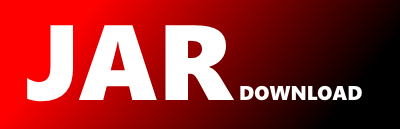
com.mux.sdk.models.CreateAssetRequest Maven / Gradle / Ivy
/*
* Mux API
* Mux is how developers build online video. This API encompasses both Mux Video and Mux Data functionality to help you build your video-related projects better and faster than ever before.
*
* The version of the OpenAPI document: v1
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.mux.sdk.models;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.mux.sdk.models.CreatePlaybackIDRequest;
import com.mux.sdk.models.InputSettings;
import com.mux.sdk.models.PlaybackPolicy;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* CreateAssetRequest
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class CreateAssetRequest {
public static final String SERIALIZED_NAME_INPUT = "input";
@SerializedName(SERIALIZED_NAME_INPUT)
private java.util.List input = null;
public static final String SERIALIZED_NAME_PLAYBACK_POLICY = "playback_policy";
@SerializedName(SERIALIZED_NAME_PLAYBACK_POLICY)
private java.util.List playbackPolicy = null;
public static final String SERIALIZED_NAME_ADVANCED_PLAYBACK_POLICIES = "advanced_playback_policies";
@SerializedName(SERIALIZED_NAME_ADVANCED_PLAYBACK_POLICIES)
private java.util.List advancedPlaybackPolicies = null;
public static final String SERIALIZED_NAME_PER_TITLE_ENCODE = "per_title_encode";
@SerializedName(SERIALIZED_NAME_PER_TITLE_ENCODE)
private Boolean perTitleEncode;
public static final String SERIALIZED_NAME_PASSTHROUGH = "passthrough";
@SerializedName(SERIALIZED_NAME_PASSTHROUGH)
private String passthrough;
/**
* Specify what level of support for mp4 playback. * The `capped-1080p` option produces a single MP4 file, called `capped-1080p.mp4`, with the video resolution capped at 1080p. This option produces an `audio.m4a` file for an audio-only asset. * The `audio-only` option produces a single M4A file, called `audio.m4a` for a video or an audio-only asset. MP4 generation will error when this option is specified for a video-only asset. * The `audio-only,capped-1080p` option produces both the `audio.m4a` and `capped-1080p.mp4` files. Only the `capped-1080p.mp4` file is produced for a video-only asset, while only the `audio.m4a` file is produced for an audio-only asset. The `standard`(deprecated) option produces up to three MP4 files with different levels of resolution (`high.mp4`, `medium.mp4`, `low.mp4`, or `audio.m4a` for an audio-only asset). MP4 files are not produced for `none` (default). In most cases you should use our default HLS-based streaming playback (`{playback_id}.m3u8`) which can automatically adjust to viewers' connection speeds, but an mp4 can be useful for some legacy devices or downloading for offline playback. See the [Download your videos guide](https://docs.mux.com/guides/enable-static-mp4-renditions) for more information.
*/
@JsonAdapter(Mp4SupportEnum.Adapter.class)
public enum Mp4SupportEnum {
NONE("none"),
STANDARD("standard"),
CAPPED_1080P("capped-1080p"),
AUDIO_ONLY("audio-only"),
AUDIO_ONLY_CAPPED_1080P("audio-only,capped-1080p");
private String value;
Mp4SupportEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static Mp4SupportEnum fromValue(String value) {
for (Mp4SupportEnum b : Mp4SupportEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final Mp4SupportEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public Mp4SupportEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return Mp4SupportEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_MP4_SUPPORT = "mp4_support";
@SerializedName(SERIALIZED_NAME_MP4_SUPPORT)
private Mp4SupportEnum mp4Support;
public static final String SERIALIZED_NAME_NORMALIZE_AUDIO = "normalize_audio";
@SerializedName(SERIALIZED_NAME_NORMALIZE_AUDIO)
private Boolean normalizeAudio = false;
/**
* Specify what level (if any) of support for master access. Master access can be enabled temporarily for your asset to be downloaded. See the [Download your videos guide](https://docs.mux.com/guides/enable-static-mp4-renditions) for more information.
*/
@JsonAdapter(MasterAccessEnum.Adapter.class)
public enum MasterAccessEnum {
NONE("none"),
TEMPORARY("temporary");
private String value;
MasterAccessEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static MasterAccessEnum fromValue(String value) {
for (MasterAccessEnum b : MasterAccessEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final MasterAccessEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public MasterAccessEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return MasterAccessEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_MASTER_ACCESS = "master_access";
@SerializedName(SERIALIZED_NAME_MASTER_ACCESS)
private MasterAccessEnum masterAccess;
public static final String SERIALIZED_NAME_TEST = "test";
@SerializedName(SERIALIZED_NAME_TEST)
private Boolean test;
/**
* Max resolution tier can be used to control the maximum `resolution_tier` your asset is encoded, stored, and streamed at. If not set, this defaults to `1080p`.
*/
@JsonAdapter(MaxResolutionTierEnum.Adapter.class)
public enum MaxResolutionTierEnum {
_1080P("1080p"),
_1440P("1440p"),
_2160P("2160p");
private String value;
MaxResolutionTierEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static MaxResolutionTierEnum fromValue(String value) {
for (MaxResolutionTierEnum b : MaxResolutionTierEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final MaxResolutionTierEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public MaxResolutionTierEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return MaxResolutionTierEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_MAX_RESOLUTION_TIER = "max_resolution_tier";
@SerializedName(SERIALIZED_NAME_MAX_RESOLUTION_TIER)
private MaxResolutionTierEnum maxResolutionTier;
/**
* This field is deprecated. Please use `video_quality` instead. The encoding tier informs the cost, quality, and available platform features for the asset. The default encoding tier for an account can be set in the Mux Dashboard. [See the video quality guide for more details.](https://docs.mux.com/guides/use-video-quality-levels)
*/
@JsonAdapter(EncodingTierEnum.Adapter.class)
public enum EncodingTierEnum {
SMART("smart"),
BASELINE("baseline"),
PREMIUM("premium");
private String value;
EncodingTierEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static EncodingTierEnum fromValue(String value) {
for (EncodingTierEnum b : EncodingTierEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final EncodingTierEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public EncodingTierEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return EncodingTierEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_ENCODING_TIER = "encoding_tier";
@SerializedName(SERIALIZED_NAME_ENCODING_TIER)
private EncodingTierEnum encodingTier;
/**
* The video quality controls the cost, quality, and available platform features for the asset. The default video quality for an account can be set in the Mux Dashboard. This field replaces the deprecated `encoding_tier` value. [See the video quality guide for more details.](https://docs.mux.com/guides/use-video-quality-levels)
*/
@JsonAdapter(VideoQualityEnum.Adapter.class)
public enum VideoQualityEnum {
BASIC("basic"),
PLUS("plus"),
PREMIUM("premium");
private String value;
VideoQualityEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static VideoQualityEnum fromValue(String value) {
for (VideoQualityEnum b : VideoQualityEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final VideoQualityEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public VideoQualityEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return VideoQualityEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_VIDEO_QUALITY = "video_quality";
@SerializedName(SERIALIZED_NAME_VIDEO_QUALITY)
private VideoQualityEnum videoQuality;
public CreateAssetRequest input(java.util.List input) {
this.input = input;
return this;
}
public CreateAssetRequest addInputItem(InputSettings inputItem) {
if (this.input == null) {
this.input = new java.util.ArrayList<>();
}
this.input.add(inputItem);
return this;
}
/**
* An array of objects that each describe an input file to be used to create the asset. As a shortcut, input can also be a string URL for a file when only one input file is used. See `input[].url` for requirements.
* @return input
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An array of objects that each describe an input file to be used to create the asset. As a shortcut, input can also be a string URL for a file when only one input file is used. See `input[].url` for requirements.")
public java.util.List getInput() {
return input;
}
public void setInput(java.util.List input) {
this.input = input;
}
public CreateAssetRequest playbackPolicy(java.util.List playbackPolicy) {
this.playbackPolicy = playbackPolicy;
return this;
}
public CreateAssetRequest addPlaybackPolicyItem(PlaybackPolicy playbackPolicyItem) {
if (this.playbackPolicy == null) {
this.playbackPolicy = new java.util.ArrayList<>();
}
this.playbackPolicy.add(playbackPolicyItem);
return this;
}
/**
* An array of playback policy names that you want applied to this asset and available through `playback_ids`. Options include: * `\"public\"` (anyone with the playback URL can stream the asset). * `\"signed\"` (an additional access token is required to play the asset). If no `playback_policy` is set, the asset will have no playback IDs and will therefore not be playable. For simplicity, a single string name can be used in place of the array in the case of only one playback policy.
* @return playbackPolicy
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An array of playback policy names that you want applied to this asset and available through `playback_ids`. Options include: * `\"public\"` (anyone with the playback URL can stream the asset). * `\"signed\"` (an additional access token is required to play the asset). If no `playback_policy` is set, the asset will have no playback IDs and will therefore not be playable. For simplicity, a single string name can be used in place of the array in the case of only one playback policy. ")
public java.util.List getPlaybackPolicy() {
return playbackPolicy;
}
public void setPlaybackPolicy(java.util.List playbackPolicy) {
this.playbackPolicy = playbackPolicy;
}
public CreateAssetRequest advancedPlaybackPolicies(java.util.List advancedPlaybackPolicies) {
this.advancedPlaybackPolicies = advancedPlaybackPolicies;
return this;
}
public CreateAssetRequest addAdvancedPlaybackPoliciesItem(CreatePlaybackIDRequest advancedPlaybackPoliciesItem) {
if (this.advancedPlaybackPolicies == null) {
this.advancedPlaybackPolicies = new java.util.ArrayList<>();
}
this.advancedPlaybackPolicies.add(advancedPlaybackPoliciesItem);
return this;
}
/**
* An array of playback policy objects that you want applied to this asset and available through `playback_ids`. `advanced_playback_policies` must be used instead of `playback_policy` when creating a DRM playback ID.
* @return advancedPlaybackPolicies
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An array of playback policy objects that you want applied to this asset and available through `playback_ids`. `advanced_playback_policies` must be used instead of `playback_policy` when creating a DRM playback ID. ")
public java.util.List getAdvancedPlaybackPolicies() {
return advancedPlaybackPolicies;
}
public void setAdvancedPlaybackPolicies(java.util.List advancedPlaybackPolicies) {
this.advancedPlaybackPolicies = advancedPlaybackPolicies;
}
public CreateAssetRequest perTitleEncode(Boolean perTitleEncode) {
this.perTitleEncode = perTitleEncode;
return this;
}
/**
* Get perTitleEncode
* @return perTitleEncode
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Boolean getPerTitleEncode() {
return perTitleEncode;
}
public void setPerTitleEncode(Boolean perTitleEncode) {
this.perTitleEncode = perTitleEncode;
}
public CreateAssetRequest passthrough(String passthrough) {
this.passthrough = passthrough;
return this;
}
/**
* Arbitrary user-supplied metadata that will be included in the asset details and related webhooks. Can be used to store your own ID for a video along with the asset. **Max: 255 characters**.
* @return passthrough
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Arbitrary user-supplied metadata that will be included in the asset details and related webhooks. Can be used to store your own ID for a video along with the asset. **Max: 255 characters**.")
public String getPassthrough() {
return passthrough;
}
public void setPassthrough(String passthrough) {
this.passthrough = passthrough;
}
public CreateAssetRequest mp4Support(Mp4SupportEnum mp4Support) {
this.mp4Support = mp4Support;
return this;
}
/**
* Specify what level of support for mp4 playback. * The `capped-1080p` option produces a single MP4 file, called `capped-1080p.mp4`, with the video resolution capped at 1080p. This option produces an `audio.m4a` file for an audio-only asset. * The `audio-only` option produces a single M4A file, called `audio.m4a` for a video or an audio-only asset. MP4 generation will error when this option is specified for a video-only asset. * The `audio-only,capped-1080p` option produces both the `audio.m4a` and `capped-1080p.mp4` files. Only the `capped-1080p.mp4` file is produced for a video-only asset, while only the `audio.m4a` file is produced for an audio-only asset. The `standard`(deprecated) option produces up to three MP4 files with different levels of resolution (`high.mp4`, `medium.mp4`, `low.mp4`, or `audio.m4a` for an audio-only asset). MP4 files are not produced for `none` (default). In most cases you should use our default HLS-based streaming playback (`{playback_id}.m3u8`) which can automatically adjust to viewers' connection speeds, but an mp4 can be useful for some legacy devices or downloading for offline playback. See the [Download your videos guide](https://docs.mux.com/guides/enable-static-mp4-renditions) for more information.
* @return mp4Support
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specify what level of support for mp4 playback. * The `capped-1080p` option produces a single MP4 file, called `capped-1080p.mp4`, with the video resolution capped at 1080p. This option produces an `audio.m4a` file for an audio-only asset. * The `audio-only` option produces a single M4A file, called `audio.m4a` for a video or an audio-only asset. MP4 generation will error when this option is specified for a video-only asset. * The `audio-only,capped-1080p` option produces both the `audio.m4a` and `capped-1080p.mp4` files. Only the `capped-1080p.mp4` file is produced for a video-only asset, while only the `audio.m4a` file is produced for an audio-only asset. The `standard`(deprecated) option produces up to three MP4 files with different levels of resolution (`high.mp4`, `medium.mp4`, `low.mp4`, or `audio.m4a` for an audio-only asset). MP4 files are not produced for `none` (default). In most cases you should use our default HLS-based streaming playback (`{playback_id}.m3u8`) which can automatically adjust to viewers' connection speeds, but an mp4 can be useful for some legacy devices or downloading for offline playback. See the [Download your videos guide](https://docs.mux.com/guides/enable-static-mp4-renditions) for more information. ")
public Mp4SupportEnum getMp4Support() {
return mp4Support;
}
public void setMp4Support(Mp4SupportEnum mp4Support) {
this.mp4Support = mp4Support;
}
public CreateAssetRequest normalizeAudio(Boolean normalizeAudio) {
this.normalizeAudio = normalizeAudio;
return this;
}
/**
* Normalize the audio track loudness level. This parameter is only applicable to on-demand (not live) assets.
* @return normalizeAudio
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Normalize the audio track loudness level. This parameter is only applicable to on-demand (not live) assets.")
public Boolean getNormalizeAudio() {
return normalizeAudio;
}
public void setNormalizeAudio(Boolean normalizeAudio) {
this.normalizeAudio = normalizeAudio;
}
public CreateAssetRequest masterAccess(MasterAccessEnum masterAccess) {
this.masterAccess = masterAccess;
return this;
}
/**
* Specify what level (if any) of support for master access. Master access can be enabled temporarily for your asset to be downloaded. See the [Download your videos guide](https://docs.mux.com/guides/enable-static-mp4-renditions) for more information.
* @return masterAccess
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specify what level (if any) of support for master access. Master access can be enabled temporarily for your asset to be downloaded. See the [Download your videos guide](https://docs.mux.com/guides/enable-static-mp4-renditions) for more information.")
public MasterAccessEnum getMasterAccess() {
return masterAccess;
}
public void setMasterAccess(MasterAccessEnum masterAccess) {
this.masterAccess = masterAccess;
}
public CreateAssetRequest test(Boolean test) {
this.test = test;
return this;
}
/**
* Marks the asset as a test asset when the value is set to true. A Test asset can help evaluate the Mux Video APIs without incurring any cost. There is no limit on number of test assets created. Test asset are watermarked with the Mux logo, limited to 10 seconds, deleted after 24 hrs.
* @return test
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Marks the asset as a test asset when the value is set to true. A Test asset can help evaluate the Mux Video APIs without incurring any cost. There is no limit on number of test assets created. Test asset are watermarked with the Mux logo, limited to 10 seconds, deleted after 24 hrs.")
public Boolean getTest() {
return test;
}
public void setTest(Boolean test) {
this.test = test;
}
public CreateAssetRequest maxResolutionTier(MaxResolutionTierEnum maxResolutionTier) {
this.maxResolutionTier = maxResolutionTier;
return this;
}
/**
* Max resolution tier can be used to control the maximum `resolution_tier` your asset is encoded, stored, and streamed at. If not set, this defaults to `1080p`.
* @return maxResolutionTier
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Max resolution tier can be used to control the maximum `resolution_tier` your asset is encoded, stored, and streamed at. If not set, this defaults to `1080p`.")
public MaxResolutionTierEnum getMaxResolutionTier() {
return maxResolutionTier;
}
public void setMaxResolutionTier(MaxResolutionTierEnum maxResolutionTier) {
this.maxResolutionTier = maxResolutionTier;
}
public CreateAssetRequest encodingTier(EncodingTierEnum encodingTier) {
this.encodingTier = encodingTier;
return this;
}
/**
* This field is deprecated. Please use `video_quality` instead. The encoding tier informs the cost, quality, and available platform features for the asset. The default encoding tier for an account can be set in the Mux Dashboard. [See the video quality guide for more details.](https://docs.mux.com/guides/use-video-quality-levels)
* @return encodingTier
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "This field is deprecated. Please use `video_quality` instead. The encoding tier informs the cost, quality, and available platform features for the asset. The default encoding tier for an account can be set in the Mux Dashboard. [See the video quality guide for more details.](https://docs.mux.com/guides/use-video-quality-levels)")
public EncodingTierEnum getEncodingTier() {
return encodingTier;
}
public void setEncodingTier(EncodingTierEnum encodingTier) {
this.encodingTier = encodingTier;
}
public CreateAssetRequest videoQuality(VideoQualityEnum videoQuality) {
this.videoQuality = videoQuality;
return this;
}
/**
* The video quality controls the cost, quality, and available platform features for the asset. The default video quality for an account can be set in the Mux Dashboard. This field replaces the deprecated `encoding_tier` value. [See the video quality guide for more details.](https://docs.mux.com/guides/use-video-quality-levels)
* @return videoQuality
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The video quality controls the cost, quality, and available platform features for the asset. The default video quality for an account can be set in the Mux Dashboard. This field replaces the deprecated `encoding_tier` value. [See the video quality guide for more details.](https://docs.mux.com/guides/use-video-quality-levels)")
public VideoQualityEnum getVideoQuality() {
return videoQuality;
}
public void setVideoQuality(VideoQualityEnum videoQuality) {
this.videoQuality = videoQuality;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CreateAssetRequest createAssetRequest = (CreateAssetRequest) o;
return Objects.equals(this.input, createAssetRequest.input) &&
Objects.equals(this.playbackPolicy, createAssetRequest.playbackPolicy) &&
Objects.equals(this.advancedPlaybackPolicies, createAssetRequest.advancedPlaybackPolicies) &&
Objects.equals(this.perTitleEncode, createAssetRequest.perTitleEncode) &&
Objects.equals(this.passthrough, createAssetRequest.passthrough) &&
Objects.equals(this.mp4Support, createAssetRequest.mp4Support) &&
Objects.equals(this.normalizeAudio, createAssetRequest.normalizeAudio) &&
Objects.equals(this.masterAccess, createAssetRequest.masterAccess) &&
Objects.equals(this.test, createAssetRequest.test) &&
Objects.equals(this.maxResolutionTier, createAssetRequest.maxResolutionTier) &&
Objects.equals(this.encodingTier, createAssetRequest.encodingTier) &&
Objects.equals(this.videoQuality, createAssetRequest.videoQuality);
}
@Override
public int hashCode() {
return Objects.hash(input, playbackPolicy, advancedPlaybackPolicies, perTitleEncode, passthrough, mp4Support, normalizeAudio, masterAccess, test, maxResolutionTier, encodingTier, videoQuality);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CreateAssetRequest {\n");
sb.append(" input: ").append(toIndentedString(input)).append("\n");
sb.append(" playbackPolicy: ").append(toIndentedString(playbackPolicy)).append("\n");
sb.append(" advancedPlaybackPolicies: ").append(toIndentedString(advancedPlaybackPolicies)).append("\n");
sb.append(" perTitleEncode: ").append(toIndentedString(perTitleEncode)).append("\n");
sb.append(" passthrough: ").append(toIndentedString(passthrough)).append("\n");
sb.append(" mp4Support: ").append(toIndentedString(mp4Support)).append("\n");
sb.append(" normalizeAudio: ").append(toIndentedString(normalizeAudio)).append("\n");
sb.append(" masterAccess: ").append(toIndentedString(masterAccess)).append("\n");
sb.append(" test: ").append(toIndentedString(test)).append("\n");
sb.append(" maxResolutionTier: ").append(toIndentedString(maxResolutionTier)).append("\n");
sb.append(" encodingTier: ").append(toIndentedString(encodingTier)).append("\n");
sb.append(" videoQuality: ").append(toIndentedString(videoQuality)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy