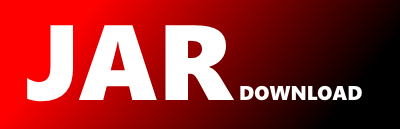
com.mozu.encryptor.EncryptionUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mozu-java-security Show documentation
Show all versions of mozu-java-security Show documentation
Mozu Java Security is an add=on library to provide security helper functions for applications using Mozu
package com.mozu.encryptor;
import org.jasypt.util.text.BasicTextEncryptor;
/**
* This class is used to encrypt and decrypt sensitive data in data stores used by mozu applications.
* @author bob_hewett
*
*/
public class EncryptionUtil {
/**
* Encrypts the value by using 'key' as salt value for the password.
* @param key salt value used to encrypt the value
* @param value the string to encrypt
* @return an encrypted string.
*/
static public String encrypt(String key, String value) {
if (value==null) {
throw new IllegalArgumentException("String to be encrypted required");
}
BasicTextEncryptor textEncryptor = new BasicTextEncryptor();
if (key==null) {
throw new IllegalArgumentException("Password key required");
}
textEncryptor.setPassword(key);
return textEncryptor.encrypt(value);
}
/**
* Decrypt the encrypted string with the salt key that was used to encrypt it.
* @param key the salt to add to encryption password.
* @param encryptedStr the string to decrypt
* @return a string in clear text.
*/
static public String decrypt(String key, String encryptedStr) {
if (encryptedStr==null) {
throw new IllegalArgumentException("String to be decrypted required");
}
BasicTextEncryptor textEncryptor = new BasicTextEncryptor();
textEncryptor.setPassword(key);
return textEncryptor.decrypt(encryptedStr);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy