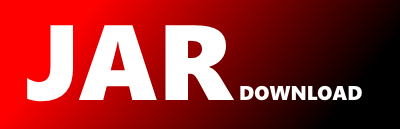
com.ochafik.lang.jnaerator.MatchingUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jnaerator Show documentation
Show all versions of jnaerator Show documentation
JNAerator (pronounce "generator") simply parses C and Objective-C headers and generates the corresponding JNA and Rococoa Java interfaces (it also has a very limited support for C++).
This lets Java programmers access native libraries transparently, with full IDE support and little to no hand-tweaking.
Users who are looking for ready-to-use libraries should check the NativeLibs4Java project instead.
package com.ochafik.lang.jnaerator;
import java.util.*;
//import org.bridj.structs.StructIO;
//import org.bridj.structs.Array;
import com.ochafik.lang.jnaerator.parser.*;
import com.ochafik.lang.jnaerator.parser.StoredDeclarations.*;
import com.ochafik.lang.jnaerator.parser.TypeRef.*;
import com.ochafik.lang.jnaerator.parser.Expression.*;
import com.ochafik.lang.jnaerator.parser.Declarator.*;
import com.ochafik.util.listenable.Pair;
import static com.ochafik.lang.jnaerator.parser.ElementsHelper.*;
public class MatchingUtils {
public static TypeRef recognizeSizeOf(Expression e) {
if (!(e instanceof FunctionCall))
return null;
FunctionCall fc = (FunctionCall)e;
List> args = fc.getArguments();
if (args.size() != 1)
return null;
Pair arg = args.get(0);
if (arg == null)
return null;
Expression f = fc.getFunction();
if (!(f instanceof VariableRef))
return null;
VariableRef vr = (VariableRef)f;
if (!"sizeof".equals(String.valueOf(vr.getName())))
return null;
Expression a = arg.getSecond();
if (!(a instanceof Expression.TypeRefExpression))
return null;
Expression.TypeRefExpression tr = (Expression.TypeRefExpression)a;
return tr.getType();
}
public static Pair recognizeSizeOfMult(Expression e) {
List mult = recognizeMultiply(e);
if (mult == null)
return null;
TypeRef typeRef = null;
Expression f = null;
for (Iterator it = mult.iterator(); it.hasNext();) {
Expression x = it.next();
TypeRef tr = recognizeSizeOf(x);
if (tr != null && typeRef == null) { // only take first sizeof
typeRef = tr;
} else {
if (f == null)
f = x;
else
f = expr(f, BinaryOperator.Multiply, x);
}
}
if (typeRef == null)
return null;
if (f == null)
f = expr(1);
return new Pair(typeRef, f);
}
public static List recognizeMultiply(Expression e) {
List ret = new ArrayList();
if (!recognizeMultiply(e, ret))
return null;
return ret;
}
public static boolean recognizeMultiply(Expression e, List out) {
if (!(e instanceof BinaryOp))
return false;
BinaryOp op = (BinaryOp)e;
if (op.getOperator() != BinaryOperator.Multiply)
return false;
for (Expression sub : new Expression[] { op.getFirstOperand(), op.getSecondOperand() })
if (!recognizeMultiply(sub, out))
out.add(sub);
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy