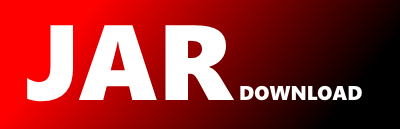
mtons.freemarker.handler.RenderHandler Maven / Gradle / Ivy
/*
+--------------------------------------------------------------------------
| Mtons [#RELEASE_VERSION#]
| ========================================
| Copyright (c) 2014, 2015 mtons. All Rights Reserved
| http://www.mtons.com
+---------------------------------------------------------------------------
*/
package mtons.freemarker.handler;
import java.io.IOException;
import java.util.Collection;
import java.util.Date;
/**
* Template Render
*
* Created by langhsu on 2015/10/2.
*/
public interface RenderHandler {
/**
* 渲染, 前提是body不为空
*
* @throws IOException
* @throws Exception
*/
void render() throws Exception;
/**
* 向页面输出一个字符串, 一般为行标签使用
* @param text
* @throws Exception
*/
void renderString(String text) throws Exception;
/**
* 控制变量不为空时,导出所有变量
*
* @param notEmptyObject
* @throws IOException
* @throws Exception
*/
void renderIfNotNull(Object notEmptyObject) throws Exception;
/**
* 控制变量不为空时,导出所有变量
*
* @param notEmptyCollection
* @throws IOException
* @throws Exception
*/
void renderIfNotEmpty(Collection notEmptyCollection) throws Exception;
/**
* put 临时变量, 在directive上下文中有效
*
* @param key
* @param value
* @return this
*/
RenderHandler put(String key, Object value);
/**
* 获取 String 参数
*
* @param name
* @return string
* @throws Exception
*/
String getString(String name) throws Exception;
/**
* 获取 String 参数
*
* @param name
* @param defaultValue
* @return string
* @throws Exception
*/
String getString(String name, String defaultValue) throws Exception;
/**
* 获取 Integer 参数
*
* @param name
* @return Integer
* @throws Exception
*/
Integer getInteger(String name) throws Exception;
/**
* 获取 Integer 参数
*
* @param name
* @param defaultValue
* @return
* @throws Exception
*/
Integer getInteger(String name, int defaultValue) throws Exception;
/**
* 获取 short 参数
*
* @param name
* @return
* @throws Exception
*/
Short getShort(String name) throws Exception;
/**
* 获取 Long 参数
*
* @param name
* @return
* @throws Exception
*/
Long getLong(String name) throws Exception;
/**
* 获取 Long 参数
*
* @param name
* @param defaultValue
* @return
* @throws Exception
*/
Long getLong(String name, long defaultValue) throws Exception;
/**
* 获取 Double 参数
*
* @param name
* @return
* @throws Exception
*/
Double getDouble(String name) throws Exception;
/**
* 获取 Integer 数组
*
* @param name
* @return
* @throws Exception
*/
Integer[] getIntegerArray(String name) throws Exception;
/**
* 获取 Long 数组
*
* @param name
* @return
* @throws Exception
*/
Long[] getLongArray(String name) throws Exception;
/**
* 获取 String 数组
*
* @param name
* @return
* @throws Exception
*/
String[] getStringArray(String name) throws Exception;
/**
* 获取 Boolean 参数
*
* @param name
* @return
* @throws Exception
*/
Boolean getBoolean(String name) throws Exception;
/**
* 获取 Boolean 参数
*
* @param name
* @param defaultValue
* @return
* @throws Exception
*/
Boolean getBoolean(String name, boolean defaultValue) throws Exception;
/**
* 获取 Date 参数
*
* @param name
* @return
* @throws Exception
*/
Date getDate(String name) throws Exception;
String getContextPath();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy