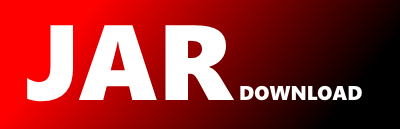
com.nativelibs4java.opencl.util.fft.FloatFFTPow2 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of javacl Show documentation
Show all versions of javacl Show documentation
JavaCL is an Object-Oriented API that makes the C OpenCL API available to Java in a very natural way.
It hides away the complexity of cross-platform C bindings, has a clean OO design (with generics, Java enums, NIO buffers, fully typed exceptions...), provides high-level features (OpenGL-interop, array reductions) and comes with samples and demos.
For more info, please visit http://code.google.com/p/nativelibs4java/wiki/OpenCL.
package com.nativelibs4java.opencl.util.fft;
import com.nativelibs4java.opencl.*;
import java.io.IOException;
import java.nio.FloatBuffer;
/**
* OpenCL Fast Fourier Transform for array sizes that are powers of two (simple precision floating point numbers)
*/
public class FloatFFTPow2 extends AbstractFFTPow2 {
final FloatFFTProgram program;
public FloatFFTPow2(CLContext context) throws IOException {
super(context, Float.class);
this.program = new FloatFFTProgram(context);
program.getProgram().setFastRelaxedMath();
}
public FloatFFTPow2() throws IOException {
this(JavaCL.createBestContext());
}
protected CLEvent cooleyTukeyFFTTwiddleFactors(CLQueue queue, int N, CLBuffer buf, CLEvent... evts) throws CLException {
return program.cooleyTukeyFFTTwiddleFactors(queue, N, buf, new int[] { N / 2 }, null, evts);
}
protected CLEvent cooleyTukeyFFTCopy(CLQueue queue, CLBuffer inBuf, CLBuffer outBuf, int length, CLBuffer offsetsBuf, boolean inverse, CLEvent... evts) throws CLException {
return program.cooleyTukeyFFTCopy(queue, inBuf, outBuf, length, offsetsBuf, inverse ? 1.0f / length : 1, new int[] { length }, null, evts);
}
protected CLEvent cooleyTukeyFFT(CLQueue queue, CLBuffer Y, int N, CLBuffer twiddleFactors, int inverse, int[] dims, CLEvent... evts) throws CLException {
return program.cooleyTukeyFFT(queue, Y, N, twiddleFactors, inverse, dims, null, evts);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy