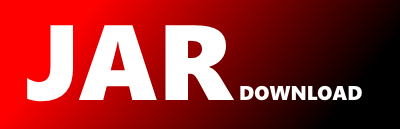
com.mycila.ujd.api.UJD Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mycila-ujd Show documentation
Show all versions of mycila-ujd Show documentation
Mycila Unecessary Jar Detector
The newest version!
/**
* Copyright (C) 2010 Mathieu Carbou
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.mycila.ujd.api;
import com.google.common.base.Function;
import com.google.common.base.Predicate;
import java.util.Collection;
/**
* @author Mathieu Carbou ([email protected])
*/
public final class UJD {
private UJD() {
}
public static final Function, Loader> JAVACLASS_TO_LOADER
= new Function, Loader>() {
public Loader apply(JavaClass> from) {
return from.getLoader();
}
};
public static final Function> LOADER_TO_CONTAINER
= new Function>() {
public Iterable extends Container> apply(Loader from) {
return from.getContainers();
}
};
public static final Function> CONTAINER_TO_CONTAINED_CLASSES
= new Function>() {
public Iterable extends ContainedClass> apply(Container from) {
return from.getClasses();
}
};
public static final Function super ContainedClass, Container> CONTAINED_CLASS_TO_CONTAINER
= new Function() {
public Container apply(ContainedClass from) {
return from.getContainer();
}
};
public static final Function, String> CONTAINED_CLASS_NAME
= new Function, String>() {
public String apply(ContainedJavaClass> from) {
return from.getClassName();
}
};
public static final Function LOADER_NAME = new Function() {
public String apply(Loader loader) {
return loader.getName();
}
};
public static Predicate hasParentLoader(final Loader loader) {
return new Predicate() {
public boolean apply(Loader input) {
return input.getParent() == loader;
}
};
}
public static Predicate> hasLoader(final Loader loader) {
return new Predicate>() {
public boolean apply(JavaClass> input) {
return input.getLoader() == loader;
}
};
}
public static Predicate> javaClassStartsWith(final String prefix) {
return new Predicate>() {
public boolean apply(JavaClass> javaClass) {
return javaClass.get().getName().startsWith(prefix);
}
};
}
public static Predicate containedClassStartsWith(final String prefix) {
return new Predicate() {
public boolean apply(ContainedClass containedClass) {
return containedClass.getClassName().startsWith(prefix);
}
};
}
public static Predicate containedClassNameIn(final Collection col) {
return new Predicate() {
public boolean apply(ContainedClass containedClass) {
return col.contains(containedClass.getClassName());
}
};
}
public static Predicate isLoaderNamed(final String loaderName) {
return new Predicate() {
public boolean apply(Loader loader) {
return loader.getName().equals(loaderName);
}
};
}
public static Iterable memoize(Iterable extends T> it) {
return new MemoizingIterable(it);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy