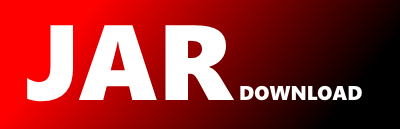
com.natpryce.makeiteasy.MakeItEasy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of make-it-easy Show documentation
Show all versions of make-it-easy Show documentation
A tiny framework that makes it easy to write Test Data Builders in Java
package com.natpryce.makeiteasy;
import com.natpryce.makeiteasy.sequence.ElementsSequence;
import java.util.*;
import static java.util.Arrays.asList;
/**
* Syntactic sugar for using Make It Easy test-data builders.
*/
@SuppressWarnings("unused")
public class MakeItEasy {
public static Maker a(Instantiator instantiator, PropertyValue super T, ?> ... propertyProviders) {
return new Maker<>(instantiator, propertyProviders);
}
public static Maker an(Instantiator instantiator, PropertyValue super T, ?> ... propertyProviders) {
return new Maker<>(instantiator, propertyProviders);
}
public static PropertyValue with(Property property, W value) {
return new PropertyValue<>(property, new SameValueDonor(value));
}
public static PropertyValue with(W value, Property property) {
return new PropertyValue<>(property, new SameValueDonor(value));
}
public static PropertyValue with(Property property, Donor valueDonor) {
return new PropertyValue<>(property, valueDonor);
}
public static PropertyValue with(Donor valueDonor, Property property) {
return new PropertyValue<>(property, valueDonor);
}
public static PropertyValue withNull(Property property) {
return new PropertyValue<>(property, new SameValueDonor(null));
}
public static Donor theSame(Instantiator instantiator, PropertyValue super T, ?> ... propertyProviders) {
return theSame(an(instantiator, propertyProviders));
}
public static Donor theSame(Donor originalDonor) {
return new SameValueDonor<>(originalDonor.value());
}
public static T make(Maker maker) {
return maker.value();
}
@SafeVarargs
public static Donor> listOf(Donor extends T>... donors) {
return new NewCollectionDonor, T>(donors) {
protected List newCollection() { return new ArrayList<>(); }
};
}
@SafeVarargs
public static Donor> setOf(Donor extends T>... donors) {
return new NewCollectionDonor, T>(donors) {
protected Set newCollection() { return new HashSet<>(); }
};
}
@SafeVarargs
public static > Donor> sortedSetOf(Donor extends T>... donors) {
return new NewCollectionDonor, T>(donors) {
protected SortedSet newCollection() { return new TreeSet<>(); }
};
}
public static Donor from(final Iterable values) {
return new ElementsSequence<>(values, Collections.emptyList());
}
@SafeVarargs
public static Donor from(T ... values) {
return from(asList(values));
}
public static Donor fromRepeating(Iterable values) {
return new ElementsSequence<>(values, values);
}
@SafeVarargs
public static Donor fromRepeating(T ... values) {
return fromRepeating(asList(values));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy