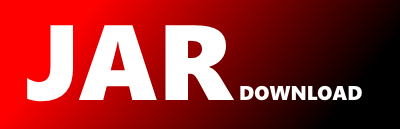
com.mypurecloud.sdk.v2.guest.api.WebChatApiAsync Maven / Gradle / Ivy
The newest version!
package com.mypurecloud.sdk.v2.guest.api;
import com.fasterxml.jackson.core.type.TypeReference;
import com.google.common.util.concurrent.Futures;
import com.google.common.util.concurrent.SettableFuture;
import com.mypurecloud.sdk.v2.guest.AsyncApiCallback;
import com.mypurecloud.sdk.v2.guest.ApiException;
import com.mypurecloud.sdk.v2.guest.ApiClient;
import com.mypurecloud.sdk.v2.guest.ApiRequest;
import com.mypurecloud.sdk.v2.guest.ApiResponse;
import com.mypurecloud.sdk.v2.guest.Configuration;
import com.mypurecloud.sdk.v2.guest.model.*;
import com.mypurecloud.sdk.v2.guest.Pair;
import com.mypurecloud.sdk.v2.guest.model.CreateWebChatConversationRequest;
import com.mypurecloud.sdk.v2.guest.model.CreateWebChatConversationResponse;
import com.mypurecloud.sdk.v2.guest.model.CreateWebChatMessageRequest;
import com.mypurecloud.sdk.v2.guest.model.ErrorBody;
import com.mypurecloud.sdk.v2.guest.model.WebChatGuestMediaRequest;
import com.mypurecloud.sdk.v2.guest.model.WebChatGuestMediaRequestEntityList;
import com.mypurecloud.sdk.v2.guest.model.WebChatMemberInfo;
import com.mypurecloud.sdk.v2.guest.model.WebChatMemberInfoEntityList;
import com.mypurecloud.sdk.v2.guest.model.WebChatMessage;
import com.mypurecloud.sdk.v2.guest.model.WebChatMessageEntityList;
import com.mypurecloud.sdk.v2.guest.model.WebChatTyping;
import com.mypurecloud.sdk.v2.guest.api.request.DeleteWebchatGuestConversationMemberRequest;
import com.mypurecloud.sdk.v2.guest.api.request.GetWebchatGuestConversationMediarequestRequest;
import com.mypurecloud.sdk.v2.guest.api.request.GetWebchatGuestConversationMediarequestsRequest;
import com.mypurecloud.sdk.v2.guest.api.request.GetWebchatGuestConversationMemberRequest;
import com.mypurecloud.sdk.v2.guest.api.request.GetWebchatGuestConversationMembersRequest;
import com.mypurecloud.sdk.v2.guest.api.request.GetWebchatGuestConversationMessageRequest;
import com.mypurecloud.sdk.v2.guest.api.request.GetWebchatGuestConversationMessagesRequest;
import com.mypurecloud.sdk.v2.guest.api.request.PatchWebchatGuestConversationMediarequestRequest;
import com.mypurecloud.sdk.v2.guest.api.request.PostWebchatGuestConversationMemberMessagesRequest;
import com.mypurecloud.sdk.v2.guest.api.request.PostWebchatGuestConversationMemberTypingRequest;
import com.mypurecloud.sdk.v2.guest.api.request.PostWebchatGuestConversationsRequest;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.concurrent.Future;
public class WebChatApiAsync {
private final ApiClient pcapiClient;
public WebChatApiAsync() {
this(Configuration.getDefaultApiClient());
}
public WebChatApiAsync(ApiClient apiClient) {
this.pcapiClient = apiClient;
}
/**
* Remove a member from a chat conversation
*
* @param request the request object
* @param callback the action to perform when the request is completed
* @return the future indication when the request has completed
*/
public Future deleteWebchatGuestConversationMemberAsync(DeleteWebchatGuestConversationMemberRequest request, final AsyncApiCallback callback) {
try {
final SettableFuture future = SettableFuture.create();
final boolean shouldThrowErrors = pcapiClient.getShouldThrowErrors();
pcapiClient.invokeAsync(request.withHttpInfo(), null, new AsyncApiCallback>() {
@Override
public void onCompleted(ApiResponse response) {
notifySuccess(future, callback, response.getBody());
}
@Override
public void onFailed(Throwable exception) {
if (shouldThrowErrors) {
notifyFailure(future, callback, exception);
}
else {
notifySuccess(future, callback, null);
}
}
});
return future;
}
catch (Throwable exception) {
return Futures.immediateFailedFuture(exception);
}
}
/**
* Remove a member from a chat conversation
*
* @param request the request object
* @param callback the action to perform when the request is completed
* @return the future indication when the request has completed
*/
public Future> deleteWebchatGuestConversationMemberAsync(ApiRequest request, final AsyncApiCallback> callback) {
try {
final SettableFuture> future = SettableFuture.create();
final boolean shouldThrowErrors = pcapiClient.getShouldThrowErrors();
pcapiClient.invokeAsync(request, null, new AsyncApiCallback>() {
@Override
public void onCompleted(ApiResponse response) {
notifySuccess(future, callback, response);
}
@Override
public void onFailed(Throwable exception) {
if (exception instanceof ApiException) {
@SuppressWarnings("unchecked")
ApiResponse response = (ApiResponse)(ApiResponse>)exception;
notifySuccess(future, callback, response);
}
if (shouldThrowErrors) {
notifyFailure(future, callback, exception);
}
else {
@SuppressWarnings("unchecked")
ApiResponse response = (ApiResponse)(ApiResponse>)(new ApiException(exception));
notifySuccess(future, callback, response);
}
}
});
return future;
}
catch (Throwable exception) {
return Futures.immediateFailedFuture(exception);
}
}
/**
* Get a media request in the conversation
* This endpoint is deprecated. Please see the article https://help.mypurecloud.com/articles/deprecation-legacy-co-browse-and-screenshare/
* @param request the request object
* @param callback the action to perform when the request is completed
* @return the future indication when the request has completed
*/
public Future getWebchatGuestConversationMediarequestAsync(GetWebchatGuestConversationMediarequestRequest request, final AsyncApiCallback callback) {
try {
final SettableFuture future = SettableFuture.create();
final boolean shouldThrowErrors = pcapiClient.getShouldThrowErrors();
pcapiClient.invokeAsync(request.withHttpInfo(), new TypeReference() {}, new AsyncApiCallback>() {
@Override
public void onCompleted(ApiResponse response) {
notifySuccess(future, callback, response.getBody());
}
@Override
public void onFailed(Throwable exception) {
if (shouldThrowErrors) {
notifyFailure(future, callback, exception);
}
else {
notifySuccess(future, callback, null);
}
}
});
return future;
}
catch (Throwable exception) {
return Futures.immediateFailedFuture(exception);
}
}
/**
* Get a media request in the conversation
* This endpoint is deprecated. Please see the article https://help.mypurecloud.com/articles/deprecation-legacy-co-browse-and-screenshare/
* @param request the request object
* @param callback the action to perform when the request is completed
* @return the future indication when the request has completed
*/
public Future> getWebchatGuestConversationMediarequestAsync(ApiRequest request, final AsyncApiCallback> callback) {
try {
final SettableFuture> future = SettableFuture.create();
final boolean shouldThrowErrors = pcapiClient.getShouldThrowErrors();
pcapiClient.invokeAsync(request, new TypeReference() {}, new AsyncApiCallback>() {
@Override
public void onCompleted(ApiResponse response) {
notifySuccess(future, callback, response);
}
@Override
public void onFailed(Throwable exception) {
if (exception instanceof ApiException) {
@SuppressWarnings("unchecked")
ApiResponse response = (ApiResponse)(ApiResponse>)exception;
notifySuccess(future, callback, response);
}
if (shouldThrowErrors) {
notifyFailure(future, callback, exception);
}
else {
@SuppressWarnings("unchecked")
ApiResponse response = (ApiResponse)(ApiResponse>)(new ApiException(exception));
notifySuccess(future, callback, response);
}
}
});
return future;
}
catch (Throwable exception) {
return Futures.immediateFailedFuture(exception);
}
}
/**
* Get all media requests to the guest in the conversation
*
* @param request the request object
* @param callback the action to perform when the request is completed
* @return the future indication when the request has completed
*/
public Future getWebchatGuestConversationMediarequestsAsync(GetWebchatGuestConversationMediarequestsRequest request, final AsyncApiCallback callback) {
try {
final SettableFuture future = SettableFuture.create();
final boolean shouldThrowErrors = pcapiClient.getShouldThrowErrors();
pcapiClient.invokeAsync(request.withHttpInfo(), new TypeReference() {}, new AsyncApiCallback>() {
@Override
public void onCompleted(ApiResponse response) {
notifySuccess(future, callback, response.getBody());
}
@Override
public void onFailed(Throwable exception) {
if (shouldThrowErrors) {
notifyFailure(future, callback, exception);
}
else {
notifySuccess(future, callback, null);
}
}
});
return future;
}
catch (Throwable exception) {
return Futures.immediateFailedFuture(exception);
}
}
/**
* Get all media requests to the guest in the conversation
*
* @param request the request object
* @param callback the action to perform when the request is completed
* @return the future indication when the request has completed
*/
public Future> getWebchatGuestConversationMediarequestsAsync(ApiRequest request, final AsyncApiCallback> callback) {
try {
final SettableFuture> future = SettableFuture.create();
final boolean shouldThrowErrors = pcapiClient.getShouldThrowErrors();
pcapiClient.invokeAsync(request, new TypeReference() {}, new AsyncApiCallback>() {
@Override
public void onCompleted(ApiResponse response) {
notifySuccess(future, callback, response);
}
@Override
public void onFailed(Throwable exception) {
if (exception instanceof ApiException) {
@SuppressWarnings("unchecked")
ApiResponse response = (ApiResponse)(ApiResponse>)exception;
notifySuccess(future, callback, response);
}
if (shouldThrowErrors) {
notifyFailure(future, callback, exception);
}
else {
@SuppressWarnings("unchecked")
ApiResponse response = (ApiResponse)(ApiResponse>)(new ApiException(exception));
notifySuccess(future, callback, response);
}
}
});
return future;
}
catch (Throwable exception) {
return Futures.immediateFailedFuture(exception);
}
}
/**
* Get a web chat conversation member
*
* @param request the request object
* @param callback the action to perform when the request is completed
* @return the future indication when the request has completed
*/
public Future getWebchatGuestConversationMemberAsync(GetWebchatGuestConversationMemberRequest request, final AsyncApiCallback callback) {
try {
final SettableFuture future = SettableFuture.create();
final boolean shouldThrowErrors = pcapiClient.getShouldThrowErrors();
pcapiClient.invokeAsync(request.withHttpInfo(), new TypeReference() {}, new AsyncApiCallback>() {
@Override
public void onCompleted(ApiResponse response) {
notifySuccess(future, callback, response.getBody());
}
@Override
public void onFailed(Throwable exception) {
if (shouldThrowErrors) {
notifyFailure(future, callback, exception);
}
else {
notifySuccess(future, callback, null);
}
}
});
return future;
}
catch (Throwable exception) {
return Futures.immediateFailedFuture(exception);
}
}
/**
* Get a web chat conversation member
*
* @param request the request object
* @param callback the action to perform when the request is completed
* @return the future indication when the request has completed
*/
public Future> getWebchatGuestConversationMemberAsync(ApiRequest request, final AsyncApiCallback> callback) {
try {
final SettableFuture> future = SettableFuture.create();
final boolean shouldThrowErrors = pcapiClient.getShouldThrowErrors();
pcapiClient.invokeAsync(request, new TypeReference() {}, new AsyncApiCallback>() {
@Override
public void onCompleted(ApiResponse response) {
notifySuccess(future, callback, response);
}
@Override
public void onFailed(Throwable exception) {
if (exception instanceof ApiException) {
@SuppressWarnings("unchecked")
ApiResponse response = (ApiResponse)(ApiResponse>)exception;
notifySuccess(future, callback, response);
}
if (shouldThrowErrors) {
notifyFailure(future, callback, exception);
}
else {
@SuppressWarnings("unchecked")
ApiResponse response = (ApiResponse)(ApiResponse>)(new ApiException(exception));
notifySuccess(future, callback, response);
}
}
});
return future;
}
catch (Throwable exception) {
return Futures.immediateFailedFuture(exception);
}
}
/**
* Get the members of a chat conversation.
*
* @param request the request object
* @param callback the action to perform when the request is completed
* @return the future indication when the request has completed
*/
public Future getWebchatGuestConversationMembersAsync(GetWebchatGuestConversationMembersRequest request, final AsyncApiCallback callback) {
try {
final SettableFuture future = SettableFuture.create();
final boolean shouldThrowErrors = pcapiClient.getShouldThrowErrors();
pcapiClient.invokeAsync(request.withHttpInfo(), new TypeReference() {}, new AsyncApiCallback>() {
@Override
public void onCompleted(ApiResponse response) {
notifySuccess(future, callback, response.getBody());
}
@Override
public void onFailed(Throwable exception) {
if (shouldThrowErrors) {
notifyFailure(future, callback, exception);
}
else {
notifySuccess(future, callback, null);
}
}
});
return future;
}
catch (Throwable exception) {
return Futures.immediateFailedFuture(exception);
}
}
/**
* Get the members of a chat conversation.
*
* @param request the request object
* @param callback the action to perform when the request is completed
* @return the future indication when the request has completed
*/
public Future> getWebchatGuestConversationMembersAsync(ApiRequest request, final AsyncApiCallback> callback) {
try {
final SettableFuture> future = SettableFuture.create();
final boolean shouldThrowErrors = pcapiClient.getShouldThrowErrors();
pcapiClient.invokeAsync(request, new TypeReference() {}, new AsyncApiCallback>() {
@Override
public void onCompleted(ApiResponse response) {
notifySuccess(future, callback, response);
}
@Override
public void onFailed(Throwable exception) {
if (exception instanceof ApiException) {
@SuppressWarnings("unchecked")
ApiResponse response = (ApiResponse)(ApiResponse>)exception;
notifySuccess(future, callback, response);
}
if (shouldThrowErrors) {
notifyFailure(future, callback, exception);
}
else {
@SuppressWarnings("unchecked")
ApiResponse response = (ApiResponse)(ApiResponse>)(new ApiException(exception));
notifySuccess(future, callback, response);
}
}
});
return future;
}
catch (Throwable exception) {
return Futures.immediateFailedFuture(exception);
}
}
/**
* Get a web chat conversation message
*
* @param request the request object
* @param callback the action to perform when the request is completed
* @return the future indication when the request has completed
*/
public Future getWebchatGuestConversationMessageAsync(GetWebchatGuestConversationMessageRequest request, final AsyncApiCallback callback) {
try {
final SettableFuture future = SettableFuture.create();
final boolean shouldThrowErrors = pcapiClient.getShouldThrowErrors();
pcapiClient.invokeAsync(request.withHttpInfo(), new TypeReference() {}, new AsyncApiCallback>() {
@Override
public void onCompleted(ApiResponse response) {
notifySuccess(future, callback, response.getBody());
}
@Override
public void onFailed(Throwable exception) {
if (shouldThrowErrors) {
notifyFailure(future, callback, exception);
}
else {
notifySuccess(future, callback, null);
}
}
});
return future;
}
catch (Throwable exception) {
return Futures.immediateFailedFuture(exception);
}
}
/**
* Get a web chat conversation message
*
* @param request the request object
* @param callback the action to perform when the request is completed
* @return the future indication when the request has completed
*/
public Future> getWebchatGuestConversationMessageAsync(ApiRequest request, final AsyncApiCallback> callback) {
try {
final SettableFuture> future = SettableFuture.create();
final boolean shouldThrowErrors = pcapiClient.getShouldThrowErrors();
pcapiClient.invokeAsync(request, new TypeReference() {}, new AsyncApiCallback>() {
@Override
public void onCompleted(ApiResponse response) {
notifySuccess(future, callback, response);
}
@Override
public void onFailed(Throwable exception) {
if (exception instanceof ApiException) {
@SuppressWarnings("unchecked")
ApiResponse response = (ApiResponse)(ApiResponse>)exception;
notifySuccess(future, callback, response);
}
if (shouldThrowErrors) {
notifyFailure(future, callback, exception);
}
else {
@SuppressWarnings("unchecked")
ApiResponse response = (ApiResponse)(ApiResponse>)(new ApiException(exception));
notifySuccess(future, callback, response);
}
}
});
return future;
}
catch (Throwable exception) {
return Futures.immediateFailedFuture(exception);
}
}
/**
* Get the messages of a chat conversation.
*
* @param request the request object
* @param callback the action to perform when the request is completed
* @return the future indication when the request has completed
*/
public Future getWebchatGuestConversationMessagesAsync(GetWebchatGuestConversationMessagesRequest request, final AsyncApiCallback callback) {
try {
final SettableFuture future = SettableFuture.create();
final boolean shouldThrowErrors = pcapiClient.getShouldThrowErrors();
pcapiClient.invokeAsync(request.withHttpInfo(), new TypeReference() {}, new AsyncApiCallback>() {
@Override
public void onCompleted(ApiResponse response) {
notifySuccess(future, callback, response.getBody());
}
@Override
public void onFailed(Throwable exception) {
if (shouldThrowErrors) {
notifyFailure(future, callback, exception);
}
else {
notifySuccess(future, callback, null);
}
}
});
return future;
}
catch (Throwable exception) {
return Futures.immediateFailedFuture(exception);
}
}
/**
* Get the messages of a chat conversation.
*
* @param request the request object
* @param callback the action to perform when the request is completed
* @return the future indication when the request has completed
*/
public Future> getWebchatGuestConversationMessagesAsync(ApiRequest request, final AsyncApiCallback> callback) {
try {
final SettableFuture> future = SettableFuture.create();
final boolean shouldThrowErrors = pcapiClient.getShouldThrowErrors();
pcapiClient.invokeAsync(request, new TypeReference() {}, new AsyncApiCallback>() {
@Override
public void onCompleted(ApiResponse response) {
notifySuccess(future, callback, response);
}
@Override
public void onFailed(Throwable exception) {
if (exception instanceof ApiException) {
@SuppressWarnings("unchecked")
ApiResponse response = (ApiResponse)(ApiResponse>)exception;
notifySuccess(future, callback, response);
}
if (shouldThrowErrors) {
notifyFailure(future, callback, exception);
}
else {
@SuppressWarnings("unchecked")
ApiResponse response = (ApiResponse)(ApiResponse>)(new ApiException(exception));
notifySuccess(future, callback, response);
}
}
});
return future;
}
catch (Throwable exception) {
return Futures.immediateFailedFuture(exception);
}
}
/**
* Update a media request in the conversation, setting the state to ACCEPTED/DECLINED/ERRORED
* This endpoint is deprecated. Please see the article https://help.mypurecloud.com/articles/deprecation-legacy-co-browse-and-screenshare/
* @param request the request object
* @param callback the action to perform when the request is completed
* @return the future indication when the request has completed
*/
public Future patchWebchatGuestConversationMediarequestAsync(PatchWebchatGuestConversationMediarequestRequest request, final AsyncApiCallback callback) {
try {
final SettableFuture future = SettableFuture.create();
final boolean shouldThrowErrors = pcapiClient.getShouldThrowErrors();
pcapiClient.invokeAsync(request.withHttpInfo(), new TypeReference() {}, new AsyncApiCallback>() {
@Override
public void onCompleted(ApiResponse response) {
notifySuccess(future, callback, response.getBody());
}
@Override
public void onFailed(Throwable exception) {
if (shouldThrowErrors) {
notifyFailure(future, callback, exception);
}
else {
notifySuccess(future, callback, null);
}
}
});
return future;
}
catch (Throwable exception) {
return Futures.immediateFailedFuture(exception);
}
}
/**
* Update a media request in the conversation, setting the state to ACCEPTED/DECLINED/ERRORED
* This endpoint is deprecated. Please see the article https://help.mypurecloud.com/articles/deprecation-legacy-co-browse-and-screenshare/
* @param request the request object
* @param callback the action to perform when the request is completed
* @return the future indication when the request has completed
*/
public Future> patchWebchatGuestConversationMediarequestAsync(ApiRequest request, final AsyncApiCallback> callback) {
try {
final SettableFuture> future = SettableFuture.create();
final boolean shouldThrowErrors = pcapiClient.getShouldThrowErrors();
pcapiClient.invokeAsync(request, new TypeReference() {}, new AsyncApiCallback>() {
@Override
public void onCompleted(ApiResponse response) {
notifySuccess(future, callback, response);
}
@Override
public void onFailed(Throwable exception) {
if (exception instanceof ApiException) {
@SuppressWarnings("unchecked")
ApiResponse response = (ApiResponse)(ApiResponse>)exception;
notifySuccess(future, callback, response);
}
if (shouldThrowErrors) {
notifyFailure(future, callback, exception);
}
else {
@SuppressWarnings("unchecked")
ApiResponse response = (ApiResponse)(ApiResponse>)(new ApiException(exception));
notifySuccess(future, callback, response);
}
}
});
return future;
}
catch (Throwable exception) {
return Futures.immediateFailedFuture(exception);
}
}
/**
* Send a message in a chat conversation.
*
* @param request the request object
* @param callback the action to perform when the request is completed
* @return the future indication when the request has completed
*/
public Future postWebchatGuestConversationMemberMessagesAsync(PostWebchatGuestConversationMemberMessagesRequest request, final AsyncApiCallback callback) {
try {
final SettableFuture future = SettableFuture.create();
final boolean shouldThrowErrors = pcapiClient.getShouldThrowErrors();
pcapiClient.invokeAsync(request.withHttpInfo(), new TypeReference() {}, new AsyncApiCallback>() {
@Override
public void onCompleted(ApiResponse response) {
notifySuccess(future, callback, response.getBody());
}
@Override
public void onFailed(Throwable exception) {
if (shouldThrowErrors) {
notifyFailure(future, callback, exception);
}
else {
notifySuccess(future, callback, null);
}
}
});
return future;
}
catch (Throwable exception) {
return Futures.immediateFailedFuture(exception);
}
}
/**
* Send a message in a chat conversation.
*
* @param request the request object
* @param callback the action to perform when the request is completed
* @return the future indication when the request has completed
*/
public Future> postWebchatGuestConversationMemberMessagesAsync(ApiRequest request, final AsyncApiCallback> callback) {
try {
final SettableFuture> future = SettableFuture.create();
final boolean shouldThrowErrors = pcapiClient.getShouldThrowErrors();
pcapiClient.invokeAsync(request, new TypeReference() {}, new AsyncApiCallback>() {
@Override
public void onCompleted(ApiResponse response) {
notifySuccess(future, callback, response);
}
@Override
public void onFailed(Throwable exception) {
if (exception instanceof ApiException) {
@SuppressWarnings("unchecked")
ApiResponse response = (ApiResponse)(ApiResponse>)exception;
notifySuccess(future, callback, response);
}
if (shouldThrowErrors) {
notifyFailure(future, callback, exception);
}
else {
@SuppressWarnings("unchecked")
ApiResponse response = (ApiResponse)(ApiResponse>)(new ApiException(exception));
notifySuccess(future, callback, response);
}
}
});
return future;
}
catch (Throwable exception) {
return Futures.immediateFailedFuture(exception);
}
}
/**
* Send a typing-indicator in a chat conversation.
*
* @param request the request object
* @param callback the action to perform when the request is completed
* @return the future indication when the request has completed
*/
public Future postWebchatGuestConversationMemberTypingAsync(PostWebchatGuestConversationMemberTypingRequest request, final AsyncApiCallback callback) {
try {
final SettableFuture future = SettableFuture.create();
final boolean shouldThrowErrors = pcapiClient.getShouldThrowErrors();
pcapiClient.invokeAsync(request.withHttpInfo(), new TypeReference() {}, new AsyncApiCallback>() {
@Override
public void onCompleted(ApiResponse response) {
notifySuccess(future, callback, response.getBody());
}
@Override
public void onFailed(Throwable exception) {
if (shouldThrowErrors) {
notifyFailure(future, callback, exception);
}
else {
notifySuccess(future, callback, null);
}
}
});
return future;
}
catch (Throwable exception) {
return Futures.immediateFailedFuture(exception);
}
}
/**
* Send a typing-indicator in a chat conversation.
*
* @param request the request object
* @param callback the action to perform when the request is completed
* @return the future indication when the request has completed
*/
public Future> postWebchatGuestConversationMemberTypingAsync(ApiRequest request, final AsyncApiCallback> callback) {
try {
final SettableFuture> future = SettableFuture.create();
final boolean shouldThrowErrors = pcapiClient.getShouldThrowErrors();
pcapiClient.invokeAsync(request, new TypeReference() {}, new AsyncApiCallback>() {
@Override
public void onCompleted(ApiResponse response) {
notifySuccess(future, callback, response);
}
@Override
public void onFailed(Throwable exception) {
if (exception instanceof ApiException) {
@SuppressWarnings("unchecked")
ApiResponse response = (ApiResponse)(ApiResponse>)exception;
notifySuccess(future, callback, response);
}
if (shouldThrowErrors) {
notifyFailure(future, callback, exception);
}
else {
@SuppressWarnings("unchecked")
ApiResponse response = (ApiResponse)(ApiResponse>)(new ApiException(exception));
notifySuccess(future, callback, response);
}
}
});
return future;
}
catch (Throwable exception) {
return Futures.immediateFailedFuture(exception);
}
}
/**
* Create an ACD chat conversation from an external customer.
* This endpoint will create a new ACD Chat conversation under the specified Chat Deployment. The conversation will begin with a guest member in it (with a role=CUSTOMER) according to the customer information that is supplied. If the guest member is authenticated, the 'memberAuthToken' field should include his JWT as generated by the 'POST /api/v2/signeddata' resource; if the guest member is anonymous (and the Deployment permits it) this field can be omitted. The returned data includes the IDs of the conversation created, along with a newly-create JWT token that you can supply to all future endpoints as authentication to perform operations against that conversation. After successfully creating a conversation, you should connect a websocket to the event stream named in the 'eventStreamUri' field of the response; the conversation is not routed until the event stream is attached.
* @param request the request object
* @param callback the action to perform when the request is completed
* @return the future indication when the request has completed
*/
public Future postWebchatGuestConversationsAsync(PostWebchatGuestConversationsRequest request, final AsyncApiCallback callback) {
try {
final SettableFuture future = SettableFuture.create();
final boolean shouldThrowErrors = pcapiClient.getShouldThrowErrors();
pcapiClient.invokeAsync(request.withHttpInfo(), new TypeReference() {}, new AsyncApiCallback>() {
@Override
public void onCompleted(ApiResponse response) {
notifySuccess(future, callback, response.getBody());
}
@Override
public void onFailed(Throwable exception) {
if (shouldThrowErrors) {
notifyFailure(future, callback, exception);
}
else {
notifySuccess(future, callback, null);
}
}
});
return future;
}
catch (Throwable exception) {
return Futures.immediateFailedFuture(exception);
}
}
/**
* Create an ACD chat conversation from an external customer.
* This endpoint will create a new ACD Chat conversation under the specified Chat Deployment. The conversation will begin with a guest member in it (with a role=CUSTOMER) according to the customer information that is supplied. If the guest member is authenticated, the 'memberAuthToken' field should include his JWT as generated by the 'POST /api/v2/signeddata' resource; if the guest member is anonymous (and the Deployment permits it) this field can be omitted. The returned data includes the IDs of the conversation created, along with a newly-create JWT token that you can supply to all future endpoints as authentication to perform operations against that conversation. After successfully creating a conversation, you should connect a websocket to the event stream named in the 'eventStreamUri' field of the response; the conversation is not routed until the event stream is attached.
* @param request the request object
* @param callback the action to perform when the request is completed
* @return the future indication when the request has completed
*/
public Future> postWebchatGuestConversationsAsync(ApiRequest request, final AsyncApiCallback> callback) {
try {
final SettableFuture> future = SettableFuture.create();
final boolean shouldThrowErrors = pcapiClient.getShouldThrowErrors();
pcapiClient.invokeAsync(request, new TypeReference() {}, new AsyncApiCallback>() {
@Override
public void onCompleted(ApiResponse response) {
notifySuccess(future, callback, response);
}
@Override
public void onFailed(Throwable exception) {
if (exception instanceof ApiException) {
@SuppressWarnings("unchecked")
ApiResponse response = (ApiResponse)(ApiResponse>)exception;
notifySuccess(future, callback, response);
}
if (shouldThrowErrors) {
notifyFailure(future, callback, exception);
}
else {
@SuppressWarnings("unchecked")
ApiResponse response = (ApiResponse)(ApiResponse>)(new ApiException(exception));
notifySuccess(future, callback, response);
}
}
});
return future;
}
catch (Throwable exception) {
return Futures.immediateFailedFuture(exception);
}
}
private void notifySuccess(SettableFuture future, AsyncApiCallback callback, T result) {
if (callback != null) {
try {
callback.onCompleted(result);
future.set(result);
}
catch (Throwable exception) {
future.setException(exception);
}
}
else {
future.set(result);
}
}
private void notifyFailure(SettableFuture future, AsyncApiCallback callback, Throwable exception) {
if (callback != null) {
try {
callback.onFailed(exception);
future.setException(exception);
}
catch (Throwable callbackException) {
future.setException(callbackException);
}
}
else {
future.setException(exception);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy