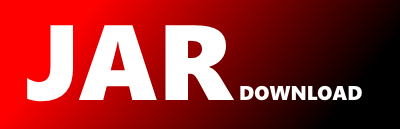
com.mooltiverse.oss.nyx.gradle.NyxExtension Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java Show documentation
Show all versions of java Show documentation
com.mooltiverse.oss.nyx:java:3.0.6 All the Nyx Java artifacts
The newest version!
/*
* Copyright 2020 Mooltiverse
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.mooltiverse.oss.nyx.gradle;
import java.io.File;
import javax.inject.Inject;
import org.gradle.api.Action;
import org.gradle.api.NamedDomainObjectContainer;
import org.gradle.api.Project;
import org.gradle.api.initialization.Settings;
import org.gradle.api.model.ObjectFactory;
import org.gradle.api.provider.ListProperty;
import org.gradle.api.provider.MapProperty;
import org.gradle.api.provider.Property;
import org.gradle.api.provider.Provider;
import com.mooltiverse.oss.nyx.entities.Defaults;
/**
* The plugin configuration object. This object is responsible for reading the {@code nyx {...} } configuration block that users
* define within the {@code build.gradle} script.
*
* See Modeling DSL-like APIs
* for more o developing extension.
*
* See Developing Custom Gradle Types,
* Developing Custom Gradle Plugins,
* Implementing Gradle plugins and
* Lazy Configuration
* for an introduction on custom Gradle types development.
*
* NOTES ON NESTED OBJECTS:
* In order to support DSL (the curly braces syntax) for nested objects we also need to support one additional method for each nested object
* to accept and configure the closure. Take the {@code commitMessageConventions} for example. According to the API docs, defining the
* field and the getter method as as:
*
* private CommitMessageConventions commitMessageConventions = getObjectfactory().newInstance(CommitMessageConventions.class);
*
* public CommitMessageConventions getCommitMessageConventions() {
* return commitMessageConventions;
* }
*
* should be enough but it's not, in fact at runtime, when using the curly braces syntax to define that property like:
*
* nyx {
* commitMessageConventions {
* enabled = ['conventionalCommits']
* }
* }
*
* throws an exception like:
*
* org.gradle.api.GradleScriptException: A problem occurred evaluating root project...
* ...
* Caused by: groovy.lang.MissingMethodException: No signature of method: build_araz4us455w31lfualzy83h1f.nyx() is applicable for argument types: (build_araz4us455w31lfualzy83h1f$_run_closure1) values: [build_araz4us455w31lfualzy83h1f$_run_closure1@22b6a7c8]
* Possible solutions: any(), any(groovy.lang.Closure), run(), run(), sync(org.gradle.api.Action), uri(java.lang.Object)
* ...
*
* So thanks to posts like this
* (which points to this example here),
* this and
* this
* it turns out there must be another method to accept the closure. I don't know Groovy so I'm not sure what it's needed for but the
* additional method can be in one of the two following variants (still using the {@code commitMessageConventions} example):
*
* public void commitMessageConventions(groovy.lang.Closure closure) {
* closure.setResolveStrategy(groovy.lang.Closure.DELEGATE_FIRST);
* closure.setDelegate(commitMessageConventions);
* closure.call();
* }
*
* or:
*
* public void commitMessageConventions(org.gradle.api.Action<? super CommitMessageConventions> configuration) {
* configuration.execute(commitMessageConventions);
* }
*
* I opted for the second one, as it's a little more comprehensible, although I don't know exactly what happens under the hood.
*
* @see org.gradle.api.plugins.ExtensionAware
* @see org.gradle.api.plugins.ExtensionContainer
*/
public abstract class NyxExtension {
/**
* The name of the extension object. This is the name of the configuration block inside Gradle scripts.
*/
public static final String NAME = "nyx";
/**
* The 'bump' property.
*/
private final Property bump = getObjectfactory().property(String.class);
/**
* The nested 'changelog' block.
*/
private final ChangelogConfiguration changelog = getObjectfactory().newInstance(ChangelogConfiguration.class);
/**
* The nested 'commitMessageConventions' block.
*/
private final CommitMessageConventions commitMessageConventions = getObjectfactory().newInstance(CommitMessageConventions.class);
/**
* The 'configurationFile' property.
*/
private final Property configurationFile = getObjectfactory().property(String.class);
/**
* The 'directory' property.
* Default is taken from the Gradle project directory but the method to retrieve that is
* based on where this object is applied (see the {@link #create(Project)} and
* {@link #create(Settings)} methods).
*
* This property uses the {@link Property#convention(Provider)} to define the default value so
* when users are good with the default value they don't need to define it in the build script.
*
* @see #create(Project)
* @see #create(Settings)
*/
private final Property directory = getObjectfactory().property(File.class);
/**
* The 'dryRun' property.
*/
private final Property dryRun = getObjectfactory().property(Boolean.class);
/**
* The nested 'git' block.
*/
private final GitConfiguration git = getObjectfactory().newInstance(GitConfiguration.class);
/**
* The 'initialVersion' property.
*/
private final Property initialVersion = getObjectfactory().property(String.class);
/**
* The 'preset' property.
*/
private final Property preset = getObjectfactory().property(String.class);
/**
* The nested 'releaseAssets' block.
*
* @see AssetConfiguration
*/
private NamedDomainObjectContainer releaseAssets = getObjectfactory().domainObjectContainer(AssetConfiguration.class);
/**
* The 'releaseLenient' property.
*/
private final Property releaseLenient = getObjectfactory().property(Boolean.class);
/**
* The 'releasePrefix' property.
*/
private final Property releasePrefix = getObjectfactory().property(String.class);
/**
* The nested 'releaseTypes' block.
*/
private final ReleaseTypes releaseTypes = getObjectfactory().newInstance(ReleaseTypes.class);
/**
* The 'resume' property.
*/
private final Property resume = getObjectfactory().property(Boolean.class);
/**
* The 'scheme' property.
*/
private final Property scheme = getObjectfactory().property(String.class);
/**
* The nested 'services' block.
*
* @see ServiceConfiguration
*/
private NamedDomainObjectContainer services = getObjectfactory().domainObjectContainer(ServiceConfiguration.class);
/**
* The 'sharedConfigurationFile' property.
*/
private final Property sharedConfigurationFile = getObjectfactory().property(String.class);
/**
* The 'summary' property.
*/
private final Property summary = getObjectfactory().property(Boolean.class);
/**
* The 'summaryFile' property.
*/
private final Property summaryFile = getObjectfactory().property(String.class);
/**
* The 'stateFile' property.
*/
private final Property stateFile = getObjectfactory().property(String.class);
/**
* The nested 'substitutions' block.
*/
private final Substitutions substitutions = getObjectfactory().newInstance(Substitutions.class);
/**
* The 'verbosity' property.
*
* Please note that the verbosity option is actually ignored in this plugin implementation and the backing Nyx implementation
* as it's controlled by Gradle.
*/
private final Property verbosity = getObjectfactory().property(String.class);
/**
* Returns an object factory instance.
*
* The instance is injected by Gradle as soon as this getter method is invoked.
*
* Using property injection
* instead of constructor injection
* has a few advantages: it allows Gradle to refer injecting the object until it's required and is safer for backward
* compatibility (older versions can be supported).
*
* @return the object factory instance
*/
@Inject
protected abstract ObjectFactory getObjectfactory();
/**
* Creates the extension into the given project.
*
* @param project the project to create the extension into
*
* @return the extension instance, within the given project
*/
public static NyxExtension create(Project project) {
NyxExtension extension = project.getExtensions().create(NyxExtension.NAME, NyxExtension.class);
extension.directory.convention(project.getLayout().getProjectDirectory().getAsFile());
return extension;
}
/**
* Creates the extension into the given settings.
*
* @param settings the settings to create the extension into
*
* @return the extension instance, within the given settings
*/
public static NyxExtension create(Settings settings) {
NyxExtension extension = settings.getExtensions().create(NyxExtension.NAME, NyxExtension.class);
extension.directory.convention(settings.getRootDir());
return extension;
}
/**
* Returns the name of the version identifier to bump. When this is set by the user it overrides
* the inference performed by Nyx.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the name of the version identifier to bump
*/
public Property getBump() {
return bump;
}
/**
* Returns the object mapping the {@code changelog} block.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the object mapping the {@code changelog} block
*/
public ChangelogConfiguration getChangelog() {
return changelog;
}
/**
* Accepts the DSL configuration for the {@code changelog} block, needed for defining
* the block using the curly braces syntax in Gradle build scripts.
* See the documentation on top of this class for more.
*
* @param configurationAction the configuration action for the {@code changelog} block
*/
public void changelog(Action super ChangelogConfiguration> configurationAction) {
configurationAction.execute(changelog);
}
/**
* Returns the object mapping the {@code commitMessageConventions} block.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the object mapping the {@code commitMessageConventions} block
*/
public CommitMessageConventions getCommitMessageConventions() {
return commitMessageConventions;
}
/**
* Accepts the DSL configuration for the {@code commitMessageConventions} block, needed for defining
* the block using the curly braces syntax in Gradle build scripts.
* See the documentation on top of this class for more.
*
* @param configurationAction the configuration action for the {@code commitMessageConventions} block
*/
public void commitMessageConventions(Action super CommitMessageConventions> configurationAction) {
configurationAction.execute(commitMessageConventions);
}
/**
* Returns the custom configuration file to use.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the custom configuration file to use
*
* @see Defaults#SHARED_CONFIGURATION_FILE
*/
public Property getConfigurationFile() {
return configurationFile;
}
/**
* Returns the directory to use as the base repository location.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the directory to use as the base repository location
*/
public Property getDirectory() {
return directory;
}
/**
* Returns the flag that, when {@code true}, prevents Nyx from applying any change to the repository or any
* other resource.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the flag that, when {@code true}, prevents Nyx from applying any change to the repository or any
* other resource
*
* @see Defaults#DRY_RUN
*/
public Property getDryRun() {
return dryRun;
}
/**
* Returns the object mapping the {@code git} block.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the object mapping the {@code git} block
*/
public GitConfiguration getGit() {
return git;
}
/**
* Accepts the DSL configuration for the {@code git} block, needed for defining
* the block using the curly braces syntax in Gradle build scripts.
* See the documentation on top of this class for more.
*
* @param configurationAction the configuration action for the {@code git} block
*/
public void git(Action super GitConfiguration> configurationAction) {
configurationAction.execute(git);
}
/**
* Returns the initial version to use when no past version can be inferred from the commit history.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the flag that, when {@code true}, prevents Nyx from applying any change to the repository or any
* other resource
*
* @see Defaults#DRY_RUN
*/
public Property getInitialVersion() {
return initialVersion;
}
/**
* Returns the selected preset configuration name.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the selected preset configuration name.
*
* @see Defaults#PRESET
*/
public Property getPreset() {
return preset;
}
/**
* Returns the object mapping the {@code releaseAssets} block.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the object mapping the {@code releaseAssets} block
*/
public NamedDomainObjectContainer getReleaseAssets() {
return releaseAssets;
}
/**
* Accepts the DSL configuration for the {@code releaseAssets} block, needed for defining
* the block using the curly braces syntax in Gradle build scripts.
* See the documentation on top of this class for more.
*
* @param configurationAction the configuration action for the {@code releaseAssets} block
*/
public void releaseAssets(Action super NamedDomainObjectContainer> configurationAction) {
configurationAction.execute(releaseAssets);
}
/**
* Returns the flag that, when {@code true}, lets Nyx interpret release names with whatever prefix.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the flag that, when {@code true}, lets Nyx interpret release names with whatever prefix
*
* @see Defaults#RELEASE_LENIENT
*/
public Property getReleaseLenient() {
return releaseLenient;
}
/**
* Returns the prefix used to generate release names.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the prefix used to generate release names
*
* @see Defaults#RELEASE_PREFIX
*/
public Property getReleasePrefix() {
return releasePrefix;
}
/**
* Returns the object mapping the {@code releaseTypes} block.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the object mapping the {@code releaseTypes} block
*/
public ReleaseTypes getReleaseTypes() {
return releaseTypes;
}
/**
* Accepts the DSL configuration for the {@code releaseTypes} block, needed for defining
* the block using the curly braces syntax in Gradle build scripts.
* See the documentation on top of this class for more.
*
* @param configurationAction the configuration action for the {@code releaseTypes} block
*/
public void releaseTypes(Action super ReleaseTypes> configurationAction) {
configurationAction.execute(releaseTypes);
}
/**
* Returns the flag that, when {@code true}, loads a previously saved state file (if any) to resume execution
* from there.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the flag that, when {@code true}, prevents Nyx from applying any change to the repository or any
* other resource
*
* @see Defaults#DRY_RUN
*/
public Property getResume() {
return resume;
}
/**
* Returns the versioning scheme to use.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the versioning scheme to use
*
* @see Defaults#SCHEME
*/
public Property getScheme() {
return scheme;
}
/**
* Returns the object mapping the {@code services} block.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the object mapping the {@code services} block
*/
public NamedDomainObjectContainer getServices() {
return services;
}
/**
* Accepts the DSL configuration for the {@code services} block, needed for defining
* the block using the curly braces syntax in Gradle build scripts.
* See the documentation on top of this class for more.
*
* @param configurationAction the configuration action for the {@code services} block
*/
public void services(Action super NamedDomainObjectContainer> configurationAction) {
configurationAction.execute(services);
}
/**
* Returns the custom shared configuration file to use.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the custom shared configuration file to use
*
* @see Defaults#SHARED_CONFIGURATION_FILE
*/
public Property getSharedConfigurationFile() {
return sharedConfigurationFile;
}
/**
* Returns the flag that, when {@code true}, prints a state summary to the console.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the flag that, when {@code true}, prevents Nyx from applying any change to the repository or any
* other resource
*
* @see Defaults#DRY_RUN
*/
public Property getSummary() {
return summary;
}
/**
* Returns the optional path where to save the summary file.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the directory to use as the base repository location
*/
public Property getSummaryFile() {
return summaryFile;
}
/**
* Returns the optional path where to save the state file.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the directory to use as the base repository location
*/
public Property getStateFile() {
return stateFile;
}
/**
* Returns the object mapping the {@code substitutions} block.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the object mapping the {@code substitutions} block
*/
public Substitutions getSubstitutions() {
return substitutions;
}
/**
* Accepts the DSL configuration for the {@code substitutions} block, needed for defining
* the block using the curly braces syntax in Gradle build scripts.
* See the documentation on top of this class for more.
*
* @param configurationAction the configuration action for the {@code substitutions} block
*/
public void substitutions(Action super Substitutions> configurationAction) {
configurationAction.execute(substitutions);
}
/**
* Returns the logging verbosity.
*
* Please note that the verbosity option is actually ignored in this plugin implementation and the backing Nyx implementation
* as it's controlled by Gradle.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the logging verbosity
*/
public Property getVerbosity() {
return verbosity;
}
/**
* The class to model the 'changelog' block within the extension.
*/
public abstract static class ChangelogConfiguration {
/**
* The flag instructing if and when to append contents to the existing changelog file.
*/
private final Property append = getObjectfactory().property(String.class);
/**
* The path to the destination file.
*/
private final Property path = getObjectfactory().property(String.class);
/**
* The path to the optional template file.
*/
private final Property template = getObjectfactory().property(String.class);
/**
* The nested 'sections' block.
*/
private final MapProperty sections = getObjectfactory().mapProperty(String.class, String.class);
/**
* The nested 'substitutions' block.
*/
private final MapProperty substitutions = getObjectfactory().mapProperty(String.class, String.class);
/**
* Returns an object factory instance.
*
* The instance is injected by Gradle as soon as this getter method is invoked.
*
* Using property injection
* instead of constructor injection
* has a few advantages: it allows Gradle to refer injecting the object until it's required and is safer for backward
* compatibility (older versions can be supported).
*
* @return the object factory instance
*/
@Inject
protected abstract ObjectFactory getObjectfactory();
/**
* Returns the flag instructing if and when to append contents to the existing changelog file.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the flag instructing if and when to append contents to the existing changelog file.
*/
public Property getAppend() {
return append;
}
/**
* Returns the path to the destination file. When this is set by the user it overrides
* the inference performed by Nyx.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the path to the destination file.
*/
public Property getPath() {
return path;
}
/**
* Returns the path to the optional template file. When this is set by the user it overrides
* the inference performed by Nyx.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the path to the optional template file.
*/
public Property getTemplate() {
return template;
}
/**
* Returns the map of changelog sections.
*
* @return the map of changelog sections.
*/
public MapProperty getSections() {
return sections;
}
/**
* Accepts the DSL configuration for the {@code sections} block, needed for defining
* the block using the curly braces syntax in Gradle build scripts.
* See the documentation on top of this class for more.
*
* @param configurationAction the configuration action for the {@code sections} block
*/
public void sections(Action super MapProperty> configurationAction) {
configurationAction.execute(sections);
}
/**
* Returns the map of changelog substitutions.
*
* @return the map of changelog substitutions.
*/
public MapProperty getSubstitutions() {
return substitutions;
}
/**
* Accepts the DSL configuration for the {@code substitutions} block, needed for defining
* the block using the curly braces syntax in Gradle build scripts.
* See the documentation on top of this class for more.
*
* @param configurationAction the configuration action for the {@code substitutions} block
*/
public void substitutions(Action super MapProperty> configurationAction) {
configurationAction.execute(substitutions);
}
}
/**
* The class to model the 'commitMessageConventions' block within the extension.
*/
public abstract static class CommitMessageConventions {
/**
* The list of enabled convention names.
*/
private final ListProperty enabled = getObjectfactory().listProperty(String.class);
/**
* The nested 'items' block.
*
* @see CommitMessageConvention
*/
private NamedDomainObjectContainer items = getObjectfactory().domainObjectContainer(CommitMessageConvention.class);
/**
* Returns an object factory instance.
*
* The instance is injected by Gradle as soon as this getter method is invoked.
*
* Using property injection
* instead of constructor injection
* has a few advantages: it allows Gradle to refer injecting the object until it's required and is safer for backward
* compatibility (older versions can be supported).
*
* @return the object factory instance
*/
@Inject
protected abstract ObjectFactory getObjectfactory();
/**
* Returns list of enabled convention names.
*
* @return list of enabled convention names.
*/
public ListProperty getEnabled() {
return enabled;
}
/**
* Returns the map of commit message convention items.
*
* @return the map of commit message convention items.
*/
public NamedDomainObjectContainer getItems() {
return items;
}
/**
* Accepts the DSL configuration for the {@code items} block, needed for defining
* the block using the curly braces syntax in Gradle build scripts.
* See the documentation on top of this class for more.
*
* @param configurationAction the configuration action for the {@code items} block
*/
public void items(Action super NamedDomainObjectContainer> configurationAction) {
configurationAction.execute(items);
}
/**
* The class to model a single 'commitMessageConventions' item within the extension.
*/
public abstract static class CommitMessageConvention {
/**
* The convention name.
*/
private final String name;
/**
* The convention regular expression property.
*/
private final Property expression = getObjectfactory().property(String.class);
/**
* The nested 'bumpExpressions' block.
*
* @see CommitMessageConvention
*/
private final MapProperty bumpExpressions = getObjectfactory().mapProperty(String.class, String.class);
/**
* Returns an object factory instance.
*
* The instance is injected by Gradle as soon as this getter method is invoked.
*
* Using property injection
* instead of constructor injection
* has a few advantages: it allows Gradle to refer injecting the object until it's required and is safer for backward
* compatibility (older versions can be supported).
*
* @return the object factory instance
*/
@Inject
protected abstract ObjectFactory getObjectfactory();
/**
* Constructor.
*
* This constructor is required as per the {@link NamedDomainObjectContainer} specification.
*
* @param name the convention name
*/
public CommitMessageConvention(String name) {
super();
this.name = name;
}
/**
* Returns the name read-only mandatory property.
*
* @return the name read-only mandatory property.
*/
public String getName() {
return name;
}
/**
* Returns the regular expression to infer fields from a commit message. When this is set by the user it overrides
* the inference performed by Nyx.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the regular expression to infer fields from a commit message
*/
public Property getExpression() {
return expression;
}
/**
* Returns the map of bump expression items.
*
* @return the map of bump expression items.
*/
public MapProperty getBumpExpressions() {
return bumpExpressions;
}
/**
* Accepts the DSL configuration for the {@code bumpExpressions} block, needed for defining
* the block using the curly braces syntax in Gradle build scripts.
* See the documentation on top of this class for more.
*
* @param configurationAction the configuration action for the {@code bumpExpressions} block
*/
public void bumpExpressions(Action super MapProperty> configurationAction) {
configurationAction.execute(bumpExpressions);
}
}
}
/**
* The class to model the 'git' block within the extension.
*/
public abstract static class GitConfiguration {
/**
* The nested 'remotes' block.
*
* @see GitRemoteConfiguration
*/
private NamedDomainObjectContainer remotes = getObjectfactory().domainObjectContainer(GitRemoteConfiguration.class);
/**
* Returns an object factory instance.
*
* The instance is injected by Gradle as soon as this getter method is invoked.
*
* Using property injection
* instead of constructor injection
* has a few advantages: it allows Gradle to refer injecting the object until it's required and is safer for backward
* compatibility (older versions can be supported).
*
* @return the object factory instance
*/
@Inject
protected abstract ObjectFactory getObjectfactory();
/**
* Returns the map of remotes.
*
* @return the map of remotes.
*/
public NamedDomainObjectContainer getRemotes() {
return remotes;
}
/**
* Accepts the DSL configuration for the {@code remotes} block, needed for defining
* the block using the curly braces syntax in Gradle build scripts.
* See the documentation on top of this class for more.
*
* @param configurationAction the configuration action for the {@code items} block
*/
public void remotes(Action super NamedDomainObjectContainer> configurationAction) {
configurationAction.execute(remotes);
}
/**
* The class to model a single 'remotes' item within the extension.
*/
public abstract static class GitRemoteConfiguration {
/**
* The remote name.
*/
private final String name;
/**
* The remote authentication method property.
*/
private final Property authenticationMethod = getObjectfactory().property(String.class);
/**
* The remote password property.
*/
private final Property password = getObjectfactory().property(String.class);
/**
* The remote user property.
*/
private final Property user = getObjectfactory().property(String.class);
/**
* The remote private key property.
*/
private final Property privateKey = getObjectfactory().property(String.class);
/**
* The remote passphrase for the private key property.
*/
private final Property passphrase = getObjectfactory().property(String.class);
/**
* Returns an object factory instance.
*
* The instance is injected by Gradle as soon as this getter method is invoked.
*
* Using property injection
* instead of constructor injection
* has a few advantages: it allows Gradle to refer injecting the object until it's required and is safer for backward
* compatibility (older versions can be supported).
*
* @return the object factory instance
*/
@Inject
protected abstract ObjectFactory getObjectfactory();
/**
* Constructor.
*
* This constructor is required as per the {@link NamedDomainObjectContainer} specification.
*
* @param name the remote name
*/
public GitRemoteConfiguration(String name) {
super();
this.name = name;
}
/**
* Returns the name read-only mandatory property.
*
* @return the name read-only mandatory property.
*/
public String getName() {
return name;
}
/**
* Returns the remote authentication method. When this is set by the user it overrides
* the inference performed by Nyx.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the remote authentication method
*/
public Property getAuthenticationMethod() {
return authenticationMethod;
}
/**
* Returns the remote password. When this is set by the user it overrides
* the inference performed by Nyx.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the remote password
*/
public Property getPassword() {
return password;
}
/**
* Returns the remote user. When this is set by the user it overrides
* the inference performed by Nyx.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the remote user
*/
public Property getUser() {
return user;
}
/**
* Returns the remote private key. When this is set by the user it overrides
* the inference performed by Nyx.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the remote private key
*/
public Property getPrivateKey() {
return privateKey;
}
/**
* Returns the remote passphrase for the private key. When this is set by the user it overrides
* the inference performed by Nyx.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the remote passphrase for the private key
*/
public Property getPassphrase() {
return passphrase;
}
}
}
/**
* The class to model a single 'releaseAssets' item within the extension.
*/
public abstract static class AssetConfiguration {
/**
* The asset name.
*/
private final String name;
/**
* The asset file name property.
*/
private final Property fileName = getObjectfactory().property(String.class);
/**
* The asset (short) description (or label) property.
*/
private final Property description = getObjectfactory().property(String.class);
/**
* The asset path property (local file or URL).
*/
private final Property path = getObjectfactory().property(String.class);
/**
* The asset MIME type property.
*/
private final Property type = getObjectfactory().property(String.class);
/**
* Returns an object factory instance.
*
* The instance is injected by Gradle as soon as this getter method is invoked.
*
* Using property injection
* instead of constructor injection
* has a few advantages: it allows Gradle to refer injecting the object until it's required and is safer for backward
* compatibility (older versions can be supported).
*
* @return the object factory instance
*/
@Inject
protected abstract ObjectFactory getObjectfactory();
/**
* Constructor.
*
* This constructor is required as per the {@link NamedDomainObjectContainer} specification.
*
* @param name the asset name (the map key)
*/
public AssetConfiguration(String name) {
super();
this.name = name;
}
/**
* Returns the asset name read-only mandatory property.
*
* @return the asset name read-only mandatory property.
*/
public String getName() {
return name;
}
/**
* Returns the asset file name.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the asset name (usually the file name)
*/
public Property getFileName() {
return fileName;
}
/**
* Returns the asset (short) description (or label) property.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the asset (short) description (or label)
*/
public Property getDescription() {
return description;
}
/**
* Returns the asset path (local file or URL).
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the asset path (local file or URL)
*/
public Property getPath() {
return path;
}
/**
* Returns the asset MIME type.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the asset MIME type
*/
public Property getType() {
return type;
}
}
/**
* The class to model the 'releaseTypes' block within the extension.
*/
public abstract static class ReleaseTypes {
/**
* The list of enabled release type names.
*/
private final ListProperty enabled = getObjectfactory().listProperty(String.class);
/**
* The list of publication service names.
*/
private final ListProperty publicationServices = getObjectfactory().listProperty(String.class);
/**
* The list of remote repository names.
*/
private final ListProperty remoteRepositories = getObjectfactory().listProperty(String.class);
/**
* The nested 'items' block.
*
* @see ReleaseType
*/
private NamedDomainObjectContainer items = getObjectfactory().domainObjectContainer(ReleaseType.class);
/**
* Returns an object factory instance.
*
* The instance is injected by Gradle as soon as this getter method is invoked.
*
* Using property injection
* instead of constructor injection
* has a few advantages: it allows Gradle to refer injecting the object until it's required and is safer for backward
* compatibility (older versions can be supported).
*
* @return the object factory instance
*/
@Inject
protected abstract ObjectFactory getObjectfactory();
/**
* Returns list of enabled convention names.
*
* @return list of enabled convention names.
*/
public ListProperty getEnabled() {
return enabled;
}
/**
* Returns list of publication service names.
*
* @return list of publication service names.
*/
public ListProperty getPublicationServices() {
return publicationServices;
}
/**
* Returns list of remote repository names.
*
* @return list of remote repository names.
*/
public ListProperty getRemoteRepositories() {
return remoteRepositories;
}
/**
* Returns the map of release type items.
*
* @return the map of release type items.
*/
public NamedDomainObjectContainer getItems() {
return items;
}
/**
* Accepts the DSL configuration for the {@code items} block, needed for defining
* the block using the curly braces syntax in Gradle build scripts.
* See the documentation on top of this class for more.
*
* @param configurationAction the configuration action for the {@code items} block
*/
public void items(Action super NamedDomainObjectContainer> configurationAction) {
configurationAction.execute(items);
}
/**
* The class to model a single 'releaseTypes' item within the extension.
*/
public abstract static class ReleaseType {
/**
* The release type name.
*/
private final String name;
/**
* The list of enabled assets for the release type.
*/
// We need to let Gradle parse this as a string rather than a list of strings.
// As documented at https://github.com/mooltiverse/nyx/issues/110, ListProperty.isPresent() never returns 'false', even when the
// property hasn't been defined by the user, so we are unable to distinguish when it was defined as empty or not defined at all.
// Within Nyx we have different semantics for the two cases o we need to read it as a simple string of comma separated items and
// then (in the ConfigurationLayer class) split them to a list, if the string property was defined.
private final Property assets = getObjectfactory().property(String.class);
/**
* The flag indicating whether or not the 'collapsed' versioning (pre-release style) must be used.
*/
private final Property collapseVersions = getObjectfactory().property(Boolean.class);
/**
* The optional qualifier or the template to render the qualifier to use for the pre-release identifier when versions are collapsed.
*/
private final Property collapsedVersionQualifier = getObjectfactory().property(String.class);
/**
* The optional template to render as a regular expression used for the release description.
*/
private final Property description = getObjectfactory().property(String.class);
/**
* The optional template to render as a regular expression used to match tags from the commit history.
*/
private final Property filterTags = getObjectfactory().property(String.class);
/**
* The optional flag or the template to render indicating whether or not a new commit must be generated in case new artifacts are generated.
*/
private final Property gitCommit = getObjectfactory().property(String.class);
/**
* The optional string or the template to render to use as the commit message if a commit has to be made.
*/
private final Property gitCommitMessage = getObjectfactory().property(String.class);
/**
* The optional flag or the template to render indicating whether or not a new commit must be generated and pushed in case new artifacts are generated.
*/
private final Property gitPush = getObjectfactory().property(String.class);
/**
* The optional flag or the template to enable/disable the Git push operation.
*/
private final Property gitPushForce = getObjectfactory().property(String.class);
/**
* The optional flag or the template to render indicating whether or not a new tag must be generated.
*/
private final Property gitTag = getObjectfactory().property(String.class);
/**
* The optional flag or the template to enable/disable the Git tag operation.
*/
private final Property gitTagForce = getObjectfactory().property(String.class);
/**
* The optional string or the template to render to use as the tag message if a tag has to be made.
*/
private final Property gitTagMessage = getObjectfactory().property(String.class);
/**
* The list of templates to use as tag names when tagging a commit.
*/
private final ListProperty gitTagNames = getObjectfactory().listProperty(String.class);
/**
* The nested 'identifiers' block.
*/
// TODO: remove this member if and when https://github.com/mooltiverse/nyx/issues/77 is solved
private NamedDomainObjectContainer identifiers = getObjectfactory().domainObjectContainer(Identifier.class);
/**
* The nested 'identifiers' block.
*/
// TODO: uncomment this member if and when https://github.com/mooltiverse/nyx/issues/77 is solved
//private final ListProperty identifiers = getObjectfactory().listProperty(Identifier.class);
/**
* The optional template to render as a regular expression used to match branch names.
*/
private final Property matchBranches = getObjectfactory().property(String.class);
/**
* The identifier of a specific workspace status to be matched.
*/
private final Property matchWorkspaceStatus = getObjectfactory().property(String.class);
/**
* The optional flag or the template to render indicating whether or not releases must be published.
*/
private final Property publish = getObjectfactory().property(String.class);
/**
* The the optional template to set the draft flag of releases published to remote services.
*/
private final Property publishDraft = getObjectfactory().property(String.class);
/**
* The optional template to set the pre-release flag of releases published to remote services.
*/
private final Property publishPreRelease = getObjectfactory().property(String.class);
/**
* The optional template to set the name of releases published to remote services.
*/
private final Property releaseName = getObjectfactory().property(String.class);
/**
* The optional template to render as a regular expression used to constrain versions issued by this release type.
*/
private final Property versionRange = getObjectfactory().property(String.class);
/**
* The optional flag telling if the version range must be inferred from the branch name.
*/
private final Property versionRangeFromBranchName = getObjectfactory().property(Boolean.class);
/**
* The nested 'matchEnvironmentVariables' block.
*/
private final MapProperty matchEnvironmentVariables = getObjectfactory().mapProperty(String.class, String.class);
/**
* Returns an object factory instance.
*
* The instance is injected by Gradle as soon as this getter method is invoked.
*
* Using property injection
* instead of constructor injection
* has a few advantages: it allows Gradle to refer injecting the object until it's required and is safer for backward
* compatibility (older versions can be supported).
*
* @return the object factory instance
*/
@Inject
protected abstract ObjectFactory getObjectfactory();
/**
* Constructor.
*
* This constructor is required as per the {@link NamedDomainObjectContainer} specification.
*
* @param name the release type name
*/
public ReleaseType(String name) {
super();
this.name = name;
}
/**
* Returns the name read-only mandatory property.
*
* @return the name read-only mandatory property.
*/
public String getName() {
return name;
}
/**
* Returns a string with the comma separatedlist of selected asset names to publish with the release.
* When {@code null} all assets configured globally (if any) must be published, otherwise only the assets
* in this list must be published for this release type.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the list of selected asset names to publish with the release.
*/
public Property getAssets() {
return assets;
}
/**
* Returns the flag indicating whether or not the 'collapsed' versioning (pre-release style) must be used.
* When this is set by the user it overrides the inference performed by Nyx.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the flag indicating whether or not the 'collapsed' versioning (pre-release style) must be used.
*/
public Property getCollapseVersions() {
return collapseVersions;
}
/**
* Returns the optional qualifier or the template to render the qualifier to use for the pre-release
* identifier when versions are collapsed. When this is set by the user it overrides
* the inference performed by Nyx.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the optional qualifier or the template to render the qualifier to use for the pre-release
* identifier when versions are collapsed
*/
public Property getCollapsedVersionQualifier() {
return collapsedVersionQualifier;
}
/**
* Returns the optional flag or the template to render indicating the release description.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the optional flag or the template to render indicating the release description.
*/
public Property getDescription() {
return description;
}
/**
* Returns the optional template to render as a regular expression used to match tags
* from the commit history. When this is set by the user it overrides
* the inference performed by Nyx.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the optional template to render as a regular expression used to match tags
* from the commit history.
*/
public Property getFilterTags() {
return filterTags;
}
/**
* Returns the optional flag or the template to render indicating whether or not a new commit must be generated in case new artifacts are generated.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the optional flag or the template to render indicating whether or not a new commit must be generated in case new artifacts are generated.
*/
public Property getGitCommit() {
return gitCommit;
}
/**
* Returns the optional string or the template to render to use as the commit message if a commit has to be made.
* When this is set by the user it overrides the inference performed by Nyx.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the optional string or the template to render to use as the commit message if a commit has to be made.
*/
public Property getGitCommitMessage() {
return gitCommitMessage;
}
/**
* Returns the optional flag or the template to render indicating whether or not a new commit must be generated and pushed in case new artifacts are generated.
* When this is set by the user it overrides the inference performed by Nyx.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the optional flag or the template to render indicating whether or not a new commit must be generated and pushed in case new artifacts are generated.
*/
public Property getGitPush() {
return gitPush;
}
/**
* Returns optional flag or the template to enable/disable the Git push operation.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the optional flag or the template to enable/disable the Git push operation.
*/
public Property getGitPushForce() {
return gitPushForce;
}
/**
* Returns the optional flag or the template to render indicating whether or not a new tag must be generated.
* When this is set by the user it overrides the inference performed by Nyx.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the optional flag or the template to render indicating whether or not a new tag must be generated.
*/
public Property getGitTag() {
return gitTag;
}
/**
* Returns optional flag or the template to enable/disable the Git tag operation.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the optional flag or the template to enable/disable the Git tag operation.
*/
public Property getGitTagForce() {
return gitTagForce;
}
/**
* Returns the optional string or the template to render to use as the tag message if a tag has to be made.
* When this is set by the user it overrides the inference performed by Nyx.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the optional string or the template to render to use as the tag message if a tag has to be made.
*/
public Property getGitTagMessage() {
return gitTagMessage;
}
/**
* Returns the list of templates to use as tag names when tagging a commit.
*
* @return the list of templates to use as tag names when tagging a commit.
*/
public ListProperty getGitTagNames() {
return gitTagNames;
}
/**
* Returns the map of identifier items.
*
* @return the map of identifier items.
*/
public NamedDomainObjectContainer getIdentifiers() {
// TODO: remove this method if and when https://github.com/mooltiverse/nyx/issues/77 is solved
return identifiers;
}
/**
* Accepts the DSL configuration for the {@code identifiers} block, needed for defining
* the block using the curly braces syntax in Gradle build scripts.
* See the documentation on top of this class for more.
*
* @param configurationAction the configuration action for the {@code identifiers} block
*/
public void identifiers(Action super NamedDomainObjectContainer> configurationAction) {
// TODO: remove this method if and when https://github.com/mooltiverse/nyx/issues/77 is solved
configurationAction.execute(identifiers);
}
/**
* Returns the object mapping the {@code identifiers} block.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the object mapping the {@code identifiers} block
*/
/*public ListProperty getIdentifiers() {
// TODO: uncomment this method if and when https://github.com/mooltiverse/nyx/issues/77 is solved
return identifiers;
}*/
/**
* Accepts the DSL configuration for the {@code identifiers} block, needed for defining
* the block using the curly braces syntax in Gradle build scripts.
* See the documentation on top of this class for more.
*
* @param configuration the configuration object for the {@code identifiers} block
*/
/*public void identifiers(Action super ListProperty> configurationAction) {
// TODO: uncomment this method if and when https://github.com/mooltiverse/nyx/issues/77 is solved
configurationAction.execute(identifiers);
}*/
/**
* Returns the optional template to render as a regular expression used to match branch names.
* When this is set by the user it overrides the inference performed by Nyx.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the optional template to render as a regular expression used to match branch names.
*/
public Property getMatchBranches() {
return matchBranches;
}
/**
* Returns the map of match environment variables names and regular expressions items.
*
* @return the map of match environment variables names and regular expressions items.
*/
public MapProperty getMatchEnvironmentVariables() {
return matchEnvironmentVariables;
}
/**
* Accepts the DSL configuration for the {@code matchEnvironmentVariables} block, needed for defining
* the block using the curly braces syntax in Gradle build scripts.
* See the documentation on top of this class for more.
*
* @param configurationAction the configuration action for the {@code matchEnvironmentVariables} block
*/
public void matchEnvironmentVariables(Action super MapProperty> configurationAction) {
configurationAction.execute(matchEnvironmentVariables);
}
/**
* Returns the identifier of a specific workspace status to be matched.
* When this is set by the user it overrides the inference performed by Nyx.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the identifier of a specific workspace status to be matched.
*/
public Property getMatchWorkspaceStatus() {
return matchWorkspaceStatus;
}
/**
* Returns the optional flag or the template to render indicating whether or not releases must be published.
* When this is set by the user it overrides the inference performed by Nyx.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the optional flag or the template to render indicating whether or not releases must be published.
*/
public Property getPublish() {
return publish;
}
/**
* Returns the optional template to set the draft flag of releases published to remote services.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the optional template to set the draft flag of releases published to remote services.
*/
public Property getPublishDraft() {
return publishDraft;
}
/**
* Returns the optional template to set the pre-release flag of releases published to remote services.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the optional template to set the pre-release flag of releases published to remote services.
*/
public Property getPublishPreRelease() {
return publishPreRelease;
}
/**
* Returns the optional template to set the name of releases published to remote services.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the optional template to set the name of releases published to remote services.
*/
public Property getReleaseName() {
return releaseName;
}
/**
* Returns the optional template to render as a regular expression used to constrain versions issued by this release type.
* When this is set by the user it overrides the inference performed by Nyx.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the optional template to render as a regular expression used to constrain versions issued by this release type.
*/
public Property getVersionRange() {
return versionRange;
}
/**
* Returns the optional flag telling if the version range must be inferred from the branch name.
* When this is set by the user it overrides the inference performed by Nyx.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the optional flag telling if the version range must be inferred from the branch name.
*/
public Property getVersionRangeFromBranchName() {
return versionRangeFromBranchName;
}
/**
* The class to model a single 'identifiers' item within the extension.
*/
public abstract static class Identifier {
/**
* The identifier name.
*/
// TODO: remove this member if and when https://github.com/mooltiverse/nyx/issues/77 is solved
private final String name;
/**
* The identifier position.
*/
private final Property position = getObjectfactory().property(String.class);
/**
* The identifier qualifier.
*/
private final Property qualifier = getObjectfactory().property(String.class);
/**
* The identifier value.
*/
private final Property value = getObjectfactory().property(String.class);
/**
* Returns an object factory instance.
*
* The instance is injected by Gradle as soon as this getter method is invoked.
*
* Using property injection
* instead of constructor injection
* has a few advantages: it allows Gradle to refer injecting the object until it's required and is safer for backward
* compatibility (older versions can be supported).
*
* @return the object factory instance
*/
@Inject
protected abstract ObjectFactory getObjectfactory();
/**
* Constructor.
*
* This constructor is required as per the {@link NamedDomainObjectContainer} specification.
*
* @param name the identifier name
*/
public Identifier(String name) {
super();
// TODO: remove this member if and when https://github.com/mooltiverse/nyx/issues/77 is solved
this.name = name;
}
/**
* Returns the name read-only mandatory property.
*
* @return the name read-only mandatory property.
*/
public String getName() {
return name;
}
/**
* Returns the identifier qualifier.
* When this is set by the user it overrides the inference performed by Nyx.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the identifier qualifier.
*/
public Property getPosition() {
return position;
}
/**
* Returns the identifier qualifier.
* When this is set by the user it overrides the inference performed by Nyx.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the identifier qualifier.
*/
public Property getQualifier() {
return qualifier;
}
/**
* Returns the identifier value.
* When this is set by the user it overrides the inference performed by Nyx.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the identifier value.
*/
public Property getValue() {
return value;
}
}
}
}
/**
* The class to model a single 'services' item within the extension.
*/
public abstract static class ServiceConfiguration {
/**
* The service name.
*/
private final String name;
/**
* The service type property.
*/
private final Property type = getObjectfactory().property(String.class);
/**
* The nested 'options' block.
*/
private final MapProperty options = getObjectfactory().mapProperty(String.class, String.class);
/**
* Returns an object factory instance.
*
* The instance is injected by Gradle as soon as this getter method is invoked.
*
* Using property injection
* instead of constructor injection
* has a few advantages: it allows Gradle to refer injecting the object until it's required and is safer for backward
* compatibility (older versions can be supported).
*
* @return the object factory instance
*/
@Inject
protected abstract ObjectFactory getObjectfactory();
/**
* Constructor.
*
* This constructor is required as per the {@link NamedDomainObjectContainer} specification.
*
* @param name the service name
*/
public ServiceConfiguration(String name) {
super();
this.name = name;
}
/**
* Returns the name read-only mandatory property.
*
* @return the name read-only mandatory property.
*/
public String getName() {
return name;
}
/**
* Returns the service type. When this is set by the user it overrides
* the inference performed by Nyx.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the service type
*/
public Property getType() {
return type;
}
/**
* Returns the map of service options.
*
* @return the map of service options.
*/
public MapProperty getOptions() {
return options;
}
/**
* Accepts the DSL configuration for the {@code options} block, needed for defining
* the block using the curly braces syntax in Gradle build scripts.
* See the documentation on top of this class for more.
*
* @param configurationAction the configuration action for the {@code options} block
*/
public void options(Action super MapProperty> configurationAction) {
configurationAction.execute(options);
}
}
/**
* The class to model the 'substitutions' block within the extension.
*/
public abstract static class Substitutions {
/**
* The list of enabled substitution names.
*/
private final ListProperty enabled = getObjectfactory().listProperty(String.class);
/**
* The nested 'items' block.
*
* @see Substitution
*/
private NamedDomainObjectContainer items = getObjectfactory().domainObjectContainer(Substitution.class);
/**
* Returns an object factory instance.
*
* The instance is injected by Gradle as soon as this getter method is invoked.
*
* Using property injection
* instead of constructor injection
* has a few advantages: it allows Gradle to refer injecting the object until it's required and is safer for backward
* compatibility (older versions can be supported).
*
* @return the object factory instance
*/
@Inject
protected abstract ObjectFactory getObjectfactory();
/**
* Returns list of enabled substitution names.
*
* @return list of enabled substitution names.
*/
public ListProperty getEnabled() {
return enabled;
}
/**
* Returns the map of substitution items.
*
* @return the map of substitution items.
*/
public NamedDomainObjectContainer getItems() {
return items;
}
/**
* Accepts the DSL configuration for the {@code items} block, needed for defining
* the block using the curly braces syntax in Gradle build scripts.
* See the documentation on top of this class for more.
*
* @param configurationAction the configuration action for the {@code items} block
*/
public void items(Action super NamedDomainObjectContainer> configurationAction) {
configurationAction.execute(items);
}
/**
* The class to model a single 'substitution' item within the extension.
*/
public abstract static class Substitution {
/**
* The substitution name.
*/
private final String name;
/**
* The substitution files property.
*/
private final Property files = getObjectfactory().property(String.class);
/**
* The substitution match property.
*/
private final Property match = getObjectfactory().property(String.class);
/**
* The substitution replace property.
*/
private final Property replace = getObjectfactory().property(String.class);
/**
* Returns an object factory instance.
*
* The instance is injected by Gradle as soon as this getter method is invoked.
*
* Using property injection
* instead of constructor injection
* has a few advantages: it allows Gradle to refer injecting the object until it's required and is safer for backward
* compatibility (older versions can be supported).
*
* @return the object factory instance
*/
@Inject
protected abstract ObjectFactory getObjectfactory();
/**
* Constructor.
*
* This constructor is required as per the {@link NamedDomainObjectContainer} specification.
*
* @param name the substitution name
*/
public Substitution(String name) {
super();
this.name = name;
}
/**
* Returns the name read-only mandatory property.
*
* @return the name read-only mandatory property.
*/
public String getName() {
return name;
}
/**
* Returns the glob expression to select the text files to replace the matched strings into.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the glob expression to select the text files to replace the matched strings into.
*/
public Property getFiles() {
return files;
}
/**
* Returns the regular expression used to match the text to be replaced replace in files.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the regular expression used to match the text to be replaced replace in files.
*/
public Property getMatch() {
return match;
}
/**
* Returns the template expression defining the text to use when replacing all matched tokens.
*
* We provide an implementation of this method instead of using the abstract definition as it's
* safer for old Gradle versions we support.
*
* @return the template expression defining the text to use when replacing all matched tokens.
*/
public Property getReplace() {
return replace;
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy