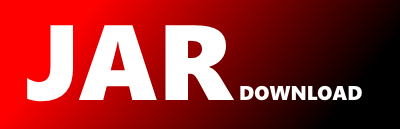
io.druid.curator.discovery.DiscoveryModule Maven / Gradle / Ivy
The newest version!
/*
* Druid - a distributed column store.
* Copyright (C) 2012, 2013 Metamarkets Group Inc.
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU General Public License
* as published by the Free Software Foundation; either version 2
* of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
*/
package io.druid.curator.discovery;
import com.google.common.base.Throwables;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.Lists;
import com.google.inject.Binder;
import com.google.inject.Injector;
import com.google.inject.Key;
import com.google.inject.Module;
import com.google.inject.Provides;
import com.google.inject.TypeLiteral;
import com.google.inject.name.Named;
import com.google.inject.name.Names;
import com.metamx.common.lifecycle.Lifecycle;
import io.druid.guice.DruidBinders;
import io.druid.guice.JsonConfigProvider;
import io.druid.guice.KeyHolder;
import io.druid.guice.LazySingleton;
import io.druid.guice.LifecycleModule;
import io.druid.guice.annotations.Self;
import io.druid.server.DruidNode;
import io.druid.server.initialization.CuratorDiscoveryConfig;
import org.apache.curator.framework.CuratorFramework;
import org.apache.curator.x.discovery.*;
import org.apache.curator.x.discovery.details.ServiceCacheListener;
import java.io.IOException;
import java.lang.annotation.Annotation;
import java.util.Collection;
import java.util.List;
import java.util.Set;
import java.util.concurrent.Executor;
import java.util.concurrent.ThreadFactory;
/**
* The DiscoveryModule allows for the registration of Keys of DruidNode objects, which it intends to be
* automatically announced at the end of the lifecycle start.
*
* In order for this to work a ServiceAnnouncer instance *must* be injected and instantiated first.
* This can often be achieved by registering ServiceAnnouncer.class with the LifecycleModule.
*/
public class DiscoveryModule implements Module
{
private static final String NAME = "DiscoveryModule:internal";
/**
* Requests that the un-annotated DruidNode instance be injected and published as part of the lifecycle.
*
* That is, this module will announce the DruidNode instance returned by
* injector.getInstance(Key.get(DruidNode.class)) automatically.
* Announcement will happen in the LAST stage of the Lifecycle
*/
public static void registerDefault(Binder binder)
{
registerKey(binder, Key.get(new TypeLiteral(){}));
}
/**
* Requests that the annotated DruidNode instance be injected and published as part of the lifecycle.
*
* That is, this module will announce the DruidNode instance returned by
* injector.getInstance(Key.get(DruidNode.class, annotation)) automatically.
* Announcement will happen in the LAST stage of the Lifecycle
*
* @param annotation The annotation instance to use in finding the DruidNode instance, usually a Named annotation
*/
public static void register(Binder binder, Annotation annotation)
{
registerKey(binder, Key.get(new TypeLiteral(){}, annotation));
}
/**
* Requests that the annotated DruidNode instance be injected and published as part of the lifecycle.
*
* That is, this module will announce the DruidNode instance returned by
* injector.getInstance(Key.get(DruidNode.class, annotation)) automatically.
* Announcement will happen in the LAST stage of the Lifecycle
*
* @param annotation The annotation class to use in finding the DruidNode instance
*/
public static void register(Binder binder, Class extends Annotation> annotation)
{
registerKey(binder, Key.get(new TypeLiteral(){}, annotation));
}
/**
* Requests that the keyed DruidNode instance be injected and published as part of the lifecycle.
*
* That is, this module will announce the DruidNode instance returned by
* injector.getInstance(Key.get(DruidNode.class, annotation)) automatically.
* Announcement will happen in the LAST stage of the Lifecycle
*
* @param key The key to use in finding the DruidNode instance
*/
public static void registerKey(Binder binder, Key key)
{
DruidBinders.discoveryAnnouncementBinder(binder).addBinding().toInstance(new KeyHolder<>(key));
LifecycleModule.register(binder, ServiceAnnouncer.class);
}
@Override
public void configure(Binder binder)
{
JsonConfigProvider.bind(binder, "druid.discovery.curator", CuratorDiscoveryConfig.class);
binder.bind(CuratorServiceAnnouncer.class).in(LazySingleton.class);
// Build the binder so that it will at a minimum inject an empty set.
DruidBinders.discoveryAnnouncementBinder(binder);
binder.bind(ServiceAnnouncer.class)
.to(Key.get(CuratorServiceAnnouncer.class, Names.named(NAME)))
.in(LazySingleton.class);
}
@Provides
@LazySingleton
@Named(NAME)
public CuratorServiceAnnouncer getServiceAnnouncer(
final CuratorServiceAnnouncer announcer,
final Injector injector,
final Set> nodesToAnnounce,
final Lifecycle lifecycle
) throws Exception
{
lifecycle.addMaybeStartHandler(
new Lifecycle.Handler()
{
private volatile List nodes = null;
@Override
public void start() throws Exception
{
if (nodes == null) {
nodes = Lists.newArrayList();
for (KeyHolder holder : nodesToAnnounce) {
nodes.add(injector.getInstance(holder.getKey()));
}
}
for (DruidNode node : nodes) {
announcer.announce(node);
}
}
@Override
public void stop()
{
if (nodes != null) {
for (DruidNode node : nodes) {
announcer.unannounce(node);
}
}
}
},
Lifecycle.Stage.LAST
);
return announcer;
}
@Provides
@LazySingleton
public ServiceDiscovery getServiceDiscovery(
CuratorFramework curator,
CuratorDiscoveryConfig config,
Lifecycle lifecycle
) throws Exception
{
if (!config.useDiscovery()) {
return new NoopServiceDiscovery<>();
}
final ServiceDiscovery serviceDiscovery =
ServiceDiscoveryBuilder.builder(Void.class)
.basePath(config.getPath())
.client(curator)
.build();
lifecycle.addMaybeStartHandler(
new Lifecycle.Handler()
{
@Override
public void start() throws Exception
{
serviceDiscovery.start();
}
@Override
public void stop()
{
try {
serviceDiscovery.close();
}
catch (Exception e) {
throw Throwables.propagate(e);
}
}
}
);
return serviceDiscovery;
}
@Provides
@LazySingleton
public ServerDiscoveryFactory getServerDiscoveryFactory(
ServiceDiscovery serviceDiscovery
)
{
return new ServerDiscoveryFactory(serviceDiscovery);
}
private static class NoopServiceDiscovery implements ServiceDiscovery
{
@Override
public void start() throws Exception
{
}
@Override
public void registerService(ServiceInstance service) throws Exception
{
}
@Override
public void updateService(ServiceInstance service) throws Exception
{
}
@Override
public void unregisterService(ServiceInstance service) throws Exception
{
}
@Override
public ServiceCacheBuilder serviceCacheBuilder()
{
return new NoopServiceCacheBuilder<>();
}
@Override
public Collection queryForNames() throws Exception
{
return ImmutableList.of();
}
@Override
public Collection> queryForInstances(String name) throws Exception
{
return ImmutableList.of();
}
@Override
public ServiceInstance queryForInstance(String name, String id) throws Exception
{
return null;
}
@Override
public ServiceProviderBuilder serviceProviderBuilder()
{
return new NoopServiceProviderBuilder<>();
}
@Override
public void close() throws IOException
{
}
}
private static class NoopServiceCacheBuilder implements ServiceCacheBuilder
{
@Override
public ServiceCache build()
{
return new NoopServiceCache<>();
}
@Override
public ServiceCacheBuilder name(String name)
{
return this;
}
@Override
public ServiceCacheBuilder threadFactory(ThreadFactory threadFactory)
{
return this;
}
private static class NoopServiceCache implements ServiceCache
{
@Override
public List> getInstances()
{
return ImmutableList.of();
}
@Override
public void start() throws Exception
{
}
@Override
public void close() throws IOException
{
}
@Override
public void addListener(ServiceCacheListener listener)
{
}
@Override
public void addListener(
ServiceCacheListener listener, Executor executor
)
{
}
@Override
public void removeListener(ServiceCacheListener listener)
{
}
}
}
private static class NoopServiceProviderBuilder implements ServiceProviderBuilder
{
@Override
public ServiceProvider build()
{
return new NoopServiceProvider<>();
}
@Override
public ServiceProviderBuilder serviceName(String serviceName)
{
return this;
}
@Override
public ServiceProviderBuilder providerStrategy(ProviderStrategy providerStrategy)
{
return this;
}
@Override
public ServiceProviderBuilder threadFactory(ThreadFactory threadFactory)
{
return this;
}
@Override
public ServiceProviderBuilder downInstancePolicy(DownInstancePolicy downInstancePolicy) {
return this;
}
@Override
public ServiceProviderBuilder additionalFilter(InstanceFilter tInstanceFilter) {
return this;
}
}
private static class NoopServiceProvider implements ServiceProvider
{
@Override
public void start() throws Exception
{
}
@Override
public ServiceInstance getInstance() throws Exception
{
return null;
}
@Override
public void noteError(ServiceInstance tServiceInstance) {
}
@Override
public void close() throws IOException
{
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy