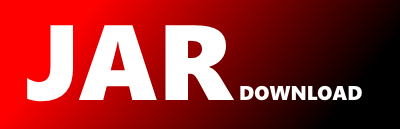
nablarch.common.handler.threadcontext.ThreadContextHandler Maven / Gradle / Ivy
package nablarch.common.handler.threadcontext;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import nablarch.core.ThreadContext;
import nablarch.fw.ExecutionContext;
import nablarch.fw.Handler;
import nablarch.fw.InboundHandleable;
import nablarch.fw.OutboundHandleable;
import nablarch.fw.Result;
/**
* スレッドコンテキストに保持される共通属性を管理するハンドラ。
*
* フレームワークには、スレッドコンテキストにユーザID・リクエストID・言語設定を保持する実装が含まれている。
* これらを有効化するには以下のリポジトリ設定を追加する。
* (同様にプロジェクト固有の属性を追加することも可能である。)
*
* <component class="nablarch.common.handler.threadcontext.ThreadContextHandler">
* <property name="attributes">
* <list>
* <!-- ユーザID -->
* <component class="nablarch.common.handler.threadcontext.UserIdAttribute">
* <property name="sessionKey" value="user.id" />
* <property name="anonymousId" value="guest" />
* </component>
*
* <!-- リクエストID -->
* <component class="nablarch.common.handler.threadcontext.RequestIdAttribute" />
*
* <!-- 言語 -->
* <component class="nablarch.common.handler.threadcontext.LanguageAttribute">
* <property name="defaultLanguage" value="ja" />
* </component>
* </list>
* </property>
* </component>
*
*/
public class ThreadContextHandler implements Handler
© 2015 - 2025 Weber Informatics LLC | Privacy Policy