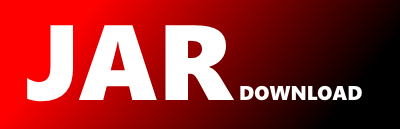
com.nativelibs4java.opencl.util.Transformer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of javacl-jna Show documentation
Show all versions of javacl-jna Show documentation
JavaCL is an Object-Oriented API that makes the C OpenCL API available to Java in a very natural way.
It hides away the complexity of cross-platform C bindings, has a clean OO design (with generics, Java enums, NIO buffers, fully typed exceptions...), provides high-level features (OpenGL-interop, array reductions) and comes with samples and demos.
For more info, please visit http://code.google.com/p/nativelibs4java/wiki/OpenCL.
The newest version!
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package com.nativelibs4java.opencl.util;
import com.nativelibs4java.opencl.CLBuffer;
import com.nativelibs4java.opencl.CLBuildException;
import com.nativelibs4java.opencl.CLContext;
import com.nativelibs4java.opencl.CLEvent;
import com.nativelibs4java.opencl.CLException;
import com.nativelibs4java.opencl.CLMem;
import com.nativelibs4java.opencl.CLQueue;
import com.nativelibs4java.util.NIOUtils;
import java.nio.Buffer;
import java.nio.DoubleBuffer;
import java.nio.FloatBuffer;
/**
* Generic homogen transformer class
* @author ochafik
* @param NIO buffer class that represents the data consumed and produced by this transformer
* @param primitive array class that represents the data consumed and produced by this transformer
*/
public interface Transformer {
CLContext getContext();
A transform(CLQueue queue, A input, boolean inverse);
B transform(CLQueue queue, B input, boolean inverse);
CLEvent transform(CLQueue queue, CLBuffer input, CLBuffer output, boolean inverse, CLEvent... eventsToWaitFor) throws CLException;
int computeOutputSize(int inputSize);
public abstract class AbstractTransformer implements Transformer {
protected final Class primitiveClass;
protected final CLContext context;
public AbstractTransformer(CLContext context, Class primitiveClass) {
this.primitiveClass = primitiveClass;
this.context = context;
}
public CLContext getContext() { return context; }
public int computeOutputSize(int inputSize) {
return inputSize;
}
public A transform(CLQueue queue, A input, boolean inverse) {
return (A)NIOUtils.getArray(transform(queue, (B)NIOUtils.wrapArray(input), inverse));
}
public B transform(CLQueue queue, B in, boolean inverse) {
int inputSize = in.capacity();
int length = inputSize / 2;
CLBuffer inBuf = context.createBuffer(CLMem.Usage.Input, in, true); // true = copy
CLBuffer outBuf = context.createBuffer(CLMem.Usage.Output, primitiveClass, computeOutputSize(inputSize));
CLEvent dftEvt = transform(queue, inBuf, outBuf, inverse);
inBuf.release();
B out = (B)outBuf.read(queue, dftEvt);
outBuf.release();
return out;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy