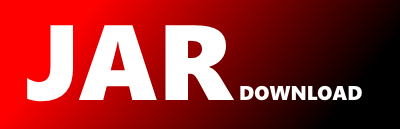
com.mybatisflex.core.query.WrapperUtil Maven / Gradle / Ivy
/*
* Copyright (c) 2022-2025, Mybatis-Flex ([email protected]).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.mybatisflex.core.query;
import com.mybatisflex.core.FlexConsts;
import com.mybatisflex.core.constant.SqlConsts;
import com.mybatisflex.core.dialect.DialectFactory;
import com.mybatisflex.core.dialect.IDialect;
import com.mybatisflex.core.dialect.impl.OracleDialect;
import com.mybatisflex.core.util.ClassUtil;
import com.mybatisflex.core.util.EnumWrapper;
import com.mybatisflex.core.util.StringUtil;
import java.lang.reflect.Array;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
class WrapperUtil {
private WrapperUtil() {
}
static List getChildQueryWrapper(QueryCondition condition) {
List list = null;
while (condition != null) {
if (condition.checkEffective()) {
if (condition instanceof Brackets) {
List childQueryWrapper = getChildQueryWrapper(((Brackets) condition).getChildCondition());
if (!childQueryWrapper.isEmpty()) {
if (list == null) {
list = new ArrayList<>();
}
list.addAll(childQueryWrapper);
}
}
// not Brackets
else {
Object value = condition.getValue();
if (value instanceof QueryWrapper) {
if (list == null) {
list = new ArrayList<>();
}
list.add((QueryWrapper) value);
list.addAll(((QueryWrapper) value).getChildSelect());
} else if (value != null && value.getClass().isArray()) {
for (int i = 0; i < Array.getLength(value); i++) {
Object arrayValue = Array.get(value, i);
if (arrayValue instanceof QueryWrapper) {
if (list == null) {
list = new ArrayList<>();
}
list.add((QueryWrapper) arrayValue);
list.addAll(((QueryWrapper) arrayValue).getChildSelect());
}
}
}
}
}
condition = condition.next;
}
return list == null ? Collections.emptyList() : list;
}
static Object[] getValues(QueryCondition condition) {
if (condition == null) {
return FlexConsts.EMPTY_ARRAY;
}
List