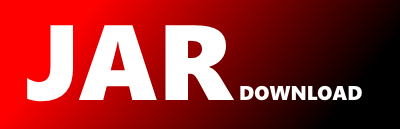
com.mtnfog.idyl.ami.sdk.IdylAmiClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of idyl-ami-java-sdk Show documentation
Show all versions of idyl-ami-java-sdk Show documentation
Java SDK for Mountain Fog's Idyl AMI.
The newest version!
/* Copyright 2014-2015 Mountain Fog, Inc.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License. */
package com.mtnfog.idyl.ami.sdk;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.URLEncoder;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.apache.commons.codec.binary.Base64;
import org.apache.commons.io.IOUtils;
import org.apache.http.HttpResponse;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.impl.client.HttpClientBuilder;
import org.apache.logging.log4j.Logger;
import org.apache.logging.log4j.LogManager;
import com.google.gson.Gson;
import com.google.gson.reflect.TypeToken;
import com.mtnfog.idyl.ami.sdk.model.Entity;
/**
* The IdylClient supports sending entity extraction requests to the
* Idyl AMI instances.
* @author mtnfog
*/
public class IdylAmiClient {
private static final Logger LOGGER = LogManager.getLogger(IdylAmiClient.class);
private static final int HTTP_OK = 200;
/**
* The Idyl AMI REST endpoint.
*/
private String endpoint;
/**
* Used for deserializing the JSON response from the Idyl AMI.
*/
private Gson gson;
/**
* The username for the Idyl AMI user.
*/
private String userName;
/**
* The password for the Idyl AMI user.
*/
private String password;
/**
* Initialize a new Idyl client with the endpoint.
* @param endpoint The Idyl AMI endpoint.
*/
public IdylAmiClient(String endpoint, String userName, String password) {
this.endpoint = endpoint;
this.userName = userName;
this.password = password;
this.gson = new Gson();
}
/**
* Causes any integrations with batches whose length is greater than
* zero to be immediately processed.
* @throws IOException
*/
public void flush() throws IOException {
// Add the operation to the endpoint.
String url = endpoint + "/flush";
// Send it as a POST to Idyl AMI.
HttpResponse response = executeHttpPostRequest(url);
// Get the response code.
int responseCode = response.getStatusLine().getStatusCode();
// Check the response code.
if(responseCode == HTTP_OK) {
// Nothing to do.
} else {
// Something went wrong that the entities could not be extracted.
// Check the Idyl AMI log for details.
LOGGER.warn("Unable to flush the integration batches. Check the Idyl AMI log for details.");
}
}
/**
* Get the current count of items in the batches for the integrations
* that support batching.
* @return
* @throws IOException
*/
public Map getBatchSizes() throws IOException {
Map batchSizes = new HashMap();
// Add the operation to the endpoint.
String url = endpoint + "/batchsize";
// Send it as a POST to Idyl AMI.
HttpResponse response = executeHttpGetRequest(url);
// Get the response code.
int responseCode = response.getStatusLine().getStatusCode();
// Check the response code.
if(responseCode == HTTP_OK) {
// Read the response.
BufferedReader rd = new BufferedReader(new InputStreamReader(response.getEntity().getContent()));
// Read the response as a string.
String json = IOUtils.toString(rd);
// Close the reader.
rd.close();
// Convert the json to an oject.
batchSizes = gson.fromJson(json, new TypeToken
© 2015 - 2024 Weber Informatics LLC | Privacy Policy