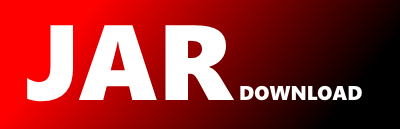
nablarch.test.core.util.ByteArrayAwareMap Maven / Gradle / Ivy
package nablarch.test.core.util;
import nablarch.core.util.BinaryUtil;
import nablarch.core.util.StringUtil;
import nablarch.core.util.map.MapWrapper;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import static nablarch.core.util.Builder.concat;
import static nablarch.core.util.Builder.join;
/**
* バイト配列を認識する{@link Map}実装クラス。
*
* @param キーの型
* @param 値の型
* @author T.Kawasaki
*/
public class ByteArrayAwareMap extends MapWrapper {
/** 元のマップ */
private Map orig;
/**
* コンストラクタ。
*
* @param orig 元のMap
*/
public ByteArrayAwareMap(Map orig) {
this.orig = orig;
}
/** {@inheritDoc} */
@Override
public Map getDelegateMap() {
return orig;
}
/**
* {@inheritDoc}
* 要素中にバイト配列が含まれていた場合、等価であれば等しい要素であるとみなす。
*/
@Override
public boolean equals(Object o) {
if (orig.equals(o)) {
return true;
}
if (!(o instanceof Map)) {
return false;
}
@SuppressWarnings("unchecked")
Map t = (Map) o;
if (t.size() != size()) {
return false;
}
for (Entry e : entrySet()) {
K key = e.getKey();
V value = e.getValue();
if (!(t.containsKey(key) && equals(value, t.get(key)))) {
return false;
}
}
return true;
}
/**
* 2つの値が等価であるか判定する。
*
* @param one 値1
* @param another 値2
* @return 判定結果
*/
private boolean equals(V one, V another) {
if (one == another) {
return true;
}
if (one instanceof byte[]) {
return another instanceof byte[] && Arrays.equals((byte[]) one, (byte[]) another);
}
return one.equals(another);
}
/**
* {@inheritDoc}
* 要素中にバイト配列が含まれていた場合、16進数文字列に変換して出力する。
*/
@Override
public String toString() {
List elements = new ArrayList(size());
for (Entry e : entrySet()) {
K key = e.getKey();
V value = e.getValue();
elements.add(concat(toString(key), "=", toString(value)));
}
return concat("{", join(elements, ", "), "}");
}
/**
* 文字列に変換する。
*
* @param orig 値
* @return 文字列
*/
private String toString(Object orig) {
if (orig == this) {
return "(this Map)";
}
if (orig instanceof byte[]) {
return BinaryUtil.convertToHexStringWithPrefix((byte[]) orig);
}
return orig == null ? "null" : StringUtil.toString(orig);
}
/** {@inheritDoc} */
@Override
public int hashCode() {
return orig.hashCode();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy