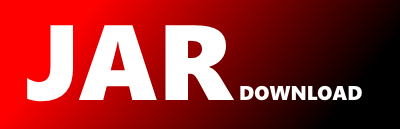
com.moviejukebox.fanarttv.model.WrapperSeries Maven / Gradle / Ivy
The newest version!
/*
* Copyright (c) 2004-2012 YAMJ Members
* http://code.google.com/p/moviejukebox/people/list
*
* Web: http://code.google.com/p/moviejukebox/
*
* This software is licensed under a Creative Commons License
* See this page: http://code.google.com/p/moviejukebox/wiki/License
*
* For any reuse or distribution, you must make clear to others the
* license terms of this work.
*/
package com.moviejukebox.fanarttv.model;
import java.util.EnumMap;
import java.util.List;
import java.util.Map;
import org.apache.log4j.Logger;
import org.codehaus.jackson.annotate.JsonAnySetter;
import org.codehaus.jackson.annotate.JsonProperty;
/**
* JSON Wrapper class for Movie artwork from Fanart.TV Not intended for use
* outside of the API
*
* @author stuart.boston
*/
public class WrapperSeries {
private static final Logger LOGGER = Logger.getLogger(WrapperSeries.class);
@JsonProperty("thetvdb_id")
private String tvdbid;
@JsonProperty("clearlogo")
private List clearLogo;
@JsonProperty("clearart")
private List clearArt;
@JsonProperty("tvthumb")
private List tvThumb;
@JsonProperty("seasonthumb")
private List seasonThumb;
@JsonProperty("characterart")
private List characterArt;
@JsonProperty("showbackground")
private List showBackground;
/**
* Get the TheTVDB ID
*
* @return
*/
public String getTvdbid() {
return tvdbid;
}
/**
* Set the TheTVDB ID
*
* @param tvdbid
*/
public void setTvdbid(String tvdbid) {
this.tvdbid = tvdbid;
}
/**
* Set the ClearArt artwork list
*
* @param clearArt
*/
public void setClearArt(List clearArt) {
this.clearArt = clearArt;
}
/**
* Set the ClearLogo artwork list
*
* @param clearLogo
*/
public void setClearLogo(List clearLogo) {
this.clearLogo = clearLogo;
}
/**
* Set the SeasonThumb artwork list
*
* @param seasonThumb
*/
public void setSeasonThumb(List seasonThumb) {
this.seasonThumb = seasonThumb;
}
/**
* Set the TvThumb artwork list
*
* @param tvThumb
*/
public void setTvThumb(List tvThumb) {
this.tvThumb = tvThumb;
}
/**
* Set the CharacterArt artwork list
*
* @param characterArt
*/
public void setCharacterArt(List characterArt) {
this.characterArt = characterArt;
}
/**
* Set the ShowBackground artwork list
*
* @param showBackground
*/
public void setShowBackground(List showBackground) {
this.showBackground = showBackground;
}
/**
* Get a map of the artwork types keyed by the artwork type
*
* @return
*/
public Map> getArtwork() {
Map> artwork = new EnumMap>(FTArtworkType.class);
artwork.put(FTArtworkType.CHARACTERART, characterArt);
artwork.put(FTArtworkType.CLEARART, clearArt);
artwork.put(FTArtworkType.CLEARLOGO, clearLogo);
artwork.put(FTArtworkType.SEASONTHUMB, seasonThumb);
artwork.put(FTArtworkType.TVTHUMB, tvThumb);
artwork.put(FTArtworkType.SHOWBACKGROUND, showBackground);
return artwork;
}
/**
* Handle unknown properties and print a message
*
* @param key
* @param value
*/
@JsonAnySetter
public void handleUnknown(String key, Object value) {
StringBuilder sb = new StringBuilder();
sb.append("Unknown property: '").append(key);
sb.append("' value: '").append(value).append("'");
LOGGER.warn(sb.toString());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy