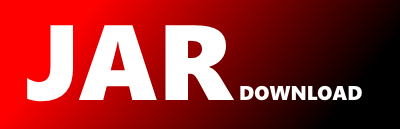
com.mokung.pomegranate.jackson.GlobalConfiguration Maven / Gradle / Ivy
package com.mokung.pomegranate.jackson;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.util.Date;
import java.util.LinkedHashMap;
import java.util.Map;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.annotation.JsonSerialize;
import com.fasterxml.jackson.databind.node.NullNode;
import com.fasterxml.jackson.databind.node.POJONode;
import com.mokung.pomegranate.jackson.handler.Getter;
import com.mokung.pomegranate.jackson.handler.getter.*;
/**
* 全局配置
*
* @author mokung
*/
public final class GlobalConfiguration {
/**
* 全局mapper
*/
private ObjectMapper objectMapper;
/**
* 值 获取配置
*/
private final Map, Getter>> getterConfig = new LinkedHashMap<>();
/**
* 获取 全局 ObjectMapper
*
* @return ObjectMapper
*/
public ObjectMapper getObjectMapper() {
return objectMapper;
}
/**
* 设置 全局 ObjectMapper
*
* @param objectMapper
* ObjectMapper
*/
@JsonSerialize
public synchronized void setObjectMapper(ObjectMapper objectMapper) {
if (objectMapper != null) {
this.objectMapper = objectMapper;
return;
}
throw new NullPointerException("ObjectMapper is Null");
}
@SuppressWarnings(value = {"unchecked"})
public Getter getter(Class clazz) {
return (Getter)getterConfig.get(clazz);
}
public Object getValue(JsonNode valueNode, ObjectMapper objectMapper, boolean cast) {
if (valueNode == null || valueNode instanceof NullNode) {
return null;
}
for (Map.Entry, Getter>> entry : getterConfig.entrySet()) {
Getter> getter = entry.getValue();
Object value = getter.getter(valueNode, objectMapper, cast);
if (value != null) {
return value;
}
}
if (valueNode instanceof POJONode) {
return ((POJONode)valueNode).getPojo();
}
throw new ClassCastException("not found class");
}
private GlobalConfiguration() {
setObjectMapper(new ObjectMapper());
getterConfig.put(Boolean.class, new BooleanGetter());
getterConfig.put(Byte.class, new ByteGetter());
getterConfig.put(Short.class, new ShortGetter());
getterConfig.put(Integer.class, new IntegerGetter());
getterConfig.put(Long.class, new LongGetter());
getterConfig.put(Float.class, new FloatGetter());
getterConfig.put(Double.class, new DoubleGetter());
getterConfig.put(BigInteger.class, new BigIntegerGetter());
getterConfig.put(BigDecimal.class, new BigDecimalGetter());
getterConfig.put(String.class, new StringGetter());
getterConfig.put(byte[].class, new BinaryGetter());
getterConfig.put(Date.class, new DateGetter());
getterConfig.put(JsonObject.class, new JsonObjectGetter());
getterConfig.put(JsonArray.class, new JsonArrayGetter());
}
private static class GlobalConfigurationInstance {
private static final GlobalConfiguration INSTANCE = new GlobalConfiguration();
}
public static GlobalConfiguration getInstance() {
return GlobalConfigurationInstance.INSTANCE;
}
@Override
protected Object clone() {
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy