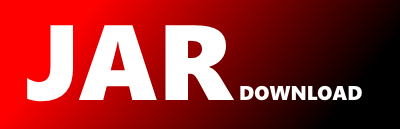
com.mozu.encryptor.Encryptor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mozu-java-security Show documentation
Show all versions of mozu-java-security Show documentation
Mozu Java Security is an add=on library to provide security helper functions for applications using Mozu
package com.mozu.encryptor;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import org.apache.commons.cli.BasicParser;
import org.apache.commons.cli.CommandLine;
import org.apache.commons.cli.CommandLineParser;
import org.apache.commons.cli.HelpFormatter;
import org.apache.commons.cli.Option;
import org.apache.commons.cli.OptionBuilder;
import org.apache.commons.cli.Options;
import org.apache.commons.cli.ParseException;
import org.apache.commons.lang3.StringUtils;
public class Encryptor {
public static final String PROPERTY_KEY_PHRASE = "2wDe2#4aq%fq23@1av'Pjsdf9(2!FsaF$FbwIz^gH";
public static final String HELP_OPTION = "?";
public static final String VALUE_OPTION = "v";
public static final String KEY_OPTION = "k";
public static void main(String[] args) {
Options options = addOptions ();
CommandLineParser parser = new BasicParser();
CommandLine cmd = null;
try {
cmd = parser.parse(options, args);
} catch (ParseException pe) {
System.out.println ("Unable to parse command line: " + pe.getMessage());
printHelp(options);
return;
}
if (cmd.hasOption(HELP_OPTION)) {
printHelp(options);
}
String saltValue = null;
if (cmd.hasOption(KEY_OPTION)) {
saltValue = cmd.getOptionValue(KEY_OPTION);
} else {
saltValue = PROPERTY_KEY_PHRASE;
}
String clearProperty = null;
if (cmd.hasOption(VALUE_OPTION)) {
clearProperty = cmd.getOptionValue(VALUE_OPTION);
} else {
//read the password, without echoing the output
BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(System.in));
String line = null;
try {
System.out.println("Enter property value to encrypt: ");
line = bufferedReader.readLine();
if (StringUtils.isNoneBlank(line)) {
clearProperty = line;
}
} catch (IOException ioe) {
System.out.println("Unable to read console input:" + ioe.getMessage());
}
}
if (clearProperty != null) {
System.out.println("Welcome to the Mozu (tm) Property Encryptor Application.");
System.out.println("Encrypted Value:" + PropertyEncryptionUtil.encryptProperty(saltValue,clearProperty));
}
}
@SuppressWarnings("static-access")
private static Options addOptions() {
Options options = new Options();
options.addOption(HELP_OPTION, "help", false, "Print this message. The help text.");
Option valueOption = OptionBuilder.withArgName("value").withLongOpt("value").hasArg().withDescription("The value to encrypt").create("v");
Option keyOption = OptionBuilder.withArgName("salt").withLongOpt("key").hasArg().withDescription("The salt value to use in encryption.").create("k");
options.addOption(valueOption);
options.addOption(keyOption);
return options;
}
private static void printHelp(Options options) {
HelpFormatter helpFormatter = new HelpFormatter();
helpFormatter.printHelp("Encryptor", options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy