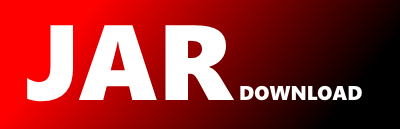
com.merkle.oss.magnolia.powernode.ValueConverter Maven / Gradle / Ivy
package com.merkle.oss.magnolia.powernode;
import org.apache.jackrabbit.value.ValueFactoryImpl;
import javax.annotation.Nullable;
import javax.inject.Provider;
import javax.jcr.*;
import java.math.BigDecimal;
import java.time.*;
import java.util.Calendar;
import java.util.Date;
import java.util.Optional;
public class ValueConverter {
private final ValueFactory factory;
private final Provider zoneIdProvider;
public ValueConverter(final ValueFactory factory, final Provider zoneIdProvider) {
this.factory = factory;
this.zoneIdProvider = zoneIdProvider;
}
public Optional toValue(@Nullable final BigDecimal value) {
return Optional.ofNullable(value).map(factory::createValue);
}
public Optional toValue(@Nullable final String value) {
return Optional.ofNullable(value).map(factory::createValue);
}
public Optional toValue(@Nullable final Double value) {
return Optional.ofNullable(value).map(factory::createValue);
}
public Optional toValue(@Nullable final Long value) {
return Optional.ofNullable(value).map(factory::createValue);
}
public Optional toValue(@Nullable final Integer value) {
return Optional.ofNullable(value).map(factory::createValue);
}
public Optional toValue(@Nullable final Boolean value) {
return Optional.ofNullable(value).map(factory::createValue);
}
public Optional toValue(@Nullable final Date value) {
return Optional.ofNullable(value)
.map(date -> {
final Calendar calendar = Calendar.getInstance();
calendar.setTime(value);
return calendar;
})
.map(factory::createValue);
}
public Optional toValue(@Nullable final Instant value) {
return Optional.ofNullable(value)
.map(date -> {
final Calendar calendar = Calendar.getInstance();
calendar.setTimeInMillis(value.toEpochMilli());
return calendar;
})
.map(factory::createValue);
}
public Optional toValue(@Nullable final LocalDate value) {
return Optional.ofNullable(value)
.map(localDate -> localDate.atStartOfDay(zoneIdProvider.get()))
.flatMap(this::toValue);
}
public Optional toValue(@Nullable final LocalDateTime value) {
return Optional.ofNullable(value)
.map(localDateTime -> localDateTime.atZone(zoneIdProvider.get()))
.flatMap(this::toValue);
}
public Optional toValue(@Nullable final ZonedDateTime value) {
return Optional.ofNullable(value)
.map(ZonedDateTime::toInstant)
.flatMap(this::toValue);
}
public Optional toValue(@Nullable final Binary value) {
return Optional.ofNullable(value).map(factory::createValue);
}
public Optional getDecimal(final Value value) throws RepositoryException {
return getPropertyOptional(value::getDecimal);
}
public Optional getString(final Value value) throws RepositoryException {
return getPropertyOptional(value::getString);
}
public Optional getDouble(final Value value) throws RepositoryException {
return getPropertyOptional(value::getDouble);
}
public Optional getLong(final Value value) throws RepositoryException {
return getPropertyOptional(value::getLong);
}
public Optional getInteger(final Value value) throws RepositoryException {
return getLong(value).map(Long::intValue);
}
public Optional getBoolean(final Value value) throws RepositoryException {
return getPropertyOptional(value::getBoolean);
}
public Optional getDate(final Value value) throws RepositoryException {
return getPropertyOptional(() -> value.getDate().getTime());
}
public Optional getInstant(final Value value) throws RepositoryException {
return getPropertyOptional(() ->
Instant.ofEpochMilli(value.getDate().getTimeInMillis())
);
}
public Optional getLocalDate(final Value value) throws RepositoryException {
return getInstant(value).map(instant -> LocalDate.ofInstant(instant, zoneIdProvider.get()));
}
public Optional getLocalDateTime(final Value value) throws RepositoryException {
return getInstant(value).map(instant -> LocalDateTime.ofInstant(instant, zoneIdProvider.get()));
}
public Optional getZonedDateTime(final Value value) throws RepositoryException {
return getInstant(value).map(instant -> ZonedDateTime.ofInstant(instant, zoneIdProvider.get()));
}
public Optional getBinary(final Value value) throws RepositoryException {
return getPropertyOptional(() ->
ValueFactoryImpl.getInstance().createBinary(value.getBinary().getStream())
);
}
private Optional getPropertyOptional(final PathNotFoundProvider provider) throws RepositoryException {
try {
return Optional.ofNullable(provider.get());
} catch (ValueFormatException e) {
return Optional.empty();
}
}
interface PathNotFoundProvider {
T get() throws RepositoryException;
}
public interface Factory {
ValueConverter create(final ValueFactory valueFactory);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy