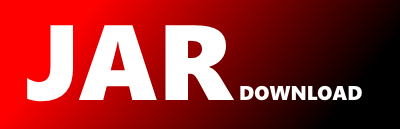
com.mzlion.easyokhttp.request.BinaryBodyPostRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of easy-okhttp Show documentation
Show all versions of easy-okhttp Show documentation
easy-okhttp是对okhttp3上层封装的网络框架,支持文件上传和下载表单提交(文件和一个参数对应多值),
链式调用,并且默认整合Gson,对返回结果多种转换,同时还支持HTTPS单向认证和双向认证等特性。
/*
* Copyright (C) 2016 mzlion([email protected]).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.mzlion.easyokhttp.request;
import com.mzlion.core.http.ContentType;
import com.mzlion.core.io.IOUtils;
import com.mzlion.core.lang.Assert;
import com.mzlion.easyokhttp.utils.MediaTypeUtils;
import okhttp3.MediaType;
import okhttp3.Request;
import okhttp3.RequestBody;
import java.io.*;
/**
*
* 2016-05-15 22:33 POST提交二进制流,服务端应该从Request请求体获取二进制流。
*
*
* @author mzlion
*/
public class BinaryBodyPostRequest extends AbstractHttpRequest {
/**
* 二进制流内容
*/
private byte[] content;
private MediaType mediaType;
/**
* 默认构造器
*
* @param url 请求地址
*/
public BinaryBodyPostRequest(String url) {
super(url);
}
/**
* 设置二进制流
*
* @param inputStream 二进制流
* @return {@link BinaryBodyPostRequest}
* @see MediaType
*/
public BinaryBodyPostRequest stream(InputStream inputStream) {
Assert.notNull(inputStream, "In must not be null.");
Assert.notNull(mediaType, "MediaType must not be null.");
try (ByteArrayOutputStream outputStream = new ByteArrayOutputStream()) {
if (IOUtils.copy(inputStream, outputStream) == -1) {
throw new IOException("Copy failed");
}
this.content = outputStream.toByteArray();
} catch (IOException e) {
throw new RuntimeException("Reading stream failed->", e);
} finally {
IOUtils.closeQuietly(inputStream);
}
return this;
}
/**
* 设置文件,转为文件流
*
* @param file 文件对象
* @return {@link BinaryBodyPostRequest}
*/
public BinaryBodyPostRequest file(File file) {
Assert.notNull(file, "File must not be null.");
String filename = file.getName();
MediaType mediaType = MediaTypeUtils.parse(filename);
try {
this.stream(new FileInputStream(file));
this.mediaType = mediaType;
return this;
} catch (FileNotFoundException e) {
throw new RuntimeException(e);
}
}
/**
* 设置请求内容类型
*
* @param contentType 请求内容类型
* @return {@link BinaryBodyPostRequest}
*/
public BinaryBodyPostRequest contentType(String contentType) {
Assert.hasLength(contentType, "ContentType must not be null.");
this.mediaType = MediaType.parse(contentType);
return this;
}
/**
* 设置请求内容类型
*
* @param contentType 请求内容类型
* @return {@link BinaryBodyPostRequest}
*/
public BinaryBodyPostRequest contentType(ContentType contentType) {
Assert.notNull(contentType, "ContentType must not be null.");
this.mediaType = MediaType.parse(contentType.toString());
return this;
}
/**
* 获取{@linkplain RequestBody}对象
*/
@Override
protected RequestBody generateRequestBody() {
return RequestBody.create(this.mediaType, this.content);
}
/**
* 根据不同的请求方式,将RequestBody转换成Request对象
*
* @param requestBody 请求体
* @return {@link Request}
* @see RequestBody
*/
@Override
protected Request generateRequest(RequestBody requestBody) {
Request.Builder builder = new Request.Builder();
builder.headers(super.buildHeaders());
return builder.url(this.buildUrl()).post(requestBody).build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy