link com.mockrunner.mock.web.MockHttpServletResponse}
*/
public MockHttpServletResponse getMockResponse()
{
return response;
}
/**
* Returns the wrapped HttpServletRequest
. If no
* wrapper is specified, this method returns the mock request itself.
* @return the wrapped HttpServletRequest
*/
public HttpServletRequest getWrappedRequest()
{
return wrappedRequest;
}
/**
* Returns the wrapped HttpServletResponse
. If no
* wrapper is specified, this method returns the mock response itself.
* @return the wrapped HttpServletRequest
*/
public HttpServletResponse getWrappedResponse()
{
return wrappedResponse;
}
/**
* Returns the {@link com.mockrunner.mock.web.MockHttpSession}.
* @return the {@link com.mockrunner.mock.web.MockHttpSession}
*/
public MockHttpSession getMockSession()
{
return session;
}
/**
* Returns the {@link com.mockrunner.mock.web.MockHttpSession}.
* @return the {@link com.mockrunner.mock.web.MockHttpSession}
* @deprecated use {@link #getMockSession}
*/
public MockHttpSession getSession()
{
return getMockSession();
}
/**
* Returns the {@link com.mockrunner.mock.web.MockPageContext}.
* @return the {@link com.mockrunner.mock.web.MockPageContext}
*/
public MockPageContext getMockPageContext()
{
return pageContext;
}
/**
* Returns the {@link com.mockrunner.mock.web.MockFilterConfig}.
* @return the {@link com.mockrunner.mock.web.MockFilterConfig}
*/
public MockFilterConfig getMockFilterConfig()
{
return filterConfig;
}
/**
* Returns the {@link com.mockrunner.mock.web.MockFilterChain}.
* @return the {@link com.mockrunner.mock.web.MockFilterChain}
*/
public MockFilterChain getMockFilterChain()
{
return filterChain;
}
/**
* Returns the {@link com.mockrunner.mock.web.MockJspFactory}.
* If the current JspFactory
is not an instance
* of {@link com.mockrunner.mock.web.MockJspFactory}, null
* will be returned.
* @return the {@link com.mockrunner.mock.web.MockJspFactory}
*/
public MockJspFactory getMockJspFactory()
{
if(jspFactory instanceof MockJspFactory)
{
return (MockJspFactory)jspFactory;
}
return null;
}
/**
* Returns the JspFactory
.
* @return the JspFactory
*/
public JspFactory getJspFactory()
{
return jspFactory;
}
/**
* Can be used to add a request wrapper. All the
* test modules are using the wrapped request returned by
* {@link #getWrappedRequest}. The method {@link #getMockRequest}
* returns the mock request without any wrapper.
* Usually the wrapper is of type javax.servlet.http.HttpServletRequestWrapper
.
* That's not absolutely necessary but the wrapper must define a constructor
* that takes a single javax.servlet.http.HttpServletRequest
argument
* and must implement javax.servlet.http.HttpServletRequest
.
* @param wrapper the wrapper class
*/
public void addRequestWrapper(Class wrapper)
{
try
{
Constructor constructor = wrapper.getConstructor(HttpServletRequest.class);
wrappedRequest = (HttpServletRequest)constructor.newInstance(wrappedRequest);
}
catch(Exception exc)
{
throw new NestedApplicationException(exc);
}
}
/**
* Can be used to add a request wrapper.
* All the test modules are using the wrapped request returned by
* {@link #getWrappedRequest}. The method {@link #getMockRequest}
* returns the mock request without any wrapper. Usually the wrapper is
* an instance of javax.servlet.http.HttpServletRequestWrapper
* and wraps the current request but that's not absolutely necessary.
* However, be careful if you want to add custom mock versions of
* javax.servlet.http.HttpServletRequest
.
* @param wrapper the request wrapper
*/
public void addRequestWrapper(HttpServletRequest wrapper)
{
wrappedRequest = wrapper;
}
/**
* Can be used to add a response wrapper. All the
* test modules are using the wrapped response returned by
* {@link #getWrappedResponse}. The method {@link #getMockResponse}
* returns the mock response without any wrapper.
* Usually the wrapper is of type javax.servlet.http.HttpServletResponseWrapper
.
* That's not absolutely necessary but the wrapper must define a constructor
* that takes a single javax.servlet.http.HttpServletResponse
argument
* and must implement javax.servlet.http.HttpServletResponse
.
* @param wrapper the wrapper class
*/
public void addResponseWrapper(Class wrapper)
{
try
{
Constructor constructor = wrapper.getConstructor(HttpServletResponse.class);
wrappedResponse = (HttpServletResponse)constructor.newInstance(wrappedResponse);
}
catch(Exception exc)
{
throw new NestedApplicationException(exc);
}
}
/**
* Can be used to add a response wrapper.
* All the test modules are using the wrapped response returned by
* {@link #getWrappedResponse}. The method {@link #getMockResponse}
* returns the mock response without any wrapper. Usually the wrapper is
* an instance of javax.servlet.http.HttpServletResponseWrapper
* and wraps the current response but that's not absolutely necessary.
* However, be careful if you want to add custom mock versions of
* javax.servlet.http.HttpServletResponse
.
* @param wrapper the wrapper
*/
public void addResponseWrapper(HttpServletResponse wrapper)
{
wrappedResponse = wrapper;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy