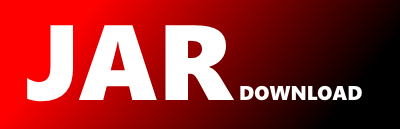
com.moparisthebest.mirror.convert.Convertable Maven / Gradle / Ivy
/*
* aptIn16 - Apt implementation with Java 6 annotation processors.
* Copyright (C) 2012 Travis Burtrum (moparisthebest)
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published y
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package com.moparisthebest.mirror.convert;
import java.util.*;
public abstract class Convertable implements ConvertableIface {
public abstract T convertToType(F from);
@Override
@SuppressWarnings({"unchecked"})
public E convertToType(F from, Class type) {
return (E) convertToType(from);
}
public List convert(Collection extends F> from, Class type) {
List ret = new ArrayList(from.size());
for (F fromF : from)
ret.add(convertToType(fromF, type));
return ret;
}
public Set convertToSet(Collection extends F> from, Class type) {
Set ret = new LinkedHashSet(from.size());
for (F fromF : from)
ret.add(convertToType(fromF, type));
return ret;
}
public List convert(Collection extends F> from) {
List ret = new ArrayList(from.size());
for (F fromF : from)
ret.add(convertToType(fromF));
return ret;
}
public Set convertToSet(Collection extends F> from) {
Set ret = new LinkedHashSet(from.size());
for (F fromF : from)
ret.add(convertToType(fromF));
return ret;
}
@SuppressWarnings({"unchecked"})
public static R[] unwrapClass(Object[] convertable, R[] dest) {
if (convertable == null || dest == null)
return null;
if (convertable.length != dest.length)
throw new RuntimeException("convertable and dest need to be arrays of same length!");
try {
Class componentType = (Class) dest.getClass().getComponentType();
for (int x = 0; x < dest.length; ++x)
dest[x] = unwrapClass(convertable[x], componentType);
} catch (Exception e) {
e.printStackTrace();
System.err.println("fatal error!");
System.exit(1);
}
return dest;
}
@SuppressWarnings({"unchecked"})
public static R unwrapClass(Object convertable, Class iface) {
if (convertable == null)
return null;
try {
return iface.cast(((ConvertableIface extends R, ?>) convertable).unwrap());
} catch (Exception e) {
e.printStackTrace();
System.err.println("fatal error!");
System.exit(1);
}
return null;
}
public static > List convertEnums(Collection extends Enum> from, Class newe) {
List ret = new ArrayList(from.size());
for (Enum old : from)
ret.add(convertEnum(old, newe));
return ret;
}
public static > E convertEnum(Enum old, Class newe) {
return Enum.valueOf(newe, old.toString().toUpperCase());
}
public static Set toSet(Collection c) {
try {
if (c instanceof Set)
return (Set) c;
} catch (Exception e) {
// do nothing
}
Set ret = new LinkedHashSet();
ret.addAll(c);
return ret;
}
@SuppressWarnings({"unchecked"})
public static List sort(List ret) {
//System.out.println("un-sorted: "+ret);
Collections.sort(ret, toStringComparator);
//System.out.println("sorted: "+ret);
return ret;
}
@SuppressWarnings({"unchecked"})
public static Set sort(Set set) {
/*return set;*/
TreeSet ret = new TreeSet(toStringComparator);
ret.addAll(set);
return ret;
}
@SuppressWarnings({"unchecked"})
public static E[] sort(E[] ret) {
Arrays.sort(ret, toStringComparator);
return ret;
}
public static final Comparator toStringComparator = new ToStringComparator();
private static class ToStringComparator implements Comparator
© 2015 - 2024 Weber Informatics LLC | Privacy Policy