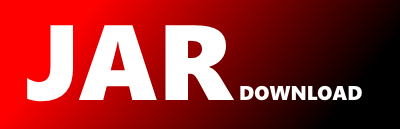
org.apache.beehive.controls.system.jdbc.parser.JdbcFragment Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* $Header:$
*/
package org.apache.beehive.controls.system.jdbc.parser;
import org.apache.beehive.controls.api.context.ControlBeanContext;
import java.lang.reflect.Method;
import java.util.ArrayList;
/**
* Represents an SQL escape sequence found in the SQL annotation's statement member. A JdbcFragment may
* contain child SqlFragments, typically these fragments consist of LiteralFragments and ReflectionFragments.
* Parameter substitutions may occur within the SQL escape delimiters {}.
*
* Syntactically an SQL escape sequence must match one of the following forms, where _space_ is a whitespace character:
*
* - {call_space_.....}
* - {?=_space_.....}
* - {d_space_.....}
* - {t_space_.....}
* - {ts_space_.....}
* - {fn_space_.....}
* - {escape_space_.....}
* - {oj_space_.....}
*/
public final class JdbcFragment extends SqlFragmentContainer {
/**
* Create a new JdbcFragment
*/
JdbcFragment() {
super();
}
/**
* Get the prepared statement parameter value(s) contained within this fragment.
*
* @param context A ControlBeanContext instance.
* @param method The annotated method.
* @param args The method's arguments.
*
* @return null if this fragment doesn't contain a parameter value.
*/
Object[] getParameterValues(ControlBeanContext context, Method method, Object[] args) {
ArrayList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy