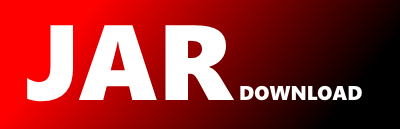
com.mooltiverse.oss.nyx.version.SemanticVersionBuildIdentifier Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java Show documentation
Show all versions of java Show documentation
com.mooltiverse.oss.nyx:java:3.0.6 All the Nyx Java artifacts
The newest version!
/*
* Copyright 2020 Mooltiverse
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.mooltiverse.oss.nyx.version;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.Objects;
/**
* The specialization of a Build version number as per Semantic Versioning 2.0.0.
*
* This identifier has a peculiar behavior as all parts are parsed as strings, even numeric ones, to preserve any
* leading zeroes.
*/
class SemanticVersionBuildIdentifier extends CompositeStringIdentifier {
/**
* Serial version UID to comply with {@link java.io.Serializable}
*/
private static final long serialVersionUID = 1L;
/**
* Builds the value identifier with the given values.
*
* @param children the children of this composite identifier. It can't be {@code null} or contain {@code null} values
*
* @throws NullPointerException if the given list of children is {@code null} or contains {@code null} values
* @throws IllegalArgumentException if the given list of children contains illegal values
*/
private SemanticVersionBuildIdentifier(List children) {
super(DEFAULT_SEPARATOR, children);
}
/**
* Returns an identifier instance representing the specified String value.
*
* @param multipleIdentifiers when {@code true} the given string is parsed as it (may) contain multiple
* identifiers, separated by the default separator, so this method may yield to multiple identifiers.
* When {@code false} the given string is expected to have a single identifier so if the given
* string has multiple identifiers an exception is thrown.
* @param s the string to parse
*
* @return the new identifier instance representing the given string.
*
* @throws NullPointerException if the given string is {@code null}
* @throws IllegalArgumentException if the given string contains illegal characters or there isn't any non
* {@code null} item
*/
static SemanticVersionBuildIdentifier valueOf(boolean multipleIdentifiers, String s) {
if (multipleIdentifiers)
return new SemanticVersionBuildIdentifier(Parser.toStringIdentifiers(s, DEFAULT_SEPARATOR));
else return new SemanticVersionBuildIdentifier(List.of(StringIdentifier.valueOf(s)));
}
/**
* Returns an identifier instance representing the specified String values.
*
* @param multipleIdentifiers when {@code true} the given string is parsed as it (may) contain multiple
* identifiers, separated by the default separator, so this method may yield to multiple identifiers.
* When {@code false} the given string is expected to have a single identifier so if the given
* string has multiple identifiers an exception is thrown.
* @param items the strings to parse
*
* @return the new identifier instance representing the given string.
*
* @throws NullPointerException if the given strings is {@code null}
* @throws IllegalArgumentException if the given string contains illegal characters or there isn't any non
* {@code null} item
*/
static SemanticVersionBuildIdentifier valueOf(boolean multipleIdentifiers, String... items) {
Objects.requireNonNull(items, "Can't build the list of identifiers from a null list");
if (items.length == 0)
throw new IllegalArgumentException("Can't build the list of identifiers from an empty list");
List allIdentifiers = new ArrayList();
for (String s: items)
if (multipleIdentifiers)
allIdentifiers.addAll(Parser.toStringIdentifiers(s, DEFAULT_SEPARATOR));
else allIdentifiers.add(StringIdentifier.valueOf(s));
return new SemanticVersionBuildIdentifier(allIdentifiers);
}
/**
* Returns {@code true} if an attribute with the given name is present, {@code false} otherwise.
*
* @param name the name of the attribute to look up. If {@code null} or empty {@code false} is returned
*
* @return {@code true} if an attribute with the given name is present, {@code false} otherwise.
*/
boolean hasAttribute(String name) {
if ((name == null) || (name.isBlank()))
return false;
return getValues().contains(name);
}
/**
* If an attribute with the given name is present, return the identifier after that, otherwise return {@code null}.
*
* @param name the name of the attribute to look up. If {@code null} or empty {@code null} is returned
*
* @return the attribute after the given name if such attribute is found and there is another attribute after it,
* otherwise {@code null}
*/
String getAttributeValue(String name) {
if ((name == null) || (name.isBlank()))
return null;
Iterator
© 2015 - 2024 Weber Informatics LLC | Privacy Policy