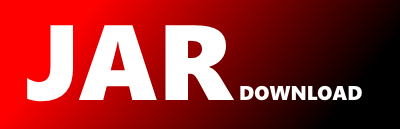
com.natpryce.krouton.composition_operators.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of krouton Show documentation
Show all versions of krouton Show documentation
Type-safe and compositional URL routing and reverse routing
package com.natpryce.krouton
operator fun String.unaryPlus() = root + this
operator fun PathTemplate.unaryPlus() = root + this
operator fun PathTemplate.plus(fixedElement: String): PathTemplate =
this + LiteralPathElement(fixedElement)
@JvmName("plusPrefix")
operator fun PathTemplate.plus(rest: PathTemplate): PathTemplate =
PrefixedPathTemplate(this, rest)
@JvmName("plusSuffix")
operator fun PathTemplate.plus(suffix: PathTemplate): PathTemplate =
SuffixedPathTemplate(this, suffix)
infix fun PathTemplate.where(p: (T) -> Boolean): PathTemplate = RestrictedPathTemplate(this, p)
infix fun PathTemplate.asA(projection: Projection): PathTemplate =
ProjectedPathTemplate(this, projection)
private fun appender(tPath: PathTemplate, uPath: PathTemplate, fromParts: (Tuple2) -> V, toParts: (V) -> Tuple2) =
AppendedPathTemplate(tPath, uPath) asA object : Projection, V> {
override fun fromParts(parts: Tuple2): V = fromParts(parts)
override fun toParts(mapped: V): Tuple2 = toParts(mapped)
}
@JvmName("appendScalarScalar")
operator fun PathTemplate.plus(rest: PathTemplate): PathTemplate> =
AppendedPathTemplate(this, rest)
@JvmName("appendScalarTuple2")
operator fun PathElement.plus(rest: PathTemplate>) =
appender(this, rest,
fromParts = { tupleFlat(it.val1, it.val2) },
toParts = { tuple(it.val1, tuple(it.val2, it.val3)) })
@JvmName("appendScalarTuple3")
operator fun PathElement.plus(rest: PathTemplate>) =
appender(this, rest,
fromParts = { tupleFlat(it.val1, it.val2) },
toParts = { tuple(it.val1, tuple(it.val2, it.val3, it.val4)) })
@JvmName("appendScalarTuple4")
operator fun PathElement.plus(rest: PathTemplate>) =
appender(this, rest,
fromParts = { tupleFlat(it.val1, it.val2) },
toParts = { tuple(it.val1, tuple(it.val2, it.val3, it.val4, it.val5)) })
@JvmName("appendTuple2Scalar")
operator fun PathTemplate>.plus(rest: PathTemplate) =
appender(this, rest, { tupleFlat(it.val1, it.val2) }, { tuple(tuple(it.val1, it.val2), it.val3) })
@JvmName("appendTuple2Tuple2")
operator fun PathTemplate>.plus(rest: PathTemplate>) =
appender(this, rest, { tupleFlat(it.val1, it.val2) }, { tuple(tuple(it.val1, it.val2), tuple(it.val3, it.val4)) })
@JvmName("appendTuple2Tuple3")
operator fun PathTemplate>.plus(rest: PathTemplate>) =
appender(this, rest, { tupleFlat(it.val1, it.val2) }, { tuple(tuple(it.val1, it.val2), tuple(it.val3, it.val4, it.val5)) })
@JvmName("appendTuple3Scalar")
operator fun PathTemplate>.plus(rest: PathTemplate) =
appender(this, rest, { tupleFlat(it.val1, it.val2) }, { tuple(tuple(it.val1, it.val2, it.val3), it.val4) })
@JvmName("appendTuple3Tuple2")
operator fun PathTemplate>.plus(rest: PathTemplate>) =
appender(this, rest, { tupleFlat(it.val1, it.val2) }, { tuple(tuple(it.val1, it.val2, it.val3), tuple(it.val4, it.val5)) })
@JvmName("appendTuple4Scalar")
operator fun PathTemplate>.plus(rest: PathTemplate) =
appender(this, rest, { tupleFlat(it.val1, it.val2) }, { tuple(tuple(it.val1, it.val2, it.val3, it.val4), it.val5) })
© 2015 - 2025 Weber Informatics LLC | Privacy Policy