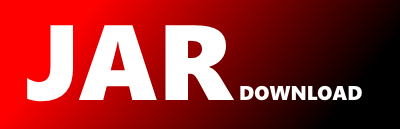
com.mux.sdk.models.Score Maven / Gradle / Ivy
/*
* Mux API
* Mux is how developers build online video. This API encompasses both Mux Video and Mux Data functionality to help you build your video-related projects better and faster than ever before.
*
* The version of the OpenAPI document: v1
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.mux.sdk.models;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.mux.sdk.models.Metric;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* Score
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class Score {
public static final String SERIALIZED_NAME_WATCH_TIME = "watch_time";
@SerializedName(SERIALIZED_NAME_WATCH_TIME)
private Long watchTime;
public static final String SERIALIZED_NAME_VIEW_COUNT = "view_count";
@SerializedName(SERIALIZED_NAME_VIEW_COUNT)
private Long viewCount;
public static final String SERIALIZED_NAME_UNIQUE_VIEWERS = "unique_viewers";
@SerializedName(SERIALIZED_NAME_UNIQUE_VIEWERS)
private Long uniqueViewers;
public static final String SERIALIZED_NAME_STARTED_VIEWS = "started_views";
@SerializedName(SERIALIZED_NAME_STARTED_VIEWS)
private Long startedViews;
public static final String SERIALIZED_NAME_TOTAL_PLAYING_TIME = "total_playing_time";
@SerializedName(SERIALIZED_NAME_TOTAL_PLAYING_TIME)
private Long totalPlayingTime;
public static final String SERIALIZED_NAME_NAME = "name";
@SerializedName(SERIALIZED_NAME_NAME)
private String name;
public static final String SERIALIZED_NAME_ENDED_VIEWS = "ended_views";
@SerializedName(SERIALIZED_NAME_ENDED_VIEWS)
private Long endedViews;
public static final String SERIALIZED_NAME_VALUE = "value";
@SerializedName(SERIALIZED_NAME_VALUE)
private Double value;
public static final String SERIALIZED_NAME_TYPE = "type";
@SerializedName(SERIALIZED_NAME_TYPE)
private String type;
public static final String SERIALIZED_NAME_METRIC = "metric";
@SerializedName(SERIALIZED_NAME_METRIC)
private String metric;
public static final String SERIALIZED_NAME_ITEMS = "items";
@SerializedName(SERIALIZED_NAME_ITEMS)
private java.util.List items = null;
public Score watchTime(Long watchTime) {
this.watchTime = watchTime;
return this;
}
/**
* Get watchTime
* @return watchTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Long getWatchTime() {
return watchTime;
}
public void setWatchTime(Long watchTime) {
this.watchTime = watchTime;
}
public Score viewCount(Long viewCount) {
this.viewCount = viewCount;
return this;
}
/**
* Get viewCount
* @return viewCount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Long getViewCount() {
return viewCount;
}
public void setViewCount(Long viewCount) {
this.viewCount = viewCount;
}
public Score uniqueViewers(Long uniqueViewers) {
this.uniqueViewers = uniqueViewers;
return this;
}
/**
* Get uniqueViewers
* @return uniqueViewers
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Long getUniqueViewers() {
return uniqueViewers;
}
public void setUniqueViewers(Long uniqueViewers) {
this.uniqueViewers = uniqueViewers;
}
public Score startedViews(Long startedViews) {
this.startedViews = startedViews;
return this;
}
/**
* Get startedViews
* @return startedViews
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Long getStartedViews() {
return startedViews;
}
public void setStartedViews(Long startedViews) {
this.startedViews = startedViews;
}
public Score totalPlayingTime(Long totalPlayingTime) {
this.totalPlayingTime = totalPlayingTime;
return this;
}
/**
* Get totalPlayingTime
* @return totalPlayingTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Long getTotalPlayingTime() {
return totalPlayingTime;
}
public void setTotalPlayingTime(Long totalPlayingTime) {
this.totalPlayingTime = totalPlayingTime;
}
public Score name(String name) {
this.name = name;
return this;
}
/**
* Get name
* @return name
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Score endedViews(Long endedViews) {
this.endedViews = endedViews;
return this;
}
/**
* Get endedViews
* @return endedViews
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Long getEndedViews() {
return endedViews;
}
public void setEndedViews(Long endedViews) {
this.endedViews = endedViews;
}
public Score value(Double value) {
this.value = value;
return this;
}
/**
* Get value
* @return value
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Double getValue() {
return value;
}
public void setValue(Double value) {
this.value = value;
}
public Score type(String type) {
this.type = type;
return this;
}
/**
* Get type
* @return type
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public Score metric(String metric) {
this.metric = metric;
return this;
}
/**
* Get metric
* @return metric
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getMetric() {
return metric;
}
public void setMetric(String metric) {
this.metric = metric;
}
public Score items(java.util.List items) {
this.items = items;
return this;
}
public Score addItemsItem(Metric itemsItem) {
if (this.items == null) {
this.items = new java.util.ArrayList<>();
}
this.items.add(itemsItem);
return this;
}
/**
* Get items
* @return items
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public java.util.List getItems() {
return items;
}
public void setItems(java.util.List items) {
this.items = items;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Score score = (Score) o;
return Objects.equals(this.watchTime, score.watchTime) &&
Objects.equals(this.viewCount, score.viewCount) &&
Objects.equals(this.uniqueViewers, score.uniqueViewers) &&
Objects.equals(this.startedViews, score.startedViews) &&
Objects.equals(this.totalPlayingTime, score.totalPlayingTime) &&
Objects.equals(this.name, score.name) &&
Objects.equals(this.endedViews, score.endedViews) &&
Objects.equals(this.value, score.value) &&
Objects.equals(this.type, score.type) &&
Objects.equals(this.metric, score.metric) &&
Objects.equals(this.items, score.items);
}
@Override
public int hashCode() {
return Objects.hash(watchTime, viewCount, uniqueViewers, startedViews, totalPlayingTime, name, endedViews, value, type, metric, items);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Score {\n");
sb.append(" watchTime: ").append(toIndentedString(watchTime)).append("\n");
sb.append(" viewCount: ").append(toIndentedString(viewCount)).append("\n");
sb.append(" uniqueViewers: ").append(toIndentedString(uniqueViewers)).append("\n");
sb.append(" startedViews: ").append(toIndentedString(startedViews)).append("\n");
sb.append(" totalPlayingTime: ").append(toIndentedString(totalPlayingTime)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" endedViews: ").append(toIndentedString(endedViews)).append("\n");
sb.append(" value: ").append(toIndentedString(value)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" metric: ").append(toIndentedString(metric)).append("\n");
sb.append(" items: ").append(toIndentedString(items)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy