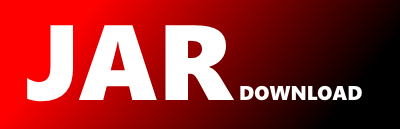
com.mx.path.model.mdx.web.controller.ProfilesController Maven / Gradle / Ivy
The newest version!
package com.mx.path.model.mdx.web.controller;
import com.mx.path.gateway.accessor.AccessorResponse;
import com.mx.path.model.mdx.model.MdxList;
import com.mx.path.model.mdx.model.challenges.Challenge;
import com.mx.path.model.mdx.model.profile.Address;
import com.mx.path.model.mdx.model.profile.ChallengeQuestions;
import com.mx.path.model.mdx.model.profile.Email;
import com.mx.path.model.mdx.model.profile.NewPassword;
import com.mx.path.model.mdx.model.profile.NewUserName;
import com.mx.path.model.mdx.model.profile.Password;
import com.mx.path.model.mdx.model.profile.Phone;
import com.mx.path.model.mdx.model.profile.Profile;
import com.mx.path.model.mdx.model.profile.SecurityQuestions;
import com.mx.path.model.mdx.model.profile.UserName;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping(value = "{clientId}", produces = BaseController.MDX_MEDIA)
public class ProfilesController extends BaseController {
public ProfilesController() {
}
@RequestMapping(value = "/users/{userId}/profile", method = RequestMethod.GET)
public final ResponseEntity getProfile() {
AccessorResponse response = gateway().profiles().get();
return new ResponseEntity<>(response.getResult().wrapped(), createMultiMapForResponse(response.getHeaders()), HttpStatus.OK);
}
@RequestMapping(value = "/users/{userId}/profile", method = RequestMethod.PUT)
public final ResponseEntity createOrUpdateProfile(@RequestBody Profile profile) {
AccessorResponse response = gateway().profiles().update(profile);
return new ResponseEntity<>(response.getResult().wrapped(), createMultiMapForResponse(response.getHeaders()), HttpStatus.OK);
}
@RequestMapping(value = "/users/{userId}/profile/addresses", method = RequestMethod.GET)
public final ResponseEntity> getAddresses() {
AccessorResponse> response = gateway().profiles().addresses().list();
return new ResponseEntity<>(response.getResult().wrapped(), createMultiMapForResponse(response.getHeaders()), HttpStatus.OK);
}
@RequestMapping(value = "/users/{userId}/profile/addresses/{addressId}", method = RequestMethod.GET)
public final ResponseEntity getAddress(@PathVariable("addressId") String addressId) {
AccessorResponse response = gateway().profiles().addresses().get(addressId);
return new ResponseEntity<>(response.getResult().wrapped(), createMultiMapForResponse(response.getHeaders()), HttpStatus.OK);
}
@RequestMapping(value = "/users/{userId}/profile/addresses", method = RequestMethod.POST)
public final ResponseEntity createAddress(@RequestBody Address address) {
AccessorResponse response = gateway().profiles().addresses().create(address);
return new ResponseEntity<>(response.getResult().wrapped(), createMultiMapForResponse(response.getHeaders()), HttpStatus.OK);
}
@RequestMapping(value = "/users/{userId}/profile/addresses/{addressId}", method = RequestMethod.PUT)
public final ResponseEntity updateAddress(@PathVariable("addressId") String addressId, @RequestBody Address address) {
address.setId(addressId);
AccessorResponse response = gateway().profiles().addresses().update(addressId, address);
Address result = response.getResult();
HttpStatus status = HttpStatus.OK;
if (result.getChallenges() != null && result.getChallenges().size() > 0) {
status = HttpStatus.ACCEPTED;
}
return new ResponseEntity<>(response.getResult().wrapped(), createMultiMapForResponse(response.getHeaders()), status);
}
@RequestMapping(value = "/users/{userId}/profile/addresses/{addressId}", method = RequestMethod.DELETE)
public final ResponseEntity> deleteAddress(@PathVariable("addressId") String addressId) {
AccessorResponse response = gateway().profiles().addresses().delete(addressId);
return new ResponseEntity<>(createMultiMapForResponse(response.getHeaders()), HttpStatus.NO_CONTENT);
}
@Deprecated
@RequestMapping(value = "/users/{userId}/profile/challenge_questions", method = RequestMethod.GET)
public final ResponseEntity getChallengeQuestions() {
AccessorResponse response = gateway().profiles().challengeQuestions().list();
return new ResponseEntity<>(response.getResult().wrapped(), createMultiMapForResponse(response.getHeaders()), HttpStatus.OK);
}
@Deprecated
@RequestMapping(value = "/users/{userId}/profile/challenge_questions", method = RequestMethod.PUT, consumes = MDX_MEDIA)
public final ResponseEntity updateChallengeQuestions(@RequestBody ChallengeQuestions challengeQuestions) {
AccessorResponse response = gateway().profiles().challengeQuestions().update(challengeQuestions);
ChallengeQuestions result = response.getResult();
if (result != null && result.getChallenges() != null && result.getChallenges().size() > 0) {
return new ResponseEntity<>(response.getResult().wrapped(), createMultiMapForResponse(response.getHeaders()), HttpStatus.ACCEPTED);
}
return new ResponseEntity<>(createMultiMapForResponse(response.getHeaders()), HttpStatus.NO_CONTENT);
}
@RequestMapping(value = "/users/{userId}/profile/security_questions", method = RequestMethod.GET)
public final ResponseEntity getSecurityQuestions() {
AccessorResponse response = gateway().profiles().securityQuestions().list();
return new ResponseEntity<>(response.getResult().wrapped(), createMultiMapForResponse(response.getHeaders()), HttpStatus.OK);
}
@RequestMapping(value = "/users/{userId}/profile/security_questions", method = RequestMethod.PUT, consumes = MDX_MEDIA)
public final ResponseEntity updateSecurityQuestions(@RequestBody SecurityQuestions securityQuestions) {
AccessorResponse response = gateway().profiles().securityQuestions().update(securityQuestions);
SecurityQuestions result = response.getResult();
if (result != null && result.getChallenges() != null && result.getChallenges().size() > 0) {
return new ResponseEntity<>(response.getResult().wrapped(), createMultiMapForResponse(response.getHeaders()), HttpStatus.ACCEPTED);
}
return new ResponseEntity<>(response.getResult().wrapped(), createMultiMapForResponse(response.getHeaders()), HttpStatus.OK);
}
@RequestMapping(value = "/users/{userId}/profile/phones", method = RequestMethod.GET)
public final ResponseEntity> getPhones() {
AccessorResponse> response = gateway().profiles().phones().list();
return new ResponseEntity<>(response.getResult().wrapped(), createMultiMapForResponse(response.getHeaders()), HttpStatus.OK);
}
@RequestMapping(value = "/users/{userId}/profile/phones/{phoneId}", method = RequestMethod.GET)
public final ResponseEntity getPhone(@PathVariable("phoneId") String phoneId) {
AccessorResponse response = gateway().profiles().phones().get(phoneId);
return new ResponseEntity<>(response.getResult().wrapped(), createMultiMapForResponse(response.getHeaders()), HttpStatus.OK);
}
@RequestMapping(value = "/users/{userId}/profile/phones", method = RequestMethod.POST)
public final ResponseEntity createPhone(@RequestBody Phone phone) {
AccessorResponse response = gateway().profiles().phones().create(phone);
Phone result = response.getResult();
HttpStatus status = HttpStatus.OK;
if (result.getChallenges() != null && result.getChallenges().size() > 0) {
status = HttpStatus.ACCEPTED;
}
return new ResponseEntity<>(response.getResult().wrapped(), createMultiMapForResponse(response.getHeaders()), status);
}
@RequestMapping(value = "/users/{userId}/profile/phones/{phoneId}", method = RequestMethod.PUT)
public final ResponseEntity updatePhone(@PathVariable("phoneId") String phoneId, @RequestBody Phone phone) {
phone.setId(phoneId);
AccessorResponse response = gateway().profiles().phones().update(phoneId, phone);
Phone result = response.getResult();
// Return 202 returning challenge questions
HttpStatus status = HttpStatus.OK;
if (result.getChallenges() != null && result.getChallenges().size() > 0) {
status = HttpStatus.ACCEPTED;
}
return new ResponseEntity<>(response.getResult().wrapped(), createMultiMapForResponse(response.getHeaders()), status);
}
@RequestMapping(value = "/users/{userId}/profile/phones/{phoneId}", method = RequestMethod.DELETE)
public final ResponseEntity deletePhone(@PathVariable("phoneId") String phoneId, @RequestBody(required = false) Phone phone) {
AccessorResponse response = gateway().profiles().phones().delete(phoneId, phone);
Phone result = response.getResult();
if (result != null && result.getChallenges() != null && result.getChallenges().size() > 0) {
return new ResponseEntity<>(response.getResult().wrapped(), createMultiMapForResponse(response.getHeaders()), HttpStatus.ACCEPTED);
}
return new ResponseEntity<>(createMultiMapForResponse(response.getHeaders()), HttpStatus.NO_CONTENT);
}
@RequestMapping(value = "/users/{userId}/profile/emails", method = RequestMethod.GET)
public final ResponseEntity> getEmails() {
AccessorResponse> response = gateway().profiles().emails().list();
return new ResponseEntity<>(response.getResult().wrapped(), createMultiMapForResponse(response.getHeaders()), HttpStatus.OK);
}
@RequestMapping(value = "/users/{userId}/profile/emails/{emailId}", method = RequestMethod.GET)
public final ResponseEntity getEmail(@PathVariable("emailId") String emailId) {
AccessorResponse response = gateway().profiles().emails().get(emailId);
return new ResponseEntity<>(response.getResult().wrapped(), createMultiMapForResponse(response.getHeaders()), HttpStatus.OK);
}
@RequestMapping(value = "/users/{userId}/profile/emails", method = RequestMethod.POST)
public final ResponseEntity createEmail(@RequestBody Email email) {
AccessorResponse response = gateway().profiles().emails().create(email);
Email result = response.getResult();
HttpStatus status = HttpStatus.OK;
if (result.getChallenges() != null && result.getChallenges().size() > 0) {
status = HttpStatus.ACCEPTED;
}
return new ResponseEntity<>(response.getResult().wrapped(), createMultiMapForResponse(response.getHeaders()), status);
}
@RequestMapping(value = "/users/{userId}/profile/emails/{emailId}", method = RequestMethod.PUT)
public final ResponseEntity updateEmail(@PathVariable("emailId") String emailId, @RequestBody Email email) {
email.setId(emailId);
AccessorResponse response = gateway().profiles().emails().update(emailId, email);
Email result = response.getResult();
HttpStatus status = HttpStatus.OK;
if (result.getChallenges() != null && result.getChallenges().size() > 0) {
status = HttpStatus.ACCEPTED;
}
return new ResponseEntity<>(response.getResult().wrapped(), createMultiMapForResponse(response.getHeaders()), status);
}
@RequestMapping(value = "/users/{userId}/profile/emails/{emailId}", method = RequestMethod.DELETE)
public final ResponseEntity deleteEmail(@PathVariable("emailId") String emailId, @RequestBody(required = false) Email email) {
AccessorResponse response = gateway().profiles().emails().delete(emailId, email);
Email result = response.getResult();
if (result != null && result.getChallenges() != null && result.getChallenges().size() > 0) {
return new ResponseEntity<>(response.getResult().wrapped(), createMultiMapForResponse(response.getHeaders()), HttpStatus.ACCEPTED);
}
return new ResponseEntity<>(createMultiMapForResponse(response.getHeaders()), HttpStatus.NO_CONTENT);
}
/**
* @deprecated This method is deprecated to allow a better interface with mfa challenges.
* Use {@link #updatePasswordWithMFA} instead.
*/
@Deprecated
@RequestMapping(value = "/users/{userId}/profile/update_password", method = RequestMethod.PUT)
public final ResponseEntity> updatePassword(@RequestBody Password password) {
AccessorResponse> response = gateway().profiles().updatePassword(password);
if (response.getResult() != null && response.getResult().size() != 0) {
return new ResponseEntity<>(response.getResult().wrapped(), createMultiMapForResponse(response.getHeaders()), HttpStatus.ACCEPTED);
}
return new ResponseEntity<>(createMultiMapForResponse(response.getHeaders()), HttpStatus.NO_CONTENT);
}
@RequestMapping(value = "/users/{userId}/profile/update_password/challenges/{challengeId}", method = RequestMethod.PUT)
public final ResponseEntity> updatePasswordResume(@PathVariable("challengeId") String challengeId, @RequestBody Challenge challenge) {
AccessorResponse> response = gateway().profiles().updatePasswordResume(challengeId, challenge);
if (response.getResult() != null && response.getResult().size() != 0) {
return new ResponseEntity<>(response.getResult().wrapped(), createMultiMapForResponse(response.getHeaders()), HttpStatus.ACCEPTED);
}
return new ResponseEntity<>(createMultiMapForResponse(response.getHeaders()), HttpStatus.NO_CONTENT);
}
/**
* @deprecated This method is deprecated to allow a better interface with mfa challenges.
* Use {@link #updateUserNameWithMFA} instead.
*/
@Deprecated
@RequestMapping(value = "/users/{userId}/profile/update_username", method = RequestMethod.PUT)
public final ResponseEntity> updateUserName(@RequestBody UserName updateUserInfo) {
AccessorResponse response = gateway().profiles().updateUserName(updateUserInfo);
return new ResponseEntity<>(createMultiMapForResponse(response.getHeaders()), HttpStatus.NO_CONTENT);
}
@RequestMapping(value = "/users/{userId}/profile/password", method = RequestMethod.PUT)
public final ResponseEntity updatePasswordWithMFA(@RequestBody NewPassword password) {
AccessorResponse response = gateway().profiles().updatePasswordWithMFA(password);
NewPassword result = response.getResult();
if (result != null && result.getChallenges() != null && result.getChallenges().size() > 0) {
return new ResponseEntity<>(response.getResult().wrapped(), createMultiMapForResponse(response.getHeaders()), HttpStatus.ACCEPTED);
}
return new ResponseEntity<>(createMultiMapForResponse(response.getHeaders()), HttpStatus.NO_CONTENT);
}
@RequestMapping(value = "/users/{userId}/profile/username", method = RequestMethod.PUT)
public final ResponseEntity updateUserNameWithMFA(@RequestBody NewUserName updateUserInfo) {
AccessorResponse response = gateway().profiles().updateUserNameWithMFA(updateUserInfo);
NewUserName result = response.getResult();
if (result != null && result.getChallenges() != null && result.getChallenges().size() > 0) {
return new ResponseEntity<>(response.getResult().wrapped(), createMultiMapForResponse(response.getHeaders()), HttpStatus.ACCEPTED);
}
return new ResponseEntity<>(createMultiMapForResponse(response.getHeaders()), HttpStatus.NO_CONTENT);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy