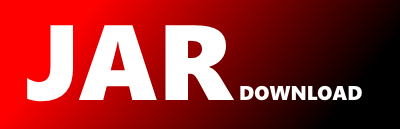
com.ochafik.xml.XMLUtils Maven / Gradle / Ivy
/*
Copyright (c) 2009-2011 Olivier Chafik, All Rights Reserved
This file is part of JNAerator (http://jnaerator.googlecode.com/).
JNAerator is free software: you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
JNAerator is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with JNAerator. If not, see .
*/
package com.ochafik.xml;
import java.io.File;
import java.io.StringWriter;
import java.text.MessageFormat;
import java.util.ArrayList;
import java.util.Collection;
import java.util.LinkedList;
import java.util.List;
import java.util.regex.Pattern;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.transform.Transformer;
import javax.xml.transform.TransformerFactory;
import javax.xml.transform.dom.DOMSource;
import javax.xml.transform.stream.StreamResult;
import org.w3c.dom.Document;
import org.w3c.dom.NamedNodeMap;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import com.ochafik.util.listenable.Adapter;
import com.ochafik.util.string.RegexUtils;
//import ochafik.babel.*;
public class XMLUtils {
public static String getAttribute(Node node, String name) {
NamedNodeMap m=node.getAttributes();
if (m==null) return null;
Node att=m.getNamedItem(name);
if (att==null) return null;
return att.getNodeValue();
}
public static Node getFirstNamedNode(Node node, String name) {
if (node.getNodeName().equalsIgnoreCase(name)) return node;
else {
NodeList list=node.getChildNodes();
for (int i=0,len=list.getLength();i getByName(Node node, String name) {
List result = new LinkedList();
getByName(node, name, result);
return result;
}
private static void getByName(Node node, String name, List result) {
if (node.getNodeName().equalsIgnoreCase(name)) {
result.add(node);
} else {
NodeList list=node.getChildNodes();
for (int i=0,len=list.getLength();i getChildrenByName(Node node, String name) {
Collection nodes=new ArrayList();
getChildrenByName(node,name,nodes);
return nodes;
}
private static void getChildrenByName(Node node, String name, Collection nodes) {
NodeList list=node.getChildNodes();
for (int i=0,len=list.getLength();i getChildElements(Node node) {
NodeList children = node.getChildNodes();
int childCount = children.getLength();
List nodes = new ArrayList(childCount);
for (int i = 0; i < childCount; i++) {
Node child = children.item(i);
if (child.getNodeType() == Node.ELEMENT_NODE) {
nodes.add(child);
}
}
return nodes;
}
public static List getAttributes(Node node) {
NamedNodeMap attrs = node.getAttributes();
int attrCount = attrs.getLength();
List nodes = new ArrayList(attrCount);
for (int i = 0; i < attrCount; i++) {
nodes.add(attrs.item(i));
}
return nodes;
}
public static StringBuffer gatherTextPCDATAAndCDATADescendants(Node node,StringBuffer sb,String separator) {
if (sb==null) sb=new StringBuffer();
int t=node.getNodeType();
if (t==Node.CDATA_SECTION_NODE || t==Node.TEXT_NODE) {
//sb.append("================= "+node.getNodeName()+" =================");
sb.append(node.getNodeValue());
} else {
NodeList list = node.getChildNodes();
for (int i=0,len=list.getLength();i", ">");
}
private static final Pattern xmlTagPattern=Pattern.compile("|?\\w+[^\">]*(?:(?:\"[^\"]*\")[\">]*)*>");
private static final Pattern spacesPattern=Pattern.compile("\\s+");
private static final MessageFormat spaceMessageFormat=new MessageFormat(" ");
public static String stripTags(String xmlString) {
String strippedHtml = RegexUtils.regexReplace(xmlTagPattern, xmlString, (Adapter)null);
return RegexUtils.regexReplace(spacesPattern,strippedHtml,spaceMessageFormat).trim();
}
public static Document readXML(File file) throws Exception {
DocumentBuilderFactory documentBuilderFactory = DocumentBuilderFactory.newInstance();
documentBuilderFactory.setNamespaceAware(true); // never forget this!
DocumentBuilder documentBuilder = documentBuilderFactory.newDocumentBuilder();
return documentBuilder.parse(file);
}
public static List list(NodeList nodeList) {
if (nodeList == null)
return null;
int len = nodeList.getLength();
List list = new ArrayList(len);
for (int i = 0; i < len; i++)
list.add(nodeList.item(i));
return list;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy