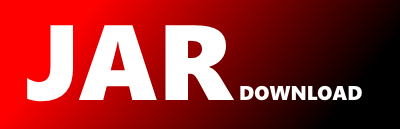
com.nathanaelrota.cmmi.maven.requirements.RequirementClassHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of requirements-plugin Show documentation
Show all versions of requirements-plugin Show documentation
Maven Requirements Traceability Plugin
The newest version!
package com.nathanaelrota.cmmi.maven.requirements;
/*
* Copyright 2001-2005 The Apache Software Foundation.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import java.lang.annotation.Annotation;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* @author Nathanael ROTA
*/
public class RequirementClassHandler extends RequirementClassLoader {
/**
* Constant Definition for CLASSNAME_METHOD_SEPARATOR
*/
private final String CLASSNAME_METHOD_SEPARATOR = "::";
/**
* ctor
*/
public RequirementClassHandler(ClassLoader parent) {
super(parent);
}
/**
* check annontation of methods
* @param className the class
* @return list of annotation by method
*/
public Map> check(String className) {
Map> values = new HashMap>();
try {
for (Method method : this.loadClass(className, true).getDeclaredMethods()) {
for (Annotation annotation : method.getDeclaredAnnotations()) {
String methodName = className + CLASSNAME_METHOD_SEPARATOR + method.getName();
this.checkAnnotation(annotation, methodName, values);
}
}
} catch (NoClassDefFoundError noClassDefFoundError) {
error("NoClassDefFoundError during the check of \"" + className + "\"");
} catch (ClassNotFoundException e) {
error("ClassNotFoundException during the check of \"" + className + "\"");
}
return values;
}
/**
* Check if the annotation contain Requirement
*
* @param annotation
* the annotation
* @param methodName
* the Method NAme
*/
private void checkAnnotation(Annotation annotation, String methodName, Map> values) {
if (annotation instanceof Requirement) {
Requirement requirements = (Requirement) annotation;
for (String requirement : requirements.value()) {
this.addMethod(methodName, requirement, values);
}
}
}
/**
* Add Method to Requirement
*
* @param methodName
* the Method Name
* @param requirement
* the Requirement
*/
public void addMethod(String methodName, String requirement, Map> values) {
if (!values.containsKey(requirement)) {
values.put(requirement, new ArrayList());
}
values.get(requirement).add(methodName);
debug("" + methodName + " defines " + requirement);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy