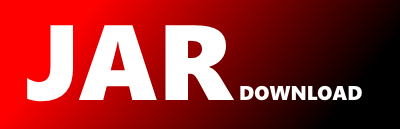
com.mux.sdk.models.LiveStreamGeneratedSubtitleSettings Maven / Gradle / Ivy
/*
* Mux API
* Mux is how developers build online video. This API encompasses both Mux Video and Mux Data functionality to help you build your video-related projects better and faster than ever before.
*
* The version of the OpenAPI document: v1
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.mux.sdk.models;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* LiveStreamGeneratedSubtitleSettings
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class LiveStreamGeneratedSubtitleSettings {
public static final String SERIALIZED_NAME_NAME = "name";
@SerializedName(SERIALIZED_NAME_NAME)
private String name;
public static final String SERIALIZED_NAME_PASSTHROUGH = "passthrough";
@SerializedName(SERIALIZED_NAME_PASSTHROUGH)
private String passthrough;
/**
* The language to generate subtitles in.
*/
@JsonAdapter(LanguageCodeEnum.Adapter.class)
public enum LanguageCodeEnum {
EN("en"),
EN_US("en-US");
private String value;
LanguageCodeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static LanguageCodeEnum fromValue(String value) {
for (LanguageCodeEnum b : LanguageCodeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final LanguageCodeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public LanguageCodeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return LanguageCodeEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_LANGUAGE_CODE = "language_code";
@SerializedName(SERIALIZED_NAME_LANGUAGE_CODE)
private LanguageCodeEnum languageCode = LanguageCodeEnum.EN;
public static final String SERIALIZED_NAME_TRANSCRIPTION_VOCABULARY_IDS = "transcription_vocabulary_ids";
@SerializedName(SERIALIZED_NAME_TRANSCRIPTION_VOCABULARY_IDS)
private java.util.List transcriptionVocabularyIds = null;
public LiveStreamGeneratedSubtitleSettings name(String name) {
this.name = name;
return this;
}
/**
* A name for this live stream subtitle track.
* @return name
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A name for this live stream subtitle track.")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public LiveStreamGeneratedSubtitleSettings passthrough(String passthrough) {
this.passthrough = passthrough;
return this;
}
/**
* Arbitrary metadata set for the live stream subtitle track. Max 255 characters.
* @return passthrough
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Arbitrary metadata set for the live stream subtitle track. Max 255 characters.")
public String getPassthrough() {
return passthrough;
}
public void setPassthrough(String passthrough) {
this.passthrough = passthrough;
}
public LiveStreamGeneratedSubtitleSettings languageCode(LanguageCodeEnum languageCode) {
this.languageCode = languageCode;
return this;
}
/**
* The language to generate subtitles in.
* @return languageCode
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The language to generate subtitles in.")
public LanguageCodeEnum getLanguageCode() {
return languageCode;
}
public void setLanguageCode(LanguageCodeEnum languageCode) {
this.languageCode = languageCode;
}
public LiveStreamGeneratedSubtitleSettings transcriptionVocabularyIds(java.util.List transcriptionVocabularyIds) {
this.transcriptionVocabularyIds = transcriptionVocabularyIds;
return this;
}
public LiveStreamGeneratedSubtitleSettings addTranscriptionVocabularyIdsItem(String transcriptionVocabularyIdsItem) {
if (this.transcriptionVocabularyIds == null) {
this.transcriptionVocabularyIds = new java.util.ArrayList<>();
}
this.transcriptionVocabularyIds.add(transcriptionVocabularyIdsItem);
return this;
}
/**
* Unique identifiers for existing Transcription Vocabularies to use while generating subtitles for the live stream. If the Transcription Vocabularies provided collectively have more than 1000 phrases, only the first 1000 phrases will be included.
* @return transcriptionVocabularyIds
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifiers for existing Transcription Vocabularies to use while generating subtitles for the live stream. If the Transcription Vocabularies provided collectively have more than 1000 phrases, only the first 1000 phrases will be included.")
public java.util.List getTranscriptionVocabularyIds() {
return transcriptionVocabularyIds;
}
public void setTranscriptionVocabularyIds(java.util.List transcriptionVocabularyIds) {
this.transcriptionVocabularyIds = transcriptionVocabularyIds;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
LiveStreamGeneratedSubtitleSettings liveStreamGeneratedSubtitleSettings = (LiveStreamGeneratedSubtitleSettings) o;
return Objects.equals(this.name, liveStreamGeneratedSubtitleSettings.name) &&
Objects.equals(this.passthrough, liveStreamGeneratedSubtitleSettings.passthrough) &&
Objects.equals(this.languageCode, liveStreamGeneratedSubtitleSettings.languageCode) &&
Objects.equals(this.transcriptionVocabularyIds, liveStreamGeneratedSubtitleSettings.transcriptionVocabularyIds);
}
@Override
public int hashCode() {
return Objects.hash(name, passthrough, languageCode, transcriptionVocabularyIds);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class LiveStreamGeneratedSubtitleSettings {\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" passthrough: ").append(toIndentedString(passthrough)).append("\n");
sb.append(" languageCode: ").append(toIndentedString(languageCode)).append("\n");
sb.append(" transcriptionVocabularyIds: ").append(toIndentedString(transcriptionVocabularyIds)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy