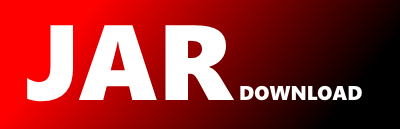
com.nativelibs4java.opencl.util.LinearAlgebraUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of javacl-jna Show documentation
Show all versions of javacl-jna Show documentation
JavaCL is an Object-Oriented API that makes the C OpenCL API available to Java in a very natural way.
It hides away the complexity of cross-platform C bindings, has a clean OO design (with generics, Java enums, NIO buffers, fully typed exceptions...), provides high-level features (OpenGL-interop, array reductions) and comes with samples and demos.
For more info, please visit http://code.google.com/p/nativelibs4java/wiki/OpenCL.
The newest version!
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package com.nativelibs4java.opencl.util;
import com.nativelibs4java.opencl.CLBuffer;
import com.nativelibs4java.opencl.CLBuildException;
import com.nativelibs4java.opencl.CLContext;
import com.nativelibs4java.opencl.CLDoubleBuffer;
import com.nativelibs4java.opencl.CLEvent;
import com.nativelibs4java.opencl.CLKernel;
import com.nativelibs4java.opencl.CLPlatform.DeviceFeature;
import com.nativelibs4java.opencl.CLProgram;
import com.nativelibs4java.opencl.CLQueue;
import com.nativelibs4java.opencl.JavaCL;
import com.nativelibs4java.opencl.util.ReductionUtils;
import com.nativelibs4java.opencl.util.ReductionUtils.Reductor;
import com.nativelibs4java.util.IOUtils;
import com.ochafik.util.listenable.Pair;
import static com.nativelibs4java.util.NIOUtils.*;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.InputStream;
import java.io.PrintWriter;
import java.io.StringWriter;
import java.nio.Buffer;
import java.nio.DoubleBuffer;
import java.util.EnumMap;
import java.util.HashMap;
import java.util.Map;
import java.util.logging.Level;
import java.util.logging.Logger;
/**
*
* @author ochafik
*/
@SuppressWarnings("unused")
public class LinearAlgebraUtils {
final LinearAlgebraKernels kernels;
final CLQueue queue;
public LinearAlgebraUtils(boolean doubleCapable) throws IOException, CLBuildException {
this(JavaCL.createBestContext(doubleCapable ? DeviceFeature.DoubleSupport : null).createDefaultQueue());
}
public LinearAlgebraUtils(CLQueue queue) throws IOException, CLBuildException {
this.queue = queue;
kernels = new LinearAlgebraKernels(queue.getContext());
}
public CLContext getContext() {
return getQueue().getContext();
}
public CLQueue getQueue() {
return queue;
}
public synchronized CLEvent multiply(
CLBuffer a, int aRows, int aColumns,
CLBuffer b, int bRows, int bColumns,
CLBuffer out, //long outRows, long outColumns,
CLEvent... eventsToWaitFor) throws CLBuildException
{
if (a.getBufferClass() == DoubleBuffer.class)
return multiplyDoubles((CLBuffer)a, aRows, aColumns, (CLBuffer)b, bRows, bColumns, (CLBuffer)out, eventsToWaitFor);
throw new UnsupportedOperationException();
}
public synchronized CLEvent multiplyDoubles(
CLBuffer a, int aRows, int aColumns,
CLBuffer b, int bRows, int bColumns,
CLBuffer out, //long outRows, long outColumns,
CLEvent... eventsToWaitFor) throws CLBuildException
{
if (a == null || b == null || out == null)
throw new IllegalArgumentException("Null matrix");
if (aColumns != bRows || out.getElementCount() != (aRows * bColumns))
throw new IllegalArgumentException("Invalid matrix sizes : multiplying matrices of sizes (A, B) and (B, C) requires output of size (A, C)");
long outRows = aRows;
long outColumns = bColumns;
return kernels.mulMat(queue,
a, (int)aColumns,
b, (int)bColumns,
out,
new int[] { (int)outRows, (int)outColumns },
null,
eventsToWaitFor
);
}
/*synchronized CLEvent dot(CLVector a b out, CLEvent... eventsToWaitFor) {
CLEvent.waitFor(eventsToWaitFor);
a.waitForRead();
b.waitForRead();
out.waitForWrite();
FV aa = newVector(fallBackLibrary, a);
FV bb = newVector(fallBackLibrary, b);
out.write(aa.dot(bb, null).read());
return null;
}*/
Reductor addReductor;
synchronized Reductor getAddReductor() {
if (addReductor == null) {
try {
addReductor = ReductionUtils.createReductor(getContext(), ReductionUtils.Operation.Add, OpenCLType.Double, 1);
} catch (CLBuildException ex) {
Logger.getLogger(LinearAlgebraUtils.class.getName()).log(Level.SEVERE, null, ex);
throw new RuntimeException("Failed to create an addition reductor !", ex);
}
}
return addReductor;
}
public synchronized CLEvent transpose(CLBuffer a, int aRows, int aColumns, CLBuffer out, CLEvent... eventsToWaitFor) throws CLBuildException {
if (a.getBufferClass() == DoubleBuffer.class)
return transposeDoubles((CLBuffer)a, aRows, aColumns, (CLBuffer)out, eventsToWaitFor);
throw new UnsupportedOperationException();
}
public synchronized CLEvent transposeDoubles(CLBuffer a, int aRows, int aColumns, CLBuffer out, CLEvent... eventsToWaitFor) throws CLBuildException {
return kernels.transpose(queue,
a, aRows, aColumns,
out,
new int[] { (int)aColumns, (int)aRows },
null,
eventsToWaitFor
);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy