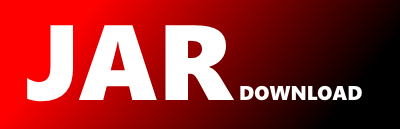
com.mzlion.easyokhttp.request.TextBodyRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of easy-okhttp Show documentation
Show all versions of easy-okhttp Show documentation
easy-okhttp是对okhttp3上层封装的网络框架,支持文件上传和下载表单提交(文件和一个参数对应多值),
链式调用,并且默认整合Gson,对返回结果多种转换,同时还支持HTTPS单向认证和双向认证等特性。
/*
* Copyright (C) 2016 mzlion([email protected]).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.mzlion.easyokhttp.request;
import com.mzlion.core.json.gson.JsonUtil;
import com.mzlion.core.lang.Assert;
import okhttp3.MediaType;
import okhttp3.Request;
import okhttp3.RequestBody;
import java.nio.charset.Charset;
import java.nio.charset.StandardCharsets;
/**
*
* 提交文本字符串,服务端应该从Request请求体获取文本字符串。
*
*
* @author mzlion
*/
public class TextBodyRequest extends AbstractHttpRequest {
private static final MediaType TEXT_LAIN = MediaType.parse("text/plain");
private static final MediaType JSON = MediaType.parse("application/json");
private static final MediaType JAVASCRIPT = MediaType.parse("application/javascript");
private static final MediaType XML = MediaType.parse("application/xml");
private static final MediaType HTML = MediaType.parse("application/html");
private String content;
private Charset charset;
private MediaType mediaType;
/**
* 默认构造器
*
* @param url 请求地址
*/
public TextBodyRequest(String url) {
super(url);
this.charset = StandardCharsets.UTF_8;
}
/**
* POST提交一段j文本内容
*
* @param text 文本字符串
* @return {@link TextBodyRequest}
*/
public TextBodyRequest text(String text) {
Assert.hasLength(text, "Text must not be null.");
this.content = text;
this.mediaType = TEXT_LAIN;
return this;
}
/**
* POST提交一段json字符串
*
* @param json json字符串
* @return {@link TextBodyRequest}
*/
public TextBodyRequest json(String json) {
Assert.hasLength(json, "Json must not be null.");
this.content = json;
this.mediaType = JSON;
return this;
}
/**
* POST提交一段json字符串
*
* @param value Java对象
* @return {@link TextBodyRequest}
*/
public TextBodyRequest json(Object value) {
Assert.notNull(value, "Value must not be null.");
this.content = JsonUtil.toJson(value);
this.mediaType = JSON;
return this;
}
/**
* POST提交一段xml代码
*
* @param xml xml字符串
* @return {@link TextBodyRequest}
*/
public TextBodyRequest xml(String xml) {
Assert.hasLength(xml, "Xml must not be null.");
this.content = xml;
this.mediaType = XML;
return this;
}
/**
* POST提交一段html代码
*
* @param html html字符串
* @return {@link TextBodyRequest}
*/
public TextBodyRequest html(String html) {
Assert.hasLength(html, "Html must not be null.");
this.content = html;
this.mediaType = HTML;
return this;
}
/**
* POST提交一段javascript代码
*
* @param javascript 字符串
* @return {@link TextBodyRequest}
*/
public TextBodyRequest javascript(String javascript) {
Assert.hasLength(javascript, "Javascript must not be null.");
this.content = javascript;
this.mediaType = JAVASCRIPT;
return this;
}
/**
* 设置字符集
*
* @param charset 字符编码
* @return {@link TextBodyRequest}
*/
public TextBodyRequest charset(String charset) {
Assert.hasLength(charset, "Charset must not be null.");
this.charset = Charset.forName(charset);
return this;
}
/**
* 获取{@linkplain RequestBody}对象
*/
@Override
protected RequestBody generateRequestBody() {
MediaType contentType = MediaType.parse(String.format("%s;%s", this.mediaType.toString(), this.charset == null ? StandardCharsets.UTF_8.toString() : this.charset.toString()));
return RequestBody.create(contentType, this.content);
}
/**
* 根据不同的请求方式,将RequestBody转换成Request对象
*
* @param requestBody 请求体
* @return {@link Request}
* @see RequestBody
*/
@Override
protected Request generateRequest(RequestBody requestBody) {
Request.Builder builder = new Request.Builder();
builder.url(this.buildUrl()).headers(super.buildHeaders())
.post(requestBody);
return builder.build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy