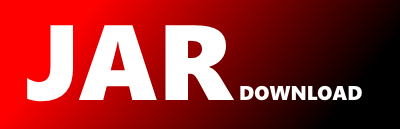
mtons.oauth2.provider.AbstractOauth2Provider Maven / Gradle / Ivy
/*
+--------------------------------------------------------------------------
| Mtons [#RELEASE_VERSION#]
| ========================================
| Copyright (c) 2014, 2015 mtons. All Rights Reserved
| http://www.mtons.com
+---------------------------------------------------------------------------
*/
package mtons.oauth2.provider;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import mtons.oauth2.Oauth2Provider;
import mtons.oauth2.utils.HttpKitExt;
import mtons.utils.HttpUtils;
import mtons.utils.PropertiesLoader;
import java.util.Map;
/**
* 第一步,构建跳转的URL,跳转后用户登录成功,返回到callback url,并带上code
* 第二步,通过code,获取access token
* 第三步,通过 access token 获取用户的open_id
* 第四步,通过 open_id 获取用户信息
* Created by langhsu on 2017/9/3.
*/
public abstract class AbstractOauth2Provider implements Oauth2Provider {
private static transient PropertiesLoader prop = new PropertiesLoader("oauth.properties");
private static final Log LOGGER = LogFactory.getLog(AbstractOauth2Provider.class);
private String key;
private String clientId;
private String clientSecret;
private String redirectUri;
public AbstractOauth2Provider() {
String name = this.getClass().getSimpleName();
name = name.substring(0, name.indexOf("Provider")).toLowerCase();
key = name;
clientId = prop.getProperty(name + ".openid");
clientSecret = prop.getProperty(name + ".openkey");
redirectUri = prop.getProperty(name + ".redirect");
}
public String getKey() { return key; }
public String getClientId() {
return clientId;
}
public String getClientSecret() {
return clientSecret;
}
public String getRedirectUri() {
return redirectUri;
}
public String getAuthorizeUrl(String state, String redirectUri) {
this.redirectUri = redirectUri;
return createAuthorizeUrl(state);
}
protected String httpGet(String url) {
try {
return HttpUtils.get(url);
} catch (Exception e) {
LOGGER.error("httpGet error", e);
}
return null;
}
protected String httpGetWithHeaders(String url, Map headers) {
try {
return HttpUtils.get(url, null, headers);
} catch (Exception e) {
LOGGER.error("httpGet error", e);
}
return null;
}
protected String httpPost(String url, Map params) {
try {
return HttpUtils.post(url, HttpKitExt.map2Url(params));
} catch (Exception e) {
LOGGER.error("httpGet error", e);
}
return null;
}
public abstract String createAuthorizeUrl(String state);
// protected abstract OauthUser getOauthUser(String code);
// public OauthUser getUser(String code) {
// return getOauthUser(code);
// }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy