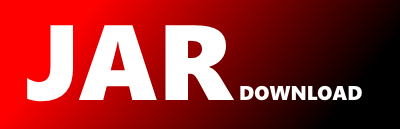
spock.genesis.generators.Generator.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spock-genesis Show documentation
Show all versions of spock-genesis Show documentation
Mostly lazy data generators for property based testing using the Spock test framework
The newest version!
package spock.genesis.generators
import groovy.transform.CompileStatic
import spock.genesis.extension.ExtensionMethods
import spock.genesis.generators.values.NullGenerator
/**
* An Iterator that generates a type (usually lazily)
* @param < E > the generated type
*/
@CompileStatic
abstract class Generator implements Iterable, Closeable {
protected final Random random = new Random()
/**
* Wraps this generator in a generator that returns values that matches the supplied predicate
* @param predicate
* @return a FilteredGenerator
*/
FilteredGenerator filter(Closure predicate) {
new FilteredGenerator(this, predicate)
}
@SuppressWarnings(['UnnecessaryPublicModifier']) // needed for generic parsing issue
public TransformingGenerator map(Closure transform) {
new TransformingGenerator(this, transform)
}
TransformingGenerator with(Closure> transform) {
Closure withClosure = { generatedValue ->
generatedValue.with(transform)
generatedValue
}
new TransformingGenerator(this, withClosure)
}
LimitedGenerator take(int qty) {
new LimitedGenerator(this, qty)
}
SequentialMultisourceGenerator then(Iterable... iterables) {
Generator[] all = new Generator[iterables.length + 1]
all[0] = this
for (int i = 0; i < iterables.length; i++) {
all[i + 1] = ExtensionMethods.toGenerator(iterables[i])
}
new SequentialMultisourceGenerator(all)
}
CyclicGenerator repeat() {
new CyclicGenerator(this)
}
LimitedGenerator multiply(int qty) {
take(qty)
}
CyclicGenerator getRepeat() {
repeat()
}
MultiSourceGenerator getWithNulls() {
withNulls(100)
}
/**Wraps this generator in a {@link spock.genesis.generators.MultiSourceGenerator} that randomly returns nulls
* @param resultsPerNull the average number of results from this generator per null result
* @return {@link spock.genesis.generators.MultiSourceGenerator}
*/
@SuppressWarnings('SpaceAroundMapEntryColon')
MultiSourceGenerator withNulls(int resultsPerNull) {
Map weightedGenerators = [(this): resultsPerNull, (new NullGenerator()): 1]
new MultiSourceGenerator(weightedGenerators)
}
MultiSourceGenerator and(Iterable iterable) {
if (MultiSourceGenerator.isAssignableFrom(this.getClass())) {
((MultiSourceGenerator) this) + iterable
} else {
new MultiSourceGenerator([this, iterable])
}
}
abstract UnmodifiableIterator iterator()
/**
* If false then the generator may still terminate when iterated
* @return true if the Generator will terminate
*/
boolean isFinite() {
!iterator().hasNext()
}
List getRealized() {
this.collect().asList()
}
@SuppressWarnings('EmptyMethodInAbstractClass')
void close() { }
/**
* Set the {@link Random} seed for this generator and all contained generators.
* This method mutates the generator!
* @param seed
* @return this generator
*/
Generator seed(Long seed) {
random.setSeed(seed)
this
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy