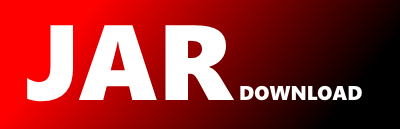
com.mytaxi.apis.phrase.api.GenericPhraseAPI Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of phrase-java-client Show documentation
Show all versions of phrase-java-client Show documentation
This projects contains of services to handle the translations from [PhraseApp API
v2](http://docs.phraseapp.com/api/v2/). It's supposed to expose Phrase translations as POJO or as File within the java world.
package com.mytaxi.apis.phrase.api;
import com.mytaxi.apis.phrase.exception.PhraseAppApiException;
import java.net.URI;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.apache.http.NameValuePair;
import org.apache.http.client.utils.URIBuilder;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpMethod;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.http.converter.ByteArrayHttpMessageConverter;
import org.springframework.http.converter.HttpMessageConverter;
import org.springframework.http.converter.json.MappingJackson2HttpMessageConverter;
import org.springframework.web.client.RestTemplate;
/**
* Created by m.winkelmann on 05.11.15.
*/
public class GenericPhraseAPI
{
private static final Logger LOG = LoggerFactory.getLogger(GenericPhraseAPI.class);
private static final String PHRASE_SCHEME = "https";
private static final String PHRASE_HOST = "api.phraseapp.com";
// --- internal services ---
protected final RestTemplate restTemplate;
protected final String authToken;
private final Map pathToETagCache = new HashMap();
private final Map pathToResponseCache = new HashMap();
public GenericPhraseAPI(final RestTemplate restTemplate, final String authToken)
{
this.restTemplate = restTemplate;
this.authToken = authToken;
}
protected static RestTemplate createRestTemplateWithConverter()
{
final RestTemplate restTemplate = new RestTemplate();
final List> httpMessageConverters = new ArrayList>();
httpMessageConverters.add(new MappingJackson2HttpMessageConverter());
httpMessageConverters.add(new ByteArrayHttpMessageConverter());
restTemplate.setMessageConverters(httpMessageConverters);
return restTemplate;
}
protected String createPath(final String path, final Map placeholders)
{
// TODO with URIBuilder or directly in createUriBuilder()
String requestPath = new String(path);
for (final Map.Entry entity : placeholders.entrySet())
{
final String value = entity.getValue();
final String key = entity.getKey();
requestPath = requestPath.replace(key, value);
}
return requestPath;
}
protected URIBuilder createUriBuilder(final String path)
{
return createUriBuilder(path, null);
}
protected URIBuilder createUriBuilder(final String path, final List parameters)
{
final URIBuilder builder = new URIBuilder();
builder.setScheme(PHRASE_SCHEME)
.setHost(PHRASE_HOST)
.setPath(path);
if (parameters != null)
{
builder.setParameters(parameters);
}
return builder;
}
protected HttpEntity
© 2015 - 2025 Weber Informatics LLC | Privacy Policy